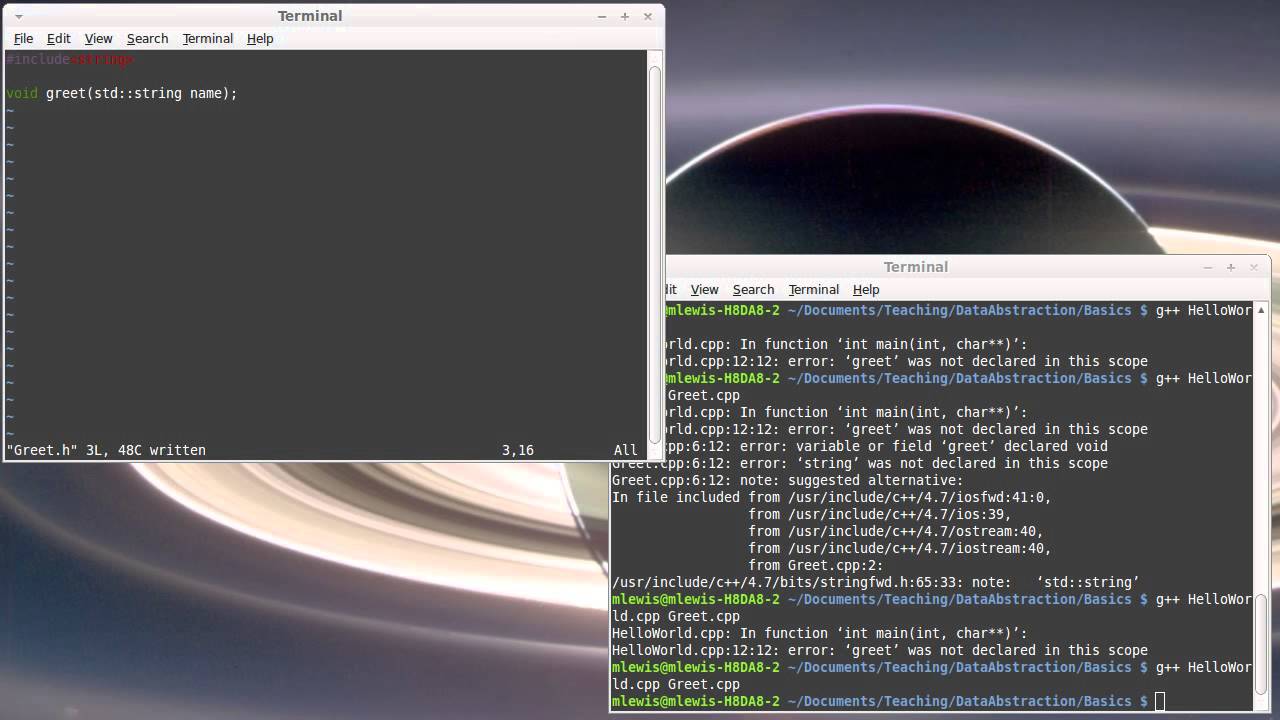
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Multi-File C++ Programming
As C++ programs grow in complexity, it becomes essential to organize code across multiple files. This approach not only improves code readability but also enhances maintainability and allows for more efficient compilation processes. In this comprehensive guide, we'll explore the intricacies of multi-file programming in C++, focusing on the proper use of header files, compilation techniques, and best practices for structuring your projects.
The Basics of Multi-File C++ Programs
When working with larger C++ projects, it's common to split the code into multiple files. This separation allows for better organization and makes it easier to manage different components of your program. Let's start by examining a simple example of a multi-file C++ program.
Creating Separate Source Files
In our example, we'll create two main source files:
-
hello_world.cpp
: This will contain our main function and serve as the entry point of our program. -
greet.cpp
: This file will contain a function that handles greeting the user.
Let's begin with the content of greet.cpp
:
#include <iostream>
#include <string>
void greet(std::string name) {
std::cout << "Hello, " << name << "!";
}
In this file, we've defined a simple greet
function that takes a string
parameter and prints a greeting message.
Now, let's look at the content of hello_world.cpp
:
#include <iostream>
#include <string>
int main() {
std::string name;
std::cout << "Enter your name: ";
std::cin >> name;
greet(name);
return 0;
}
This file contains our main
function, which prompts the user for their name and then calls the greet
function.
The Importance of Header Files
While we've created separate source files, our program won't compile as is. The main issue is that the hello_world.cpp
file doesn't know about the greet
function defined in greet.cpp
. This is where header files come into play.
Header files serve as a bridge between different source files, providing declarations of functions, classes, and variables that can be used across multiple files. They allow the compiler to know about the existence and structure of code elements without needing the full implementation.
Creating a Header File
Let's create a header file for our greet
function. We'll name it greet.h
:
#ifndef GREET_H
#define GREET_H
#include <string>
void greet(std::string name);
#endif // GREET_H
Let's break down the components of this header file:
- The
#ifndef
,#define
, and#endif
directives form an include guard. This prevents multiple inclusions of the same header file, which can lead to compilation errors. - We include the
<string>
header because our function usesstd::string
. - We provide the declaration (not definition) of the
greet
function.
Using the Header File
Now, we need to modify our source files to use this header file:
In greet.cpp
:
#include "greet.h"
#include <iostream>
void greet(std::string name) {
std::cout << "Hello, " << name << "!";
}
In hello_world.cpp
:
#include <iostream>
#include <string>
#include "greet.h"
int main() {
std::string name;
std::cout << "Enter your name: ";
std::cin >> name;
greet(name);
return 0;
}
By including greet.h
in both files, we ensure that the compiler knows about the greet
function in all relevant parts of our program.
The Compilation Process
Understanding the compilation process is crucial when working with multi-file C++ programs. The process typically involves two main steps: compilation and linking.
Compilation
During the compilation phase, each source file (.cpp) is compiled separately into an object file (.o). This step checks for syntax errors and generates machine code, but it doesn't create an executable yet.
To compile our files individually, we can use the following commands:
g++ -c hello_world.cpp
g++ -c greet.cpp
The -c
flag tells the compiler to generate object files without linking.
Linking
After compilation, the linker combines the object files into a single executable. This step resolves references between the different parts of your program.
To link our object files and create an executable, we use:
g++ hello_world.o greet.o -o my_program
This command creates an executable named my_program
.
One-Step Compilation and Linking
For smaller projects, you can often combine compilation and linking into a single step:
g++ hello_world.cpp greet.cpp -o my_program
This command compiles both source files and links them into an executable in one go.
Best Practices for Multi-File C++ Programming
As you work with larger C++ projects, keep these best practices in mind:
-
Use Header Guards: Always include header guards in your .h files to prevent multiple inclusions.
-
Minimize Inclusions: Only include what's necessary in each file. This reduces compilation time and potential conflicts.
-
Forward Declarations: When possible, use forward declarations in headers instead of including other headers.
-
Separate Interface from Implementation: Use header files for declarations (the interface) and .cpp files for definitions (the implementation).
-
Consistent Naming: Use consistent naming conventions for your files. For example, if you have a class named
MyClass
, name the filesmy_class.h
andmy_class.cpp
. -
Organize Your Project: Group related files into directories as your project grows.
-
Use Include Paths: Set up your build system to use include paths rather than relying on relative paths in your #include statements.
Advanced Topics in Multi-File C++ Programming
As you become more comfortable with basic multi-file programming, there are several advanced topics you might want to explore:
Precompiled Headers
For large projects, using precompiled headers can significantly reduce compilation times. Precompiled headers allow you to compile a set of headers once and reuse the compiled version in subsequent compilations.
Template Implementation
When working with templates, you often need to include the implementation in the header file. This is because the compiler needs to see the full template definition to generate code for specific template instantiations.
Inline Functions
Inline functions are typically defined in header files. The inline
keyword suggests to the compiler that it should try to replace function calls with the function body directly, which can improve performance for small, frequently-called functions.
Namespaces
Using namespaces can help organize your code and prevent naming conflicts in larger projects. You can declare namespaces in header files and define them across multiple source files.
Common Pitfalls and How to Avoid Them
When working with multi-file C++ programs, there are several common pitfalls that developers often encounter. Being aware of these can save you time and frustration:
Circular Dependencies
Circular dependencies occur when two or more files include each other, directly or indirectly. This can lead to compilation errors or unexpected behavior.
Solution: Use forward declarations where possible, and restructure your code to break circular dependencies.
Multiple Definitions
Defining the same function or variable in multiple source files can lead to linker errors.
Solution: Ensure that functions and global variables are defined only once, typically in a .cpp file, and declared in a header file.
Inconsistent Declarations
Having different declarations for the same entity in different files can lead to subtle bugs or compilation errors.
Solution: Always use a single header file for declarations and include this header wherever the declaration is needed.
Large Header Files
Including large header files can significantly increase compilation times.
Solution: Keep headers as small as possible. Consider using forward declarations and moving implementations to source files.
Forgetting to Update Headers
When you change a function signature or class definition in a .cpp file, it's easy to forget to update the corresponding header file.
Solution: Develop a habit of updating both the header and source file together when making changes.
Build Systems and Multi-File Projects
As your projects grow, managing compilation and linking manually becomes impractical. This is where build systems come in handy. Some popular build systems for C++ include:
Make
Make is a classic build automation tool that uses Makefiles to define how to derive the target program from its dependencies.
CMake
CMake is a cross-platform build system generator. It can generate native makefiles and workspaces that can be used in the compiler environment of your choice.
Ninja
Ninja is a small build system with a focus on speed. It's often used in conjunction with CMake.
Visual Studio
For Windows developers, Visual Studio provides a comprehensive IDE with built-in build capabilities.
Using a build system allows you to:
- Automate the compilation and linking process
- Manage dependencies between files
- Compile only the files that have changed since the last build
- Configure different build types (e.g., debug, release)
- Manage complex projects with multiple directories and libraries
Debugging Multi-File Programs
Debugging multi-file programs can be more challenging than single-file programs. Here are some tips to make the process easier:
-
Use a Debugger: Tools like GDB (GNU Debugger) or Visual Studio's debugger allow you to step through your code across multiple files.
-
Set Breakpoints: Place breakpoints at key points in your program, especially at the boundaries between different files.
-
Examine Call Stacks: When you hit a breakpoint, examine the call stack to understand how you got there through different files.
-
Use Logging: Implement a logging system that can help you trace the flow of your program across different files.
-
Modular Testing: Test individual components (functions, classes) in isolation before integrating them into the larger program.
Performance Considerations in Multi-File Programs
While multi-file programs offer better organization, they can impact performance if not managed properly:
-
Compilation Time: More files generally mean longer compilation times. Use techniques like precompiled headers to mitigate this.
-
Link-Time Optimization (LTO): Modern compilers offer LTO, which can optimize across translation units (i.e., across different source files).
-
Inlining Across Files: The compiler may have difficulty inlining functions defined in different files. Consider using inline functions in headers for performance-critical code.
-
Header Organization: Organize your headers to minimize the number of includes needed in each file. This can improve compilation speed and reduce the risk of circular dependencies.
Conclusion
Mastering multi-file programming in C++ is crucial for developing large, maintainable projects. By understanding the role of header files, the compilation process, and best practices, you can create well-structured programs that are easier to develop, debug, and maintain.
Remember that the key to successful multi-file programming lies in proper organization, clear separation of concerns, and a good understanding of the language and tools at your disposal. As you work on larger projects, continue to refine your approach and explore advanced techniques to improve your C++ programming skills.
With practice, you'll find that multi-file programming becomes second nature, allowing you to tackle complex projects with confidence and efficiency. Keep exploring, keep learning, and most importantly, keep coding!
Article created from: https://youtu.be/D0TazQIkc8Q