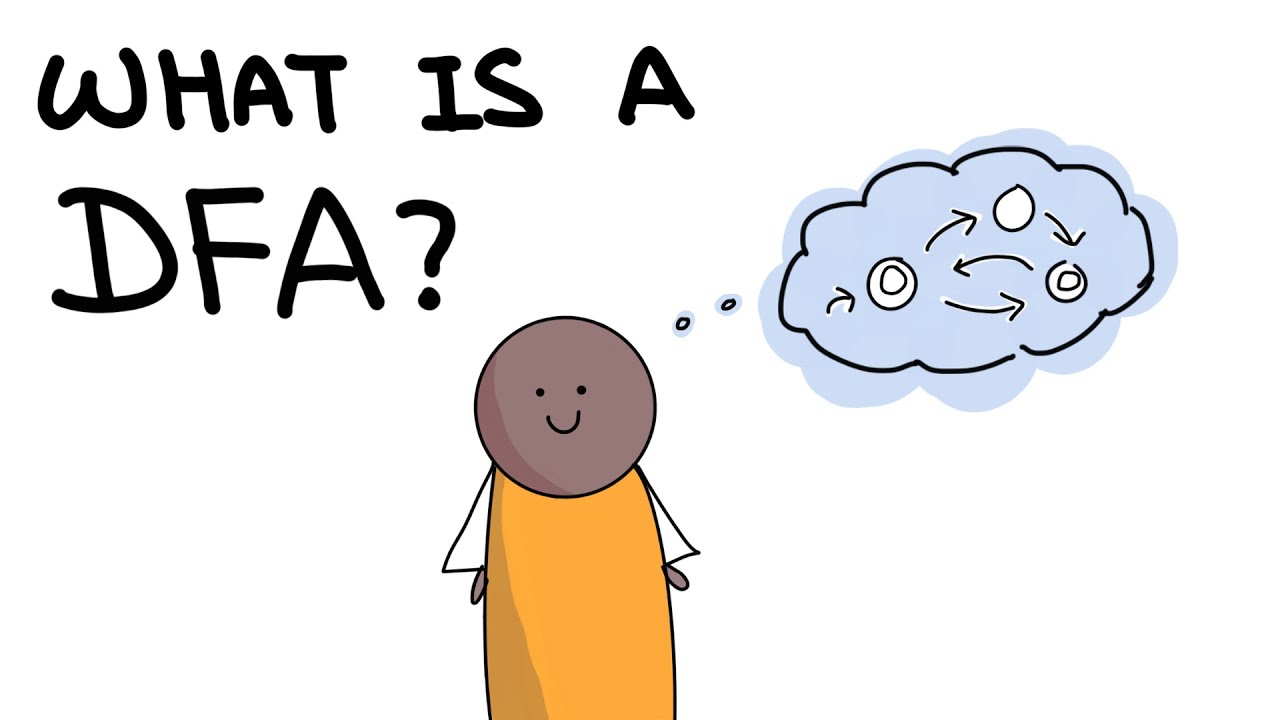
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Finite State Machines
In the realm of computer science and engineering, finite state machines (FSMs) stand as fundamental building blocks of computational theory and practical applications. These elegant yet powerful models of computation find their way into numerous aspects of our daily lives, often operating behind the scenes in devices we use without a second thought.
What is a Finite State Machine?
A finite state machine, also known as a finite automaton, represents the simplest form of computational model we can construct. Despite its limited memory capacity, an FSM can perform a wide array of tasks, making it an invaluable tool in various technological applications.
The term "machine" and "automaton" are often used interchangeably when discussing FSMs, with "automata" being the plural form of automaton. This interchangeability reflects the mechanical nature of these computational models, which operate based on predefined rules and states.
Real-World Applications of Finite State Machines
The Oven Controller: A Simple FSM Example
To grasp the concept of finite state machines, let's start with a familiar household appliance: an oven. The controller responsible for switching an oven on or off serves as a perfect example of a basic finite state machine.
States and Transitions
In this simple FSM:
- There are two states: ON and OFF
- The initial state (also called the start state) is typically OFF for a new oven
- Pressing the control button triggers a transition between states
Here's how it works:
- The oven starts in the OFF state
- When the button is pressed, it transitions to the ON state
- Pressing the button again returns it to the OFF state
This back-and-forth pattern continues, allowing users to control the oven's operation with a single button. The simplicity of this model belies its effectiveness in managing the oven's basic functionality.
Building a More Complex FSM: The Heatwave Detector
While the oven controller demonstrates the basic principles of finite state machines, let's explore a more sophisticated example to showcase the versatility of these computational models. We'll construct an FSM that analyzes weather data to detect the occurrence of a heatwave.
Defining a Heatwave
For this example, we'll use the definition provided by the India Meteorological Department:
A heatwave occurs when maximum temperatures reach 45 degrees Celsius for two consecutive days.
This definition gives us clear parameters to work with in designing our FSM.
Constructing the Heatwave Detector FSM
Let's break down the construction of our heatwave detector FSM:
States
- q0: The start state (normal temperature)
- q1: One day of high temperature (>45°C)
- q2: Two consecutive days of high temperature (heatwave confirmed)
Transitions
- From q0:
- If temperature > 45°C, move to q1
- If temperature ≤ 45°C, stay in q0
- From q1:
- If temperature > 45°C, move to q2 (heatwave detected)
- If temperature ≤ 45°C, return to q0
- From q2:
- Remain in q2 regardless of temperature (heatwave already detected)
Accept State
q2 is designated as the accept state, indicating that a heatwave has occurred. This is typically represented by drawing an additional circle around the state in the diagram.
Simplifying the Input
To make our FSM more manageable, we can simplify the input as follows:
- 1 represents a day with temperature > 45°C
- 0 represents a day with temperature ≤ 45°C
This binary representation allows for easier processing and analysis of the weather data.
Understanding the Language of the FSM
The set of all input strings that lead the FSM to its accept state (q2 in our heatwave detector) is called the language of the machine. In this case, the language consists of all strings containing two consecutive 1s.
Examples of strings in this language include:
- 11
- 110
- 0110
- 100110
The FSM recognizes this language, accepting all strings within it and rejecting those that are not.
Deterministic Finite Automata (DFA)
The heatwave detector we've constructed is an example of a Deterministic Finite Automaton (DFA). The term "deterministic" is crucial here, as it means that for each input symbol read, the next state is always known with certainty.
Formal Definition of a DFA
A DFA is formally defined using a five-tuple notation:
(Q, Σ, δ, q0, F)
Where:
- Q is the finite set of states
- Σ (Sigma) is the finite set of input symbols (alphabet)
- δ (Delta) is the transition function
- q0 is the start state
- F is the set of accept (final) states
For our heatwave detector:
- Q = {q0, q1, q2}
- Σ = {0, 1}
- δ is defined by our transition rules
- q0 is the start state
- F = {q2}
Regular Languages and Finite Automata
A language is considered regular if it can be recognized by some finite automaton. The language of our heatwave detector—all strings containing two consecutive 1s—is a regular language because we have constructed an automaton that recognizes it.
Practical Applications of Finite State Machines
While we've focused on theoretical examples, finite state machines have numerous practical applications in various fields:
1. Digital Circuit Design
FSMs are extensively used in the design of digital circuits, including:
- Control units for CPUs
- Memory management systems
- Traffic light controllers
2. Communication Protocols
Network protocols often use FSMs to manage different states of communication, such as:
- TCP connection establishment
- HTTP request-response cycles
- Bluetooth device pairing
3. Pattern Recognition
FSMs can be employed in various pattern recognition tasks:
- Text processing and searching
- Regular expression matching
- Simple natural language processing tasks
4. Game Development
Video game developers use FSMs to manage:
- Character behavior and AI
- Game state transitions
- User interface interactions
5. Embedded Systems
Many embedded systems rely on FSMs for their operation:
- Vending machines
- Elevator control systems
- Automotive control units
Advantages of Using Finite State Machines
FSMs offer several benefits that make them attractive for both theoretical and practical applications:
-
Simplicity: FSMs are easy to understand and implement, making them accessible to a wide range of developers and engineers.
-
Predictability: The deterministic nature of DFAs ensures consistent behavior, which is crucial for many applications.
-
Efficiency: FSMs can be implemented with minimal computational resources, making them suitable for embedded systems and other resource-constrained environments.
-
Verifiability: The well-defined structure of FSMs allows for formal verification of their behavior, ensuring correctness in critical systems.
-
Modularity: Complex systems can be broken down into multiple interconnected FSMs, promoting modular design and easier maintenance.
Limitations of Finite State Machines
Despite their usefulness, FSMs do have some limitations:
-
Limited Memory: FSMs have no memory beyond their current state, which restricts their ability to handle complex, context-dependent tasks.
-
Scalability Issues: As the number of states and transitions grows, FSMs can become unwieldy and difficult to manage.
-
Inability to Count: Standard FSMs cannot count or perform arithmetic operations, limiting their applicability in certain computational tasks.
-
Lack of Hierarchy: Basic FSMs do not inherently support hierarchical structures, which can make modeling complex systems challenging.
Advanced Concepts in Finite State Machines
While we've covered the basics of DFAs, there are several advanced concepts and variations of finite state machines worth exploring:
Non-deterministic Finite Automata (NFA)
Unlike DFAs, NFAs allow for multiple possible next states for a given input symbol. This non-determinism can simplify the design of certain automata but requires more complex processing.
Mealy and Moore Machines
These are types of FSMs that produce output:
- Mealy machines generate output based on the current state and input
- Moore machines generate output based solely on the current state
Pushdown Automata
An extension of FSMs that includes a stack, allowing for the recognition of context-free languages.
Timed Automata
These incorporate timing constraints into the FSM model, useful for modeling real-time systems.
Designing Effective Finite State Machines
Creating efficient and correct finite state machines is both an art and a science. Here are some tips for designing effective FSMs:
-
Start Simple: Begin with the most basic representation of your problem and gradually add complexity as needed.
-
Identify States Clearly: Ensure each state represents a distinct and meaningful condition in your system.
-
Minimize States: Strive to use the minimum number of states necessary to represent your problem accurately.
-
Consider All Inputs: Account for all possible input combinations, including edge cases and error conditions.
-
Use Tools: Utilize FSM design software and simulators to visualize and test your automata.
-
Document Thoroughly: Provide clear documentation of state meanings, transition conditions, and overall machine behavior.
-
Iterate and Refine: Don't expect to get it perfect on the first try. Continuously test and refine your FSM design.
Conclusion
Finite state machines represent a fundamental concept in computer science and engineering, bridging theoretical computation and practical application. From the simple on-off controller of an oven to complex weather pattern analyzers, FSMs demonstrate their versatility and power in modeling a wide range of systems and processes.
Key takeaways from our exploration of finite state machines include:
- DFAs are defined by five-tuples and can be visually represented with state diagrams.
- A machine accepts a string if the final symbol causes it to end in an accept state.
- The language of a machine encompasses all strings it accepts.
- Any language recognized by a finite automaton is classified as a regular language.
As we've seen, designing automata is a creative process that requires practice and patience. Like any form of design or engineering, crafting effective finite state machines is a skill that develops over time. By understanding the principles and applications of FSMs, we gain valuable insights into the fundamental nature of computation and the elegant solutions it offers to complex problems.
Whether you're a computer scientist, an engineer, or simply curious about the inner workings of the devices around you, the study of finite state machines opens up a fascinating world of computational theory and practical problem-solving. As technology continues to advance, the principles embodied in these simple yet powerful models will undoubtedly continue to play a crucial role in shaping the systems and devices of the future.
Article created from: https://www.youtube.com/watch?v=PK3wL7DXuuw&list=PLhqug0UEsC-IDomfNsn8e3neoy34o8oye&index=3