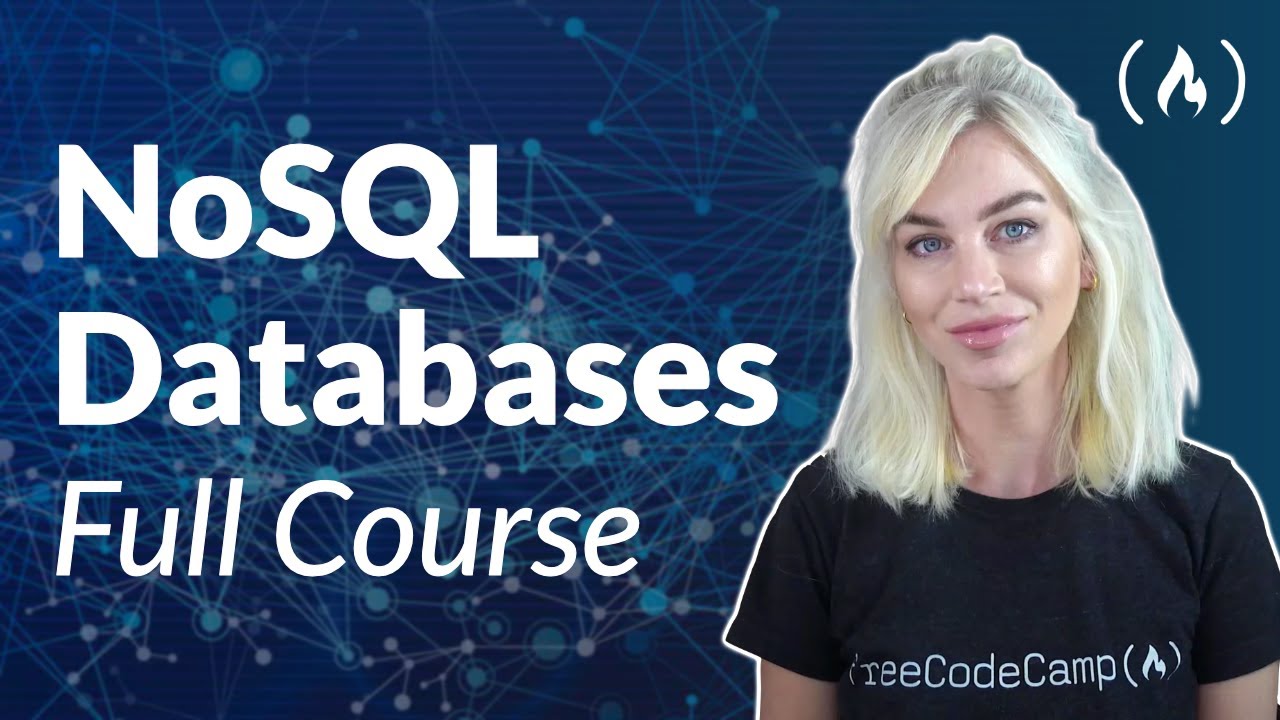
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeNoSQL databases have become increasingly popular for managing large-scale data and building flexible applications. This comprehensive guide will help you understand NoSQL databases, their types, and how to use them in real-world projects.
What is NoSQL?
NoSQL is an approach to database management that allows for a variety of data models, including key-value, document, wide-column (tabular), and graph formats. The defining characteristics of NoSQL databases are:
- Non-relational
- Distributed
- Scalable
- Partition tolerant
- Highly available
NoSQL databases are designed to handle large-scale data and support high transaction throughput while maintaining flexibility in data modeling.
SQL vs NoSQL
While it's tempting to compare SQL and NoSQL, it's important to understand that they are fundamentally different:
- SQL is a structured query language for relational databases
- NoSQL is an approach to database management
NoSQL actually means "not only SQL," as many NoSQL databases have added support for SQL-like querying.
Key differences:
- Data modeling: SQL uses tables with fixed columns and rows, while NoSQL allows for flexible schemas
- Scalability: SQL typically scales vertically, while NoSQL scales horizontally
- Schema: SQL has a fixed schema, while NoSQL offers flexible schemas
Types of NoSQL Databases
There are four main types of NoSQL databases:
- Document databases
- Key-value databases
- Wide-column (tabular) databases
- Graph databases
Let's explore each type in detail.
Document Databases
Document databases store data in flexible, JSON-like documents. They are easy to use and require no schema definition.
Key features:
- Flexible schema
- JSON-like documents
- Easy to scale
Example of a document:
{
"id": "0",
"title": "Fix bike",
"description": "Fix the broken bike in the garage",
"done": false
}
Key-Value Databases
Key-value databases are the simplest type of NoSQL database. They store data as key-value pairs, similar to a dictionary or hash table.
Key features:
- Simple data model
- Fast read/write operations
- Highly scalable
Example of key-value pairs:
Key: "user:1001"
Value: {"name": "John Doe", "email": "[email protected]"}
Key: "product:5678"
Value: {"name": "Smartphone", "price": 599.99}
Wide-Column (Tabular) Databases
Wide-column databases, also known as columnar databases, store data in tables with rows and columns, but with a more flexible structure than traditional relational databases.
Key features:
- Flexible column structure
- Efficient for large-scale data analytics
- Good for time-series data
Example of a wide-column table:
Row Key | Column Family: User Info | Column Family: Orders
--------+------------------------------+------------------------
001 | name: John | age: 30 | order_id: 1001 | total: 99.99
002 | name: Jane | age: 25 | city: NY | order_id: 1002 | total: 149.99
Graph Databases
Graph databases are designed to store and query interconnected data. They use nodes, edges, and properties to represent and store data.
Key features:
- Efficient for querying relationships
- Natural fit for social networks, recommendation engines
- Flexible data model
Example of a graph structure:
(Person: John) -[FRIENDS_WITH]-> (Person: Jane)
(Person: John) -[LIKES]-> (Product: Smartphone)
(Person: Jane) -[WORKS_AT]-> (Company: TechCorp)
Using NoSQL Databases in Real-World Projects
Let's explore how to use NoSQL databases in real-world projects by building two applications: a burger restaurant app using a document database and a hotel app using a graph database.
Project 1: Burger Restaurant App (Document Database)
For this project, we'll use a document database to store information about burger restaurants. We'll use the DataStax Astra DB, which is built on Apache Cassandra, and interact with it using the Document API.
Step 1: Set up the database
- Create a new database in DataStax Astra DB
- Create a new keyspace called "burgers"
- Generate an application token for authentication
Step 2: Create a collection and add documents
Use the Swagger UI to create a collection called "burger_info" and add documents representing burger restaurants:
{
"id": "340",
"name": "Bob's Burgers",
"description": "Tasty burgers from a fictional character from TV",
"ingredients": ["beef patty", "tomato", "cucumber", "lettuce", "cheese"],
"visited": true,
"location": {
"address": "45 Lambda Drive",
"zipCode": 12345,
"webAddress": "www.bobsburgers.com"
}
}
Step 3: Set up the React project
- Create a new React project using Create React App
- Install necessary dependencies (axios, dotenv)
- Set up environment variables for the API endpoint and token
Step 4: Create a serverless function to fetch data
Use Netlify serverless functions to create a secure backend for fetching data from the database:
const fetch = require('node-fetch');
exports.handler = async function() {
const url = process.env.ENDPOINT;
const options = {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'x-cassandra-token': process.env.ASTRA_TOKEN
},
body: JSON.stringify({
query: `{
burgerInfo {
values {
id
name
description
ingredients
visited
location {
address
webAddress
}
}
}
}`
})
};
try {
const response = await fetch(url, options);
const data = await response.json();
return {
statusCode: 200,
body: JSON.stringify(data)
};
} catch (error) {
console.error(error);
return {
statusCode: 500,
body: JSON.stringify({ error: 'Failed to fetch data' })
};
}
};
Step 5: Fetch and display data in the React app
Use the useState
and useEffect
hooks to fetch and display the burger restaurant data:
import React, { useState, useEffect } from 'react';
function App() {
const [burgers, setBurgers] = useState([]);
useEffect(() => {
async function fetchData() {
const response = await fetch('/.netlify/functions/get-burgers');
const data = await response.json();
setBurgers(data.data.burgerInfo.values);
}
fetchData();
}, []);
return (
<div className="App">
<h1>Favorite Burger Restaurants</h1>
<div className="burger-feed">
{burgers.map((burger) => (
<div key={burger.id} className="burger-card">
<h2>{burger.name}</h2>
<p>{burger.description}</p>
<p>{burger.location.address}</p>
<div className={burger.visited ? 'visited' : 'not-visited'}></div>
</div>
))}
</div>
</div>
);
}
export default App;
Project 2: Hotel App (Graph Database)
For this project, we'll use a graph database to store information about hotels. We'll use DataStax Astra DB with the Graph QL API.
Step 1: Set up the database
- Create a new database in DataStax Astra DB
- Create a new keyspace called "hotels"
- Generate an application token for authentication
Step 2: Create a table and add data
Use the Graph QL playground to create a table and add hotel data:
mutation {
createTable(
keyspaceName: "hotels",
tableName: "hotel_data",
partitionKeys: [{ name: "id", type: { basic: TEXT } }]
values: [
{ name: "name", type: { basic: TEXT } },
{ name: "rating", type: { basic: INT } }
]
)
}
mutation {
inserthotel_data(
value: { id: "h1", name: "Abby's Hotel", rating: 4 }
) {
value { name }
}
}
Step 3: Set up the React project
- Create a new React project using Create React App
- Install necessary dependencies (node-fetch, dotenv)
- Set up environment variables for the API endpoint and token
Step 4: Create a serverless function to fetch data
Use Netlify serverless functions to create a secure backend for fetching data from the database:
const fetch = require('node-fetch');
exports.handler = async function() {
const url = process.env.ENDPOINT;
const query = `
query {
hotel_data {
values {
id
name
rating
}
}
}
`;
const options = {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'x-cassandra-token': process.env.ASTRA_TOKEN
},
body: JSON.stringify({ query })
};
try {
const response = await fetch(url, options);
const data = await response.json();
return {
statusCode: 200,
body: JSON.stringify(data)
};
} catch (error) {
console.error(error);
return {
statusCode: 500,
body: JSON.stringify({ error: 'Failed to fetch data' })
};
}
};
Step 5: Fetch and display data in the React app
Use the useState
and useEffect
hooks to fetch and display the hotel data:
import React, { useState, useEffect } from 'react';
function App() {
const [hotels, setHotels] = useState([]);
useEffect(() => {
async function fetchData() {
const response = await fetch('/.netlify/functions/get-hotels');
const data = await response.json();
setHotels(data.data.hotel_data.values);
}
fetchData();
}, []);
return (
<div className="App">
<h1>Hotels</h1>
<div className="hotel-list">
{hotels.map((hotel) => (
<div key={hotel.id} className="hotel">
<h2>{hotel.name}</h2>
<p>Rating: {hotel.rating}</p>
</div>
))}
</div>
</div>
);
}
export default App;
Conclusion
NoSQL databases offer flexible and scalable solutions for managing diverse data types and structures. By understanding the different types of NoSQL databases and their use cases, you can choose the right database for your project requirements.
In this guide, we've covered:
- The basics of NoSQL databases
- The four main types of NoSQL databases
- How to use document and graph databases in real-world projects
To further solidify your knowledge, consider exploring more advanced topics such as:
- Data modeling best practices for NoSQL databases
- Implementing advanced querying and indexing strategies
- Optimizing NoSQL database performance
- Integrating NoSQL databases with other technologies in your stack
By mastering NoSQL databases, you'll be well-equipped to handle a wide range of data management challenges in modern application development.
Article created from: https://www.youtube.com/watch?v=xh4gy1lbL2k