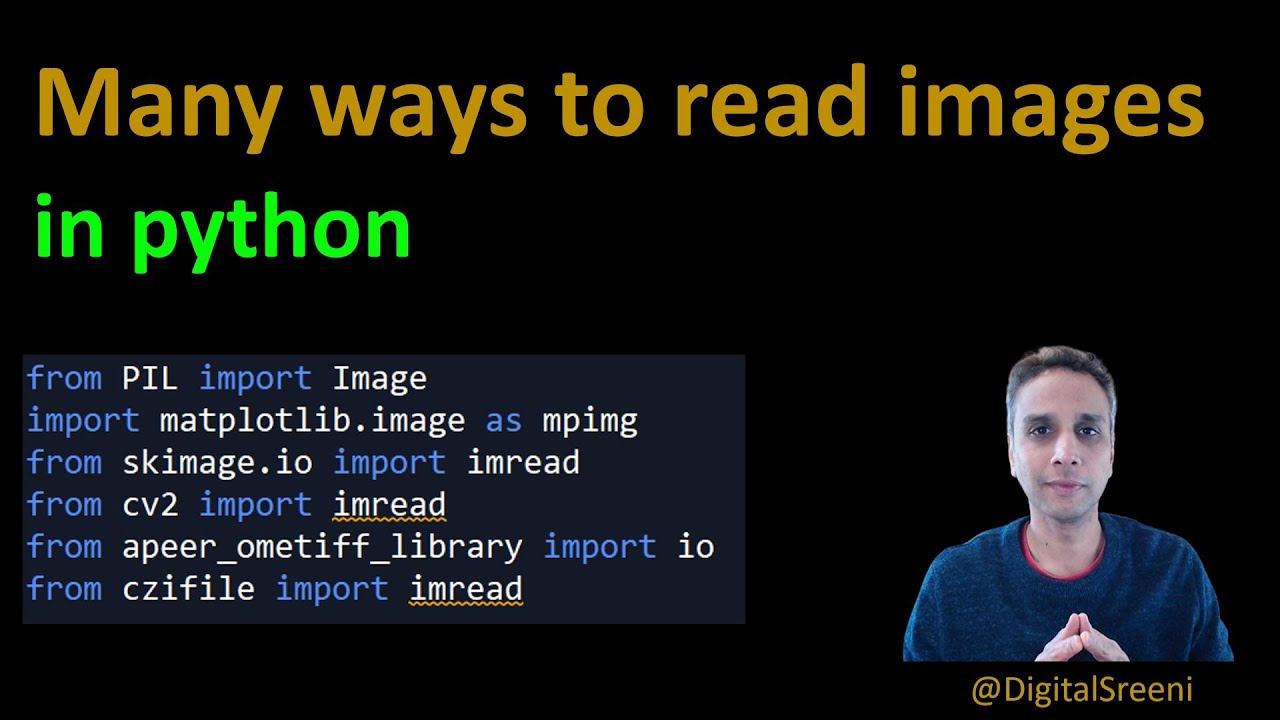
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Image Processing with Python
Image processing is an essential skill for students, researchers, and enthusiasts looking to analyze and manipulate images programmatically. Python, with its rich ecosystem of libraries, provides a powerful toolkit for image processing tasks. In this comprehensive guide, we'll explore various Python libraries that enable you to read, manipulate, and process images effectively.
Choosing the Right Library
Python offers several libraries for image processing, each with its unique strengths. Understanding these differences is crucial to selecting the right tool for your specific needs.
Pillow (PIL)
- Primary Purpose: Basic image handling and processing tasks
-
Installation:
pip install Pillow
(import asPIL
) - Strengths: Offers functionalities like cropping, resizing, and basic filtering
Matplotlib
- Primary Purpose: Primarily a plotting library, but can also handle image visualization
-
Installation:
pip install matplotlib
Scikit-Image
- Primary Purpose: Advanced image processing tasks
- Strengths: Segmentation, geometric transformations, color space manipulation
OpenCV
- Primary Purpose: Computer vision tasks and advanced image processing
- Strengths: Facial detection, object detection, motion tracking, and more
-
Installation:
pip install opencv-python
Reading Images in Python
Reading images into Python is the first step in the image processing workflow. Here's how to do it with each library:
Using Pillow
from PIL import Image
img = Image.open('path/to/image.jpg')
Remember, images read with Pillow are not in NumPy array format by default, which might be necessary for mathematical operations.
Using Matplotlib
import matplotlib.image as mpimg
img = mpimg.imread('path/to/image.jpg')
Matplotlib imports images as NumPy arrays by default, making it convenient for further processing.
Using Scikit-Image
from skimage import io
img = io.imread('path/to/image.jpg')
Like Matplotlib, Scikit-Image also loads images as NumPy arrays.
Using OpenCV
import cv2
img = cv2.imread('path/to/image.jpg', cv2.IMREAD_COLOR)
OpenCV reads images in BGR format, which might require conversion to RGB for certain applications.
Handling Proprietary and Special Image Formats
Proprietary formats like CZI (Zeiss microscope images) or OME-TIFF (Open Microscopy Environment TIFF) require specialized libraries or modules for reading. Libraries such as czifile
and apeer-ometiff-library
can be used for these purposes. Always ensure to install the necessary packages and understand the format's specificities.
Automating Image Processing Tasks
Processing multiple images or navigating through directories of images can be automated using the glob
library. This allows for batch processing of images, significantly improving efficiency.
import cv2
import glob
for file in glob.glob('path/to/images/*.jpg'):
img = cv2.imread(file)
# Process each image as needed
Conclusion
Python's diverse libraries offer robust tools for a variety of image processing tasks. Whether you're resizing a batch of photos with Pillow, analyzing scientific images with Scikit-Image, or building a computer vision model with OpenCV, Python has a library to meet your needs. Start exploring these libraries and unlock the full potential of image processing in Python.
For more detailed examples and tutorials, check out the original video here.