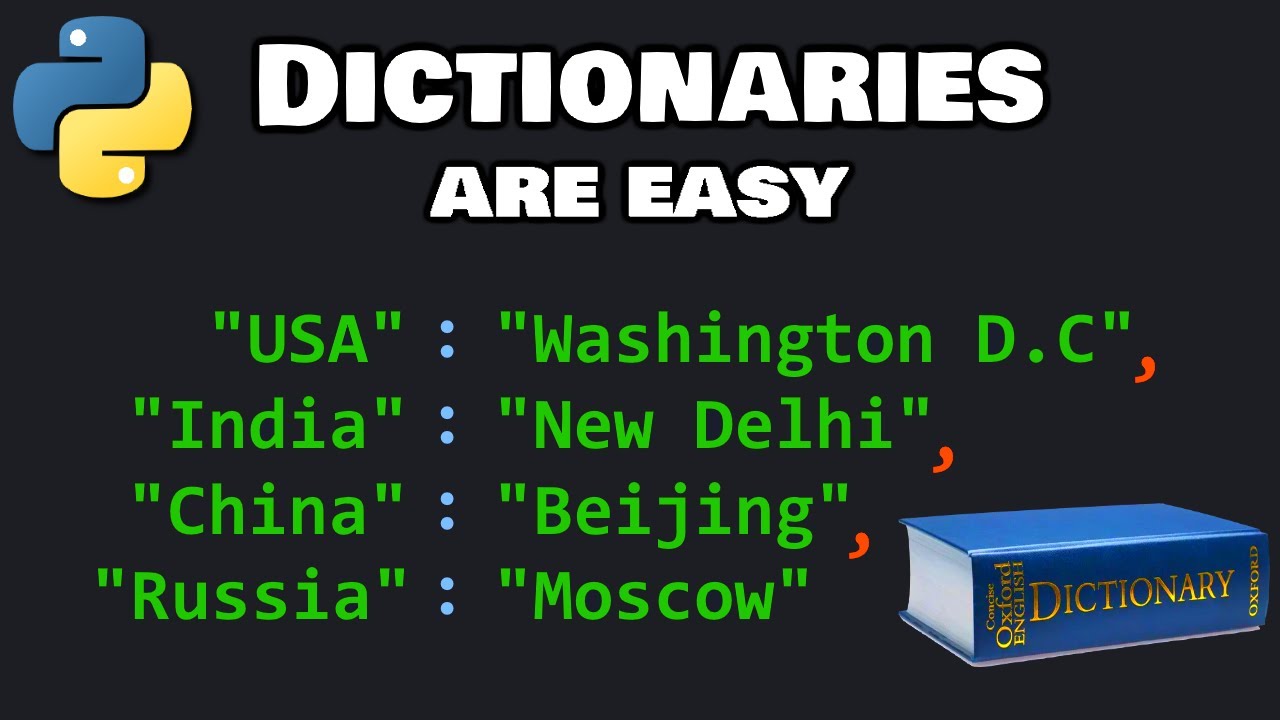
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Python Dictionaries
Python dictionaries are versatile data structures that store key-value pairs. They are one of the four basic collection types in Python, alongside lists, tuples, and sets. Dictionaries are ordered, changeable, and do not allow duplicate keys. This comprehensive guide will walk you through the ins and outs of working with dictionaries in Python.
Understanding Key-Value Pairs
The foundation of a dictionary is the key-value pair. Each key in a dictionary is associated with a specific value. Some examples of key-value pairs include:
- ID and name
- Item and price
- Country and capital
In this guide, we'll focus on creating a dictionary of countries and their capitals.
Creating a Dictionary
To create a dictionary in Python, you use curly braces {}. Here's an example of how to create a dictionary of countries and their capitals:
capitals = {
"USA": "Washington DC",
"India": "New Delhi",
"China": "Beijing",
"Russia": "Moscow"
}
In this example, the countries are the keys, and the capitals are the values.
Accessing Dictionary Information
Using the dir() Function
To see all the attributes and methods available for a dictionary, you can use the dir()
function:
print(dir(capitals))
This will display a list of all the attributes and methods you can use with your dictionary.
Using the help() Function
For more detailed information about dictionary methods and attributes, you can use the help()
function:
help(dict)
This will provide an in-depth description of all dictionary attributes and methods.
Retrieving Values from a Dictionary
The get() Method
To retrieve a value from a dictionary, you can use the get()
method:
print(capitals.get("USA")) # Output: Washington DC
print(capitals.get("India")) # Output: New Delhi
If the key doesn't exist in the dictionary, the get()
method returns None
by default:
print(capitals.get("Japan")) # Output: None
Using get() in Conditional Statements
You can use the get()
method in conditional statements to check if a key exists in the dictionary:
if capitals.get("Japan"):
print("Capital exists")
else:
print("Capital doesn't exist")
if capitals.get("Russia"):
print("Capital exists")
else:
print("Capital doesn't exist")
Modifying Dictionaries
The update() Method
To add new key-value pairs or update existing ones, you can use the update()
method:
capitals.update({"Germany": "Berlin"})
print(capitals)
capitals.update({"USA": "Detroit"})
print(capitals)
Removing Key-Value Pairs
To remove a specific key-value pair, use the pop()
method:
capitals.pop("China")
print(capitals)
To remove the last inserted key-value pair, use the popitem()
method:
capitals.popitem()
print(capitals)
Clearing a Dictionary
To remove all key-value pairs from a dictionary, use the clear()
method:
capitals.clear()
print(capitals)
Working with Keys, Values, and Items
The keys() Method
To get all the keys in a dictionary, use the keys()
method:
keys = capitals.keys()
print(keys)
The keys()
method returns an object that resembles a list. You can iterate over these keys using a for loop:
for key in capitals.keys():
print(key)
The values() Method
To get all the values in a dictionary, use the values()
method:
values = capitals.values()
print(values)
for value in capitals.values():
print(value)
The items() Method
The items()
method returns a view object that contains tuples of key-value pairs:
items = capitals.items()
print(items)
for key, value in capitals.items():
print(f"{key}: {value}")
Advanced Dictionary Techniques
Dictionary Comprehension
Dictionary comprehension is a concise way to create dictionaries:
squares = {x: x**2 for x in range(6)}
print(squares)
Merging Dictionaries
In Python 3.5+, you can merge dictionaries using the **
operator:
dict1 = {"a": 1, "b": 2}
dict2 = {"c": 3, "d": 4}
merged = {**dict1, **dict2}
print(merged)
Nested Dictionaries
Dictionaries can contain other dictionaries as values:
employees = {
"John": {"id": 1, "position": "Manager"},
"Alice": {"id": 2, "position": "Developer"}
}
print(employees["John"]["position"]) # Output: Manager
Dictionary Methods Overview
Here's a quick reference of the most commonly used dictionary methods:
-
get(key, default)
: Returns the value for the specified key, or a default value if the key doesn't exist. -
update(iterable)
: Updates the dictionary with key-value pairs from another dictionary or iterable. -
pop(key, default)
: Removes and returns the value for the specified key. If the key doesn't exist, returns the default value (or raises KeyError if no default is provided). -
popitem()
: Removes and returns the last inserted key-value pair as a tuple. -
clear()
: Removes all items from the dictionary. -
keys()
: Returns a view object containing all keys in the dictionary. -
values()
: Returns a view object containing all values in the dictionary. -
items()
: Returns a view object containing all key-value pairs as tuples.
Best Practices for Using Dictionaries
-
Use meaningful keys: Choose keys that clearly represent the associated values.
-
Avoid mutable keys: Use immutable types (like strings or tuples) as dictionary keys to prevent unexpected behavior.
-
Handle missing keys: Use the
get()
method ortry-except
blocks to handle cases where a key might not exist in the dictionary. -
Use dictionary comprehension: When appropriate, use dictionary comprehension for more concise and readable code.
-
Consider defaultdict: For dictionaries with default values, consider using
collections.defaultdict
.
Common Use Cases for Dictionaries
-
Caching and memoization: Store computed results for quick lookup.
-
Counting occurrences: Count the frequency of items in a collection.
-
Grouping data: Organize data by specific attributes.
-
Configuration settings: Store application settings in a structured format.
-
JSON-like data structures: Represent hierarchical data in a Python-friendly format.
Performance Considerations
Dictionaries in Python are implemented using hash tables, which provide O(1) average-case time complexity for insertions, deletions, and lookups. This makes them extremely efficient for large datasets.
However, keep in mind that dictionaries consume more memory than lists or tuples due to their hash table structure.
Dictionaries in Python 3.7+
Since Python 3.7, dictionaries maintain insertion order by default. This means that when you iterate over a dictionary, the items will be returned in the order they were inserted.
my_dict = {"c": 3, "a": 1, "b": 2}
for key in my_dict:
print(key, end=" ") # Output: c a b
Dictionary Unpacking
Python 3.5+ introduced dictionary unpacking, which allows you to merge dictionaries easily:
dict1 = {"a": 1, "b": 2}
dict2 = {"c": 3, "d": 4}
merged = {**dict1, **dict2}
print(merged) # Output: {'a': 1, 'b': 2, 'c': 3, 'd': 4}
Type Hinting with Dictionaries
Python 3.5+ introduced type hinting, which can be used with dictionaries to improve code readability and catch potential errors:
from typing import Dict
def process_data(data: Dict[str, int]) -> int:
return sum(data.values())
result = process_data({"a": 1, "b": 2, "c": 3})
print(result) # Output: 6
Dictionary Views
The keys()
, values()
, and items()
methods return view objects, which are dynamic and reflect changes made to the dictionary:
my_dict = {"a": 1, "b": 2}
keys_view = my_dict.keys()
print(keys_view) # Output: dict_keys(['a', 'b'])
my_dict["c"] = 3
print(keys_view) # Output: dict_keys(['a', 'b', 'c'])
Dictionary Comparison
Dictionaries can be compared using the ==
operator. Two dictionaries are considered equal if they have the same key-value pairs, regardless of their order:
dict1 = {"a": 1, "b": 2}
dict2 = {"b": 2, "a": 1}
print(dict1 == dict2) # Output: True
Dictionary Comprehension with Conditions
You can use conditions in dictionary comprehensions to filter key-value pairs:
numbers = range(10)
even_squares = {x: x**2 for x in numbers if x % 2 == 0}
print(even_squares) # Output: {0: 0, 2: 4, 4: 16, 6: 36, 8: 64}
Combining Dictionaries with zip()
The zip()
function can be used to create dictionaries from two iterables:
keys = ["a", "b", "c"]
values = [1, 2, 3]
combined = dict(zip(keys, values))
print(combined) # Output: {'a': 1, 'b': 2, 'c': 3}
Sorting Dictionaries
While dictionaries maintain insertion order in Python 3.7+, you might want to sort them based on keys or values:
# Sorting by keys
sorted_by_key = dict(sorted(my_dict.items()))
# Sorting by values
sorted_by_value = dict(sorted(my_dict.items(), key=lambda item: item[1]))
Using defaultdict
The defaultdict
class from the collections
module can be useful when you want to provide a default value for missing keys:
from collections import defaultdict
word_count = defaultdict(int)
for word in ["apple", "banana", "apple", "cherry"]:
word_count[word] += 1
print(word_count) # Output: defaultdict(<class 'int'>, {'apple': 2, 'banana': 1, 'cherry': 1})
Conclusion
Dictionaries are powerful and flexible data structures in Python. They offer efficient key-value storage and retrieval, making them ideal for a wide range of applications. From basic operations like adding and removing items to more advanced techniques like dictionary comprehension and unpacking, mastering dictionaries will significantly enhance your Python programming skills.
Remember to choose appropriate keys, handle potential KeyErrors, and consider the performance implications when working with large datasets. With practice, you'll find that dictionaries become an indispensable tool in your Python programming toolkit.
As you continue to work with dictionaries, you'll discover even more ways to leverage their power in your Python projects. Whether you're developing data processing scripts, building web applications, or working on machine learning models, a solid understanding of dictionaries will serve you well throughout your Python journey.
Article created from: https://www.youtube.com/watch?v=MZZSMaEAC2g