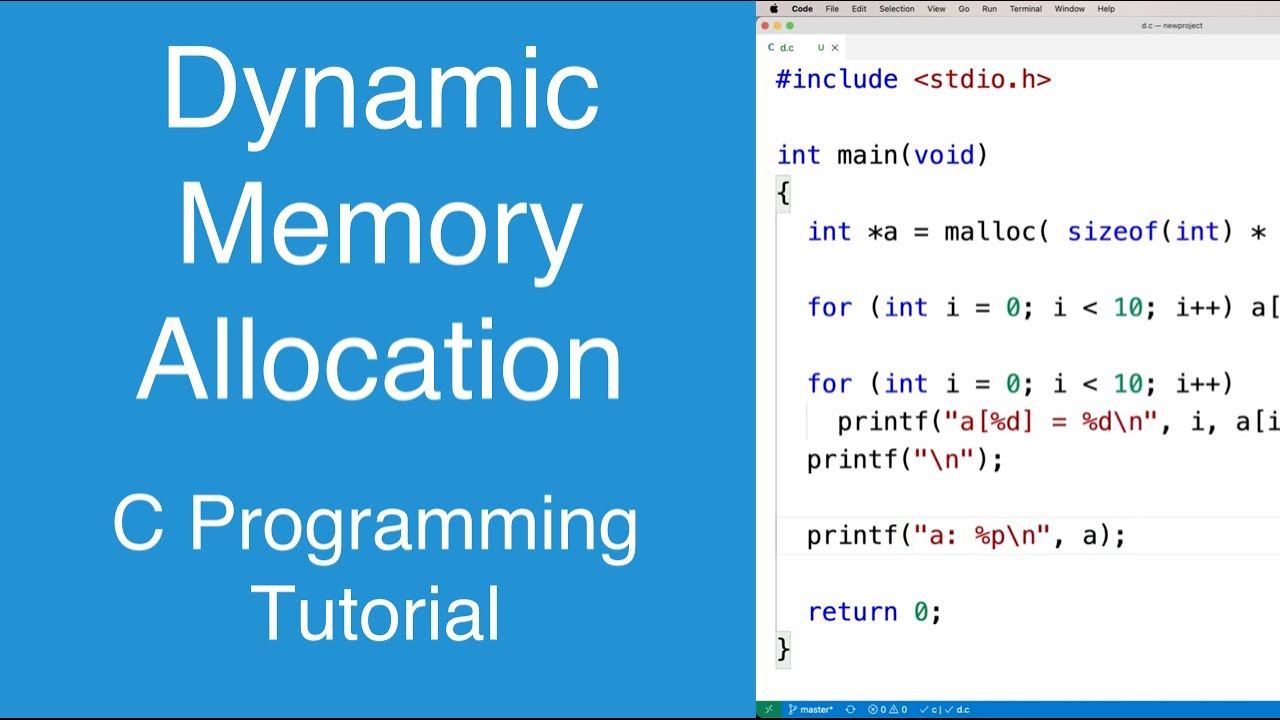
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Dynamic Memory Allocation in C
Dynamic memory allocation is a crucial concept in C programming that allows for efficient use of memory resources. Unlike static memory allocation, where the size of variables is determined at compile-time, dynamic memory allocation enables programs to request and release memory as needed during runtime. This flexibility is particularly useful when dealing with data structures of varying sizes or when the memory requirements of a program are not known in advance.
Understanding Memory in C: Stack vs Heap
Before diving into dynamic memory allocation, it's essential to understand how memory works in C. In C programs, memory is primarily organized into two main regions: the stack and the heap.
The Stack
The stack is a region of memory that stores local variables, function parameters, and return addresses. It operates in a Last-In-First-Out (LIFO) manner, meaning that the most recently added item is the first to be removed. Key characteristics of the stack include:
- Automatic allocation and deallocation of memory
- Fixed size for variables
- Fast access times
- Limited in size
The Heap
The heap is a region of memory used for dynamic memory allocation. Unlike the stack, memory in the heap is allocated and deallocated manually by the programmer. Key characteristics of the heap include:
- Manual memory management
- Variable size allocation
- Slower access times compared to the stack
- Larger available memory space
Dynamic Memory Allocation Functions
C provides several functions for dynamic memory allocation, each with its own purpose and characteristics. The most commonly used functions are:
malloc()
The malloc()
function is used to allocate a block of memory on the heap. It takes the number of bytes to allocate as an argument and returns a pointer to the allocated memory.
#include <stdlib.h>
int *ptr = (int *)malloc(10 * sizeof(int));
In this example, we allocate space for 10 integers on the heap.
calloc()
The calloc()
function is similar to malloc()
, but it initializes the allocated memory to zero. It takes two arguments: the number of elements to allocate and the size of each element.
#include <stdlib.h>
int *ptr = (int *)calloc(10, sizeof(int));
This allocates space for 10 integers and initializes them to zero.
realloc()
The realloc()
function is used to resize a previously allocated block of memory. It takes two arguments: a pointer to the previously allocated memory and the new size in bytes.
#include <stdlib.h>
int *ptr = (int *)malloc(10 * sizeof(int));
ptr = (int *)realloc(ptr, 15 * sizeof(int));
This example resizes the previously allocated memory to accommodate 15 integers instead of 10.
free()
The free()
function is used to deallocate memory that was previously allocated using malloc()
, calloc()
, or realloc()
.
#include <stdlib.h>
int *ptr = (int *)malloc(10 * sizeof(int));
// Use the allocated memory
free(ptr);
It's crucial to call free()
when the dynamically allocated memory is no longer needed to prevent memory leaks.
Best Practices for Dynamic Memory Allocation
When working with dynamic memory allocation in C, it's important to follow these best practices to ensure efficient and safe memory management:
- Always check if memory allocation was successful:
int *ptr = (int *)malloc(10 * sizeof(int));
if (ptr == NULL) {
// Handle allocation failure
fprintf(stderr, "Memory allocation failed\n");
exit(1);
}
- Free allocated memory when it's no longer needed:
free(ptr);
ptr = NULL; // Set the pointer to NULL after freeing
- Avoid accessing memory after it has been freed:
free(ptr);
// Don't do this:
// *ptr = 5; // This is undefined behavior
- Be cautious with realloc():
int *new_ptr = (int *)realloc(ptr, new_size);
if (new_ptr != NULL) {
ptr = new_ptr;
} else {
// Handle reallocation failure
// Original ptr is still valid
}
- Use sizeof() for portability:
int *ptr = (int *)malloc(10 * sizeof(int));
- Initialize memory after allocation if necessary:
memset(ptr, 0, 10 * sizeof(int));
Common Pitfalls and How to Avoid Them
Dynamic memory allocation, while powerful, can lead to several common issues if not handled properly. Here are some pitfalls to watch out for:
Memory Leaks
Memory leaks occur when allocated memory is not freed, leading to a gradual loss of available memory. To avoid memory leaks:
- Always free dynamically allocated memory when it's no longer needed
- Use tools like Valgrind to detect memory leaks
- Implement proper error handling to ensure memory is freed in all code paths
Buffer Overflows
Buffer overflows happen when writing beyond the bounds of allocated memory. To prevent buffer overflows:
- Always allocate sufficient memory for your data
- Use bounds checking when accessing dynamically allocated arrays
- Consider using safer alternatives like
calloc()
which initializes memory to zero
Use After Free
Using memory after it has been freed can lead to undefined behavior. To avoid this:
- Set pointers to NULL after freeing them
- Implement checks to ensure memory is not accessed after being freed
- Use tools like AddressSanitizer to detect use-after-free errors
Double Free
Attempting to free memory that has already been freed can cause crashes or corruption. To prevent double frees:
- Set pointers to NULL after freeing them
- Implement checks to ensure memory is not freed twice
- Use tools like AddressSanitizer to detect double-free errors
Advanced Techniques
For more advanced memory management in C, consider exploring these techniques:
Custom Memory Allocators
Implementing a custom memory allocator can provide better performance and control over memory usage for specific use cases.
Memory Pools
Memory pools can be used to efficiently allocate and deallocate fixed-size blocks of memory, reducing fragmentation and improving performance.
Reference Counting
Implementing a simple reference counting system can help manage the lifetime of dynamically allocated objects more effectively.
Comparison with Other Languages
Unlike C, many modern programming languages handle memory management automatically through garbage collection. While this removes the burden of manual memory management from the programmer, it can come at the cost of performance and predictability.
Garbage Collection
Languages like Java, Python, and C# use garbage collection to automatically free memory that is no longer in use. This approach has several advantages:
- Reduces the risk of memory leaks and other memory-related bugs
- Simplifies memory management for the programmer
- Can lead to more productive development
However, garbage collection also has some drawbacks:
- Can introduce unpredictable pauses in program execution
- May use more memory than manual management
- Less control over when memory is freed
Manual Memory Management in C
C's approach to manual memory management offers several benefits:
- Fine-grained control over memory usage
- Predictable performance
- Efficient use of system resources
But it also comes with challenges:
- Requires careful attention to memory allocation and deallocation
- More prone to memory-related bugs if not handled correctly
- Can be more time-consuming to implement correctly
Conclusion
Dynamic memory allocation is a powerful feature in C that allows for flexible and efficient use of memory resources. By understanding the concepts of the stack and heap, mastering the use of functions like malloc()
, calloc()
, realloc()
, and free()
, and following best practices, you can write more robust and efficient C programs.
Remember that with great power comes great responsibility. Manual memory management in C requires careful attention to detail and a thorough understanding of memory-related concepts. By avoiding common pitfalls and implementing proper error handling, you can harness the full potential of dynamic memory allocation while maintaining the stability and performance of your C programs.
As you continue to develop your skills in C programming, consider exploring more advanced memory management techniques and comparing C's approach with that of other programming languages. This broader perspective will help you make informed decisions about when and how to use dynamic memory allocation effectively in your projects.
Article created from: https://www.youtube.com/watch?v=R0qIYWo8igs