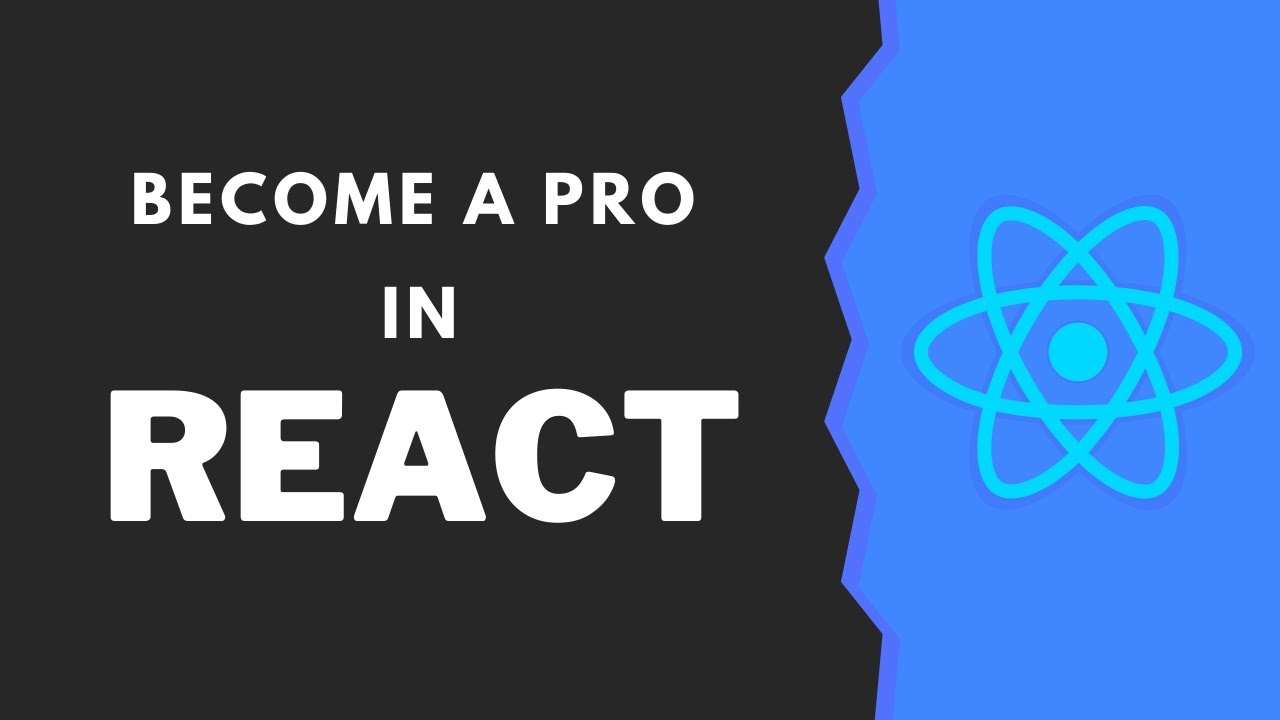
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeEmbrace Optional Chaining for Cleaner Code
One of the lesser-utilized features among beginner React developers is optional chaining. This feature, while relatively new, can significantly simplify how you handle undefined or null values in your objects. By simply adding a ?.
before accessing an object's property, you ensure that your code doesn’t crash if the object turns out to be null or undefined. Instead of writing bulky if statements to check if an object exists before accessing its properties, optional chaining allows for a more concise and readable syntax. This method proves especially useful when dealing with data that might not always be consistent, such as API responses.
Understanding null
vs. undefined
Before diving deeper into optional chaining, it's crucial to understand the difference between a variable with a null
value and one that is undefined
. A null
value indicates that a variable has been explicitly assigned to have no value, whereas undefined
means a variable has been declared but not assigned any value yet. This distinction is important because it influences how you handle potentially absent data in your React components.
Leveraging Optional Chaining in Real Scenarios
Handling API Data Gracefully
When fetching data from an API, you might initially receive a null
value while the request is pending. Traditional methods might involve conditional rendering or initializing your state with an empty array to avoid errors. However, optional chaining offers a more elegant solution. By simply adding a ?
before accessing properties or calling methods on potentially null objects, you can avoid unnecessary conditional checks and make your code cleaner and more readable.
Enhancing Component Flexibility with Props
Optional chaining isn't limited to object properties; it also extends to handling optional props in components. This approach allows you to conditionally call functions or access properties from props without worrying about them being undefined or null. This technique can significantly reduce the amount of defensive coding required, making your components more concise and easier to maintain.
Adopting Composition Over Inheritance
Another critical React concept is preferring composition over inheritance for component design. While inheritance might seem like a straightforward way to reuse code, React encourages using composition to achieve more flexible and reusable components. By composing components together rather than extending them, you can create more customizable and maintainable UIs. This approach allows you to pass components as props, leveraging the children
prop to dynamically insert content into your components.
Practical Application of Composition
Imagine you have a Card
component that can display a title, description, and an optional footer. Instead of creating multiple variations of the Card
component for each possible footer configuration, you can use composition to pass the footer as a child component. This method not only simplifies your component structure but also makes it more adaptable to future changes.
The Context API: Use with Caution
The Context API is a powerful tool for state management in React, allowing you to avoid prop drilling by providing a global state accessible throughout your component tree. However, it's essential to use it judiciously. Overusing the Context API can lead to performance issues, especially in large applications with many components. Each time the context's state changes, all components consuming it re-render, potentially leading to unnecessary updates and performance bottlenecks.
When to Use the Context API
The Context API is most effective in small to medium-sized applications or in scenarios where the state does not change frequently. For dynamic states that update often, consider alternative state management solutions or carefully structure your context to minimize performance impacts.
In conclusion, React offers a vast array of features and techniques that can help you write more efficient, cleaner code. By understanding and applying concepts like optional chaining, composition over inheritance, and judicious use of the Context API, you can take your React development skills to the next level. Remember, the key to becoming a better React developer lies in continuous learning and experimenting with new approaches to solve common problems.
For more insightful tips and tricks on React development, don't forget to check out the original video.