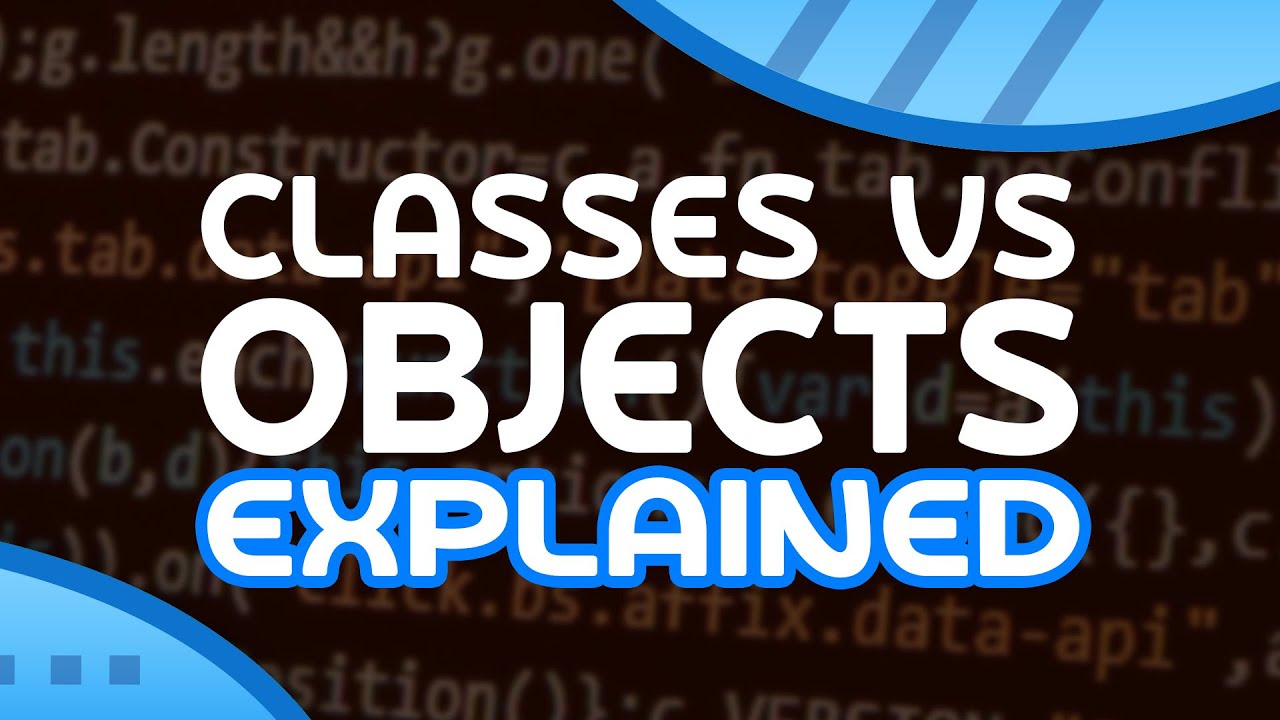
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Classes and Objects in Python
Python is an object-oriented programming language, which means it relies heavily on the concepts of classes and objects. For many beginners, understanding the distinction between these two fundamental concepts can be challenging. This comprehensive guide aims to clarify the difference between classes and objects in Python, providing you with a solid foundation for object-oriented programming.
The Basics: Variables and Types
Before diving into classes and objects, let's start with the basics of variables and types in Python. When we create variables in Python, each variable has a specific type. For example:
x = 1
y = "Hello"
In this case, x
is of type int
(integer), and y
is of type str
(string). These types are actually classes built into the Python language. When we create a variable, we're essentially creating an object of a particular class.
Classes as Blueprints
A class in Python can be thought of as a blueprint or a template. It defines the structure and behavior that objects of that class will have. Think of a class as a set of instructions for creating objects.
For example, let's consider a simple Dog
class:
class Dog:
def __init__(self, name, age, breed):
self.name = name
self.age = age
self.breed = breed
def bark(self):
print("Woof!")
def get_info(self):
return f"{self.name} is a {self.age} year old {self.breed}."
This class defines the attributes (name, age, breed) and methods (bark, get_info) that all Dog objects will have.
Objects as Instances
An object is an instance of a class. It's a concrete entity created from the class blueprint. Each object has its own set of attributes, but shares the methods defined in the class.
Using our Dog
class, we can create dog objects like this:
dog1 = Dog("Buddy", 3, "Labrador")
dog2 = Dog("Max", 5, "German Shepherd")
Here, dog1
and dog2
are separate objects, each with their own set of attributes (name, age, breed), but they share the same methods (bark, get_info) defined in the Dog
class.
The Relationship Between Classes and Objects
The relationship between classes and objects is crucial to understand:
-
Classes define structure and behavior: A class outlines what attributes and methods its objects will have.
-
Objects are instances of classes: Each object is a unique instance of a class, with its own set of attribute values.
-
Objects inherit behavior from classes: All objects of a class can use the methods defined in that class.
-
Multiple objects can be created from one class: You can create as many objects as you need from a single class definition.
Built-in Classes in Python
Python has many built-in classes that we use regularly, often without realizing it. For example:
-
int
for integers -
str
for strings -
list
for lists -
dict
for dictionaries
When we create a variable like x = 5
, we're actually creating an object of the int
class. The int
class defines all the operations we can perform on integers, such as addition, subtraction, etc.
The __init__ Method
In our Dog
class example, you might have noticed the __init__
method. This is a special method in Python classes, often referred to as a constructor. It's called when an object is created and is used to initialize the object's attributes.
The self
parameter in the __init__
method (and in other methods) refers to the instance being created or operated on. It's how the object keeps track of its own attributes and methods.
Class Methods vs Instance Methods
Methods defined in a class can be either class methods or instance methods:
-
Instance methods operate on individual objects and have access to the object's attributes via
self
. - Class methods operate on the class itself and are often used for operations that are not specific to any individual object.
In our Dog
class example, bark()
and get_info()
are instance methods because they operate on individual dog objects.
Attributes: Class vs Instance
Attributes in Python can be associated with either the class or individual instances:
- Class attributes are shared by all instances of the class.
- Instance attributes are unique to each instance of the class.
In our Dog
class, name
, age
, and breed
are instance attributes because they're specific to each dog object.
Inheritance
Inheritance is a powerful feature of object-oriented programming that allows a new class to be based on an existing class. The new class (subclass) inherits attributes and methods from the existing class (superclass).
For example, we could create a Puppy
class that inherits from our Dog
class:
class Puppy(Dog):
def __init__(self, name, age, breed, training_status):
super().__init__(name, age, breed)
self.training_status = training_status
def play(self):
print(f"{self.name} is playing!")
The Puppy
class inherits all attributes and methods from Dog
, and adds its own attribute (training_status
) and method (play()
).
Encapsulation
Encapsulation is the bundling of data and the methods that operate on that data within a single unit (i.e., a class). It's a way of restricting direct access to some of an object's components, which is a fundamental principle of object-oriented programming.
In Python, we can use double underscores to create "private" attributes:
class BankAccount:
def __init__(self, balance):
self.__balance = balance
def deposit(self, amount):
self.__balance += amount
def withdraw(self, amount):
if amount <= self.__balance:
self.__balance -= amount
return True
return False
def get_balance(self):
return self.__balance
In this example, __balance
is a private attribute that can't be accessed directly from outside the class. Instead, we provide methods to interact with it (deposit
, withdraw
, get_balance
).
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It's the ability of different classes to respond to the same method call, possibly in different ways.
For example:
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
animals = [Dog(), Cat()]
for animal in animals:
print(animal.speak())
Here, both Dog
and Cat
classes have a speak()
method, but they implement it differently. We can call speak()
on any Animal
object, and the correct implementation will be used based on the actual type of the object.
Abstract Classes
An abstract class is a class that's meant to be inherited from, but not instantiated directly. It often contains one or more abstract methods - methods that are declared, but don't have an implementation.
Python doesn't have built-in abstract classes, but we can use the abc
module to create them:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius ** 2
Here, Shape
is an abstract class with an abstract area()
method. The Circle
class inherits from Shape
and provides an implementation for area()
.
Static Methods
Static methods are methods that belong to a class rather than an instance of the class. They don't have access to the instance (self
) or the class (cls
) and work like regular functions but are defined inside a class.
class MathOperations:
@staticmethod
def add(x, y):
return x + y
print(MathOperations.add(5, 3)) # Outputs: 8
Static methods are often used for utility functions that are related to the class but don't need to access instance-specific data.
Properties
Properties allow you to use methods like attributes. They're a way to add getter, setter, and deleter functionality to class attributes:
class Temperature:
def __init__(self, celsius):
self._celsius = celsius
@property
def fahrenheit(self):
return (self._celsius * 9/5) + 32
@fahrenheit.setter
def fahrenheit(self, value):
self._celsius = (value - 32) * 5/9
temp = Temperature(25)
print(temp.fahrenheit) # Outputs: 77.0
temp.fahrenheit = 100
print(temp._celsius) # Outputs: 37.77777777777778
Here, fahrenheit
is a property that allows us to get and set the temperature in Fahrenheit, while internally storing it in Celsius.
Conclusion
Understanding the difference between classes and objects is crucial for mastering object-oriented programming in Python. Classes serve as blueprints, defining the structure and behavior of objects. Objects are instances of classes, each with their own set of attribute values but sharing the methods defined in the class.
By grasping these concepts, you'll be better equipped to design and implement complex systems using object-oriented principles. Remember, practice is key to fully understanding these concepts. Try creating your own classes and objects, experiment with inheritance and polymorphism, and you'll soon find yourself comfortable with these powerful programming paradigms.
As you continue your Python journey, you'll discover that classes and objects are fundamental to many advanced concepts and design patterns in software development. They allow for code reuse, modularity, and the creation of complex systems that model real-world entities and relationships.
Keep exploring, keep coding, and most importantly, keep learning!
Article created from: https://youtu.be/BM9tPve8T1o