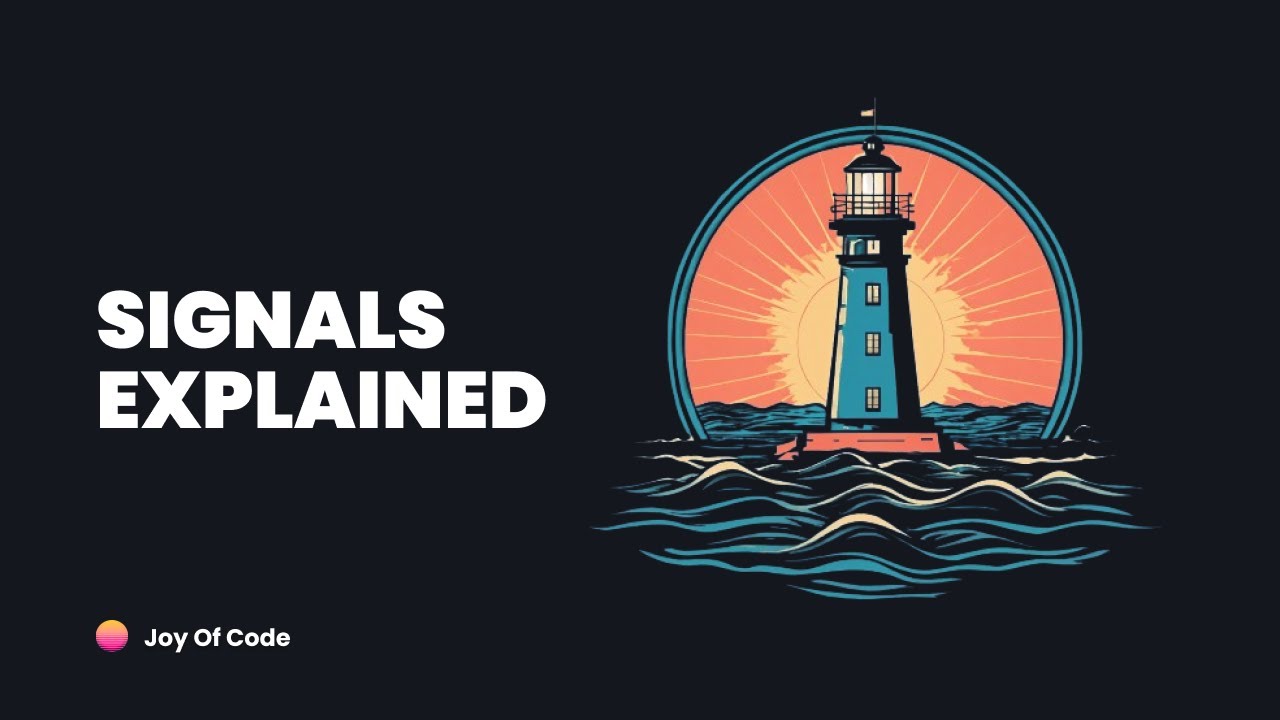
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeUnderstanding Reactivity in JavaScript with Signals and the Observer Pattern
In the evolving world of JavaScript, reactivity has become a central concept that drives modern web development. Reactivity, in simple terms, refers to the ability of a system to update itself automatically in response to changes. This concept is crucial for developing interactive applications where the state changes frequently. One of the foundational elements behind this reactivity is the implementation of signals and their relationship with the Observer pattern.
The Basics of Reactivity and Its Challenges
Reactivity in JavaScript can initially seem straightforward. For instance, you might define a variable count
set to zero and a derived value double
as count * 2
. However, challenges arise when count
changes — the derived value does not automatically update. This illustrates a basic limitation in JavaScript's native reactivity capabilities, which signals seek to overcome.
To address these limitations, developers have historically used patterns like the Observer pattern. This design pattern involves an observable (subject) that maintains a list of dependents (observers). When an event occurs or data changes, the observable notifies all observers about these changes. While effective, this approach can become cumbersome without additional abstractions or utilities.
From Observer Pattern to Signals
The introduction of signals marks a significant evolution from traditional patterns like Observers. Initially inspired by frameworks like Knockout back in 2010, signals provide a more granular approach to reactivity. Unlike Observers that rely on manual subscription and notification mechanisms, signals automate these processes by establishing direct reactive connections between data sources and their consumers.
A signal is essentially an observable with enhanced capabilities. When you interact with a signal by getting or setting its value, it automatically manages subscriptions and notifications. This not only simplifies code but also optimizes performance by minimizing unnecessary computations and updates.
Implementing Basic Reactivity Using Observers
To understand how observers work within JavaScript, consider a simple counter example where clicking a button increments a count displayed onscreen. By implementing an observable class or function that tracks subscribers and their updates, you can manually manage notifications whenever count
changes:
document.querySelector('button').addEventListener('click', function() {
observable.update(count++);
});
This setup demonstrates basic reactivity but requires explicit management of subscriptions and updates — often leading to verbose and error-prone code.
Advancing with Signals for Enhanced Reactivity
Signals simplify observer implementations by automating dependency management. When using signals:
- You define reactive variables as signals.
- Accessing or modifying these variables automatically triggers updates across dependent computations or effects.
- This reduces boilerplate code significantly while ensuring that updates are timely and efficient. For example:
count = signal(0);
count.subscribe(() => console.log(`Count updated to ${count.value}`));
count.value++; // Automatically logs update due to reactive subscription.
The simplicity of signals allows developers to focus more on business logic rather than on maintaining reactivity plumbing. The integration of effects further enhances signal-based architectures by allowing side effects (like UI updates) to be tied directly to state changes without additional overhead. The combination of signals with effects creates powerful patterns for building dynamic user interfaces where state consistency is maintained without manual intervention — making development faster, less error-prone, and more intuitive. The evolution from basic observer patterns towards sophisticated signal systems illustrates significant advancements in handling JavaScript reactivity efficiently — paving the way for more robust web application architectures.
Article created from: https://youtu.be/1TSLEzNzGQM?si=kARBzMkzOm1fYPLY