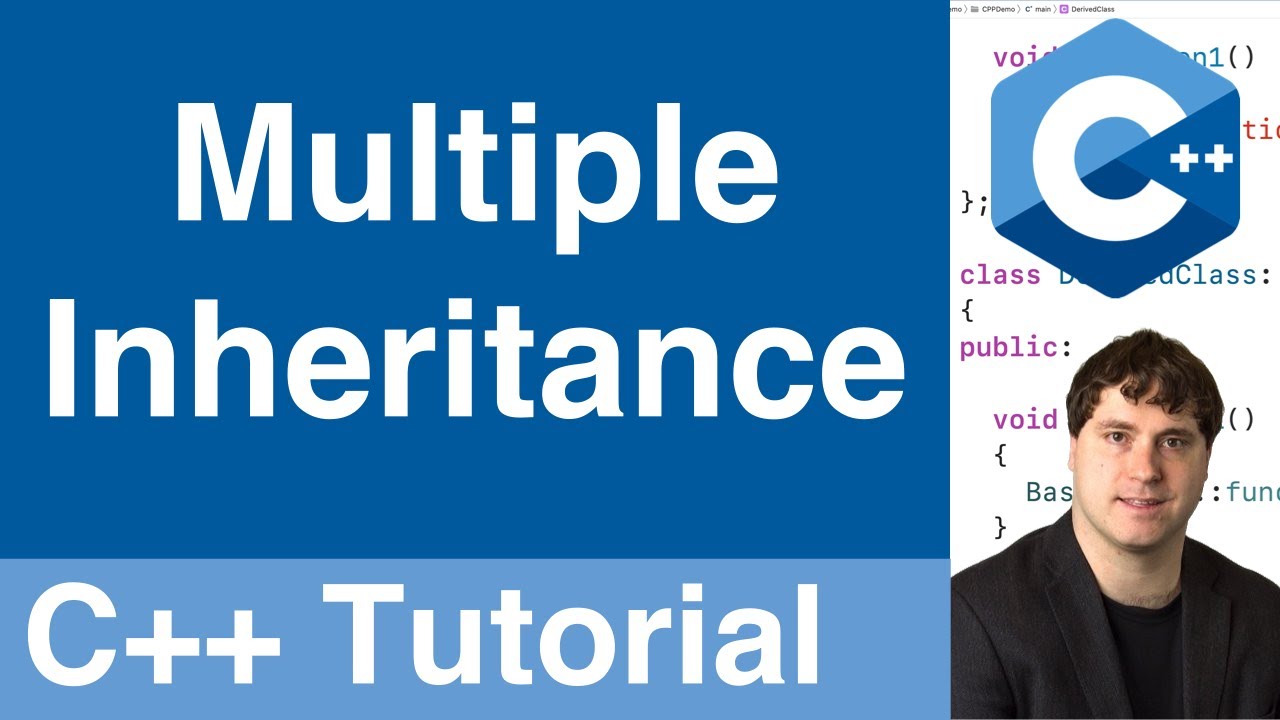
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Multiple Inheritance in C++
Multiple inheritance is a feature in C++ that allows a derived class to inherit from more than one base class. This powerful capability can lead to more flexible and expressive code designs, but it also introduces several complexities that developers need to be aware of. In this comprehensive guide, we'll delve into the intricacies of multiple inheritance, explore potential issues, and discuss practical solutions.
Basic Multiple Inheritance
Let's start with a simple example to illustrate how multiple inheritance works in C++:
class BaseClass1 {
public:
void function1() {
cout << "Function1 BaseClass1" << endl;
}
};
class BaseClass2 {
public:
void function2() {
cout << "Function2 BaseClass2" << endl;
}
};
class DerivedClass : public BaseClass1, public BaseClass2 {
public:
void function3() {
cout << "Function3 DerivedClass" << endl;
}
};
In this example, DerivedClass
inherits from both BaseClass1
and BaseClass2
. It gains access to function1()
from BaseClass1
and function2()
from BaseClass2
, while also defining its own function3()
.
We can create an instance of DerivedClass
and call all three functions:
DerivedClass derived;
derived.function1();
derived.function2();
derived.function3();
This code demonstrates the basic concept of multiple inheritance, where a derived class can access and use members from multiple base classes.
Ambiguity in Multiple Inheritance
While multiple inheritance offers flexibility, it can lead to ambiguity issues. Let's examine a scenario where this might occur:
class BaseClass1 {
public:
void function1() {
cout << "Function1 BaseClass1" << endl;
}
};
class BaseClass2 {
public:
void function1() {
cout << "Function1 BaseClass2" << endl;
}
};
class DerivedClass : public BaseClass1, public BaseClass2 {
// No override for function1()
};
In this case, both base classes have a function1()
, but DerivedClass
doesn't override it. If we try to call function1()
on a DerivedClass
object, we'll encounter an ambiguity error:
DerivedClass derived;
derived.function1(); // Error: Ambiguous call to function1()
Resolving Ambiguity
There are several ways to resolve this ambiguity:
-
Scope Resolution Operator: We can explicitly specify which base class's function to call:
derived.BaseClass1::function1(); // Calls BaseClass1's function1() derived.BaseClass2::function1(); // Calls BaseClass2's function1()
-
Override in Derived Class: We can provide an implementation in the derived class that calls the desired base class function:
class DerivedClass : public BaseClass1, public BaseClass2 { public: void function1() { BaseClass1::function1(); // Calls BaseClass1's function1() } };
-
Using declaration: We can use a using declaration to bring one of the base class functions into the derived class's scope:
class DerivedClass : public BaseClass1, public BaseClass2 { public: using BaseClass1::function1; // Resolves ambiguity in favor of BaseClass1's function1() };
The Diamond Problem
The diamond problem is a more complex issue that can arise with multiple inheritance. It occurs when a derived class inherits from two classes that both inherit from a common base class. This creates a diamond-shaped inheritance hierarchy:
CommonBase
/ \
/ \
Base1 Base2
\ /
\ /
DerivedClass
Here's an example that illustrates the diamond problem:
class CommonBase {
public:
int commonValue;
};
class Base1 : public CommonBase {};
class Base2 : public CommonBase {};
class DerivedClass : public Base1, public Base2 {};
In this scenario, DerivedClass
inherits commonValue
twice, once through Base1
and once through Base2
. This leads to ambiguity when trying to access commonValue
from a DerivedClass
object:
DerivedClass derived;
derived.commonValue = 10; // Error: Ambiguous access
Solving the Diamond Problem
The solution to the diamond problem in C++ is to use virtual inheritance:
class Base1 : virtual public CommonBase {};
class Base2 : virtual public CommonBase {};
class DerivedClass : public Base1, public Base2 {};
By using virtual
inheritance, we ensure that only one instance of CommonBase
is inherited by DerivedClass
, resolving the ambiguity.
Constructor Complexities in Multiple Inheritance
Constructors in multiple inheritance scenarios can be tricky, especially when dealing with inherited constructors from a common base class. Let's examine this with an example:
class CommonBase {
public:
CommonBase(int value) : commonValue(value) {}
CommonBase() : commonValue(-99) {}
int commonValue;
};
class Base1 : virtual public CommonBase {
public:
Base1() : CommonBase(100) {}
};
class Base2 : virtual public CommonBase {
public:
Base2() : CommonBase(200) {}
};
class DerivedClass : public Base1, public Base2 {
public:
DerivedClass() : CommonBase(999) {}
};
In this scenario, Base1
and Base2
both initialize CommonBase
with different values. However, when creating a DerivedClass
object, it's the responsibility of DerivedClass
to initialize CommonBase
directly.
DerivedClass derived;
cout << "Common value: " << derived.commonValue << endl; // Outputs: Common value: 999
This behavior might be surprising at first, but it's designed to avoid ambiguity and ensure that the common base class is initialized only once in the diamond inheritance structure.
Best Practices for Multiple Inheritance
While multiple inheritance can be powerful, it should be used judiciously. Here are some best practices to consider:
-
Use Interfaces: When possible, use abstract base classes (interfaces) for multiple inheritance rather than concrete classes.
-
Avoid Deep Hierarchies: Keep inheritance hierarchies shallow to reduce complexity.
-
Prefer Composition: In many cases, composition can be a cleaner alternative to multiple inheritance.
-
Use Virtual Inheritance: When dealing with diamond inheritance, always use virtual inheritance to avoid ambiguity.
-
Document Clearly: If using multiple inheritance, clearly document the class hierarchy and the purpose of each inheritance relationship.
-
Be Aware of Name Conflicts: Pay attention to potential name conflicts between base classes and resolve them explicitly.
-
Consider Alternative Designs: Before implementing multiple inheritance, consider if there are simpler design patterns that could achieve the same goal.
Conclusion
Multiple inheritance in C++ is a powerful feature that allows for complex class hierarchies and code reuse. However, it comes with its own set of challenges, including ambiguity issues, the diamond problem, and constructor complexities.
By understanding these challenges and knowing how to address them, developers can effectively use multiple inheritance when appropriate. Remember to always consider the trade-offs between the flexibility offered by multiple inheritance and the potential for increased complexity in your code.
As with many advanced features in C++, the key is to use multiple inheritance judiciously, with a clear understanding of its implications and alternatives. When used correctly, it can lead to elegant and efficient code structures, but it should not be the default choice for every design problem.
Continue to practice and experiment with multiple inheritance in various scenarios to gain a deeper understanding of its nuances and best use cases in your C++ projects.
Article created from: https://youtu.be/sswTE0u0r7g