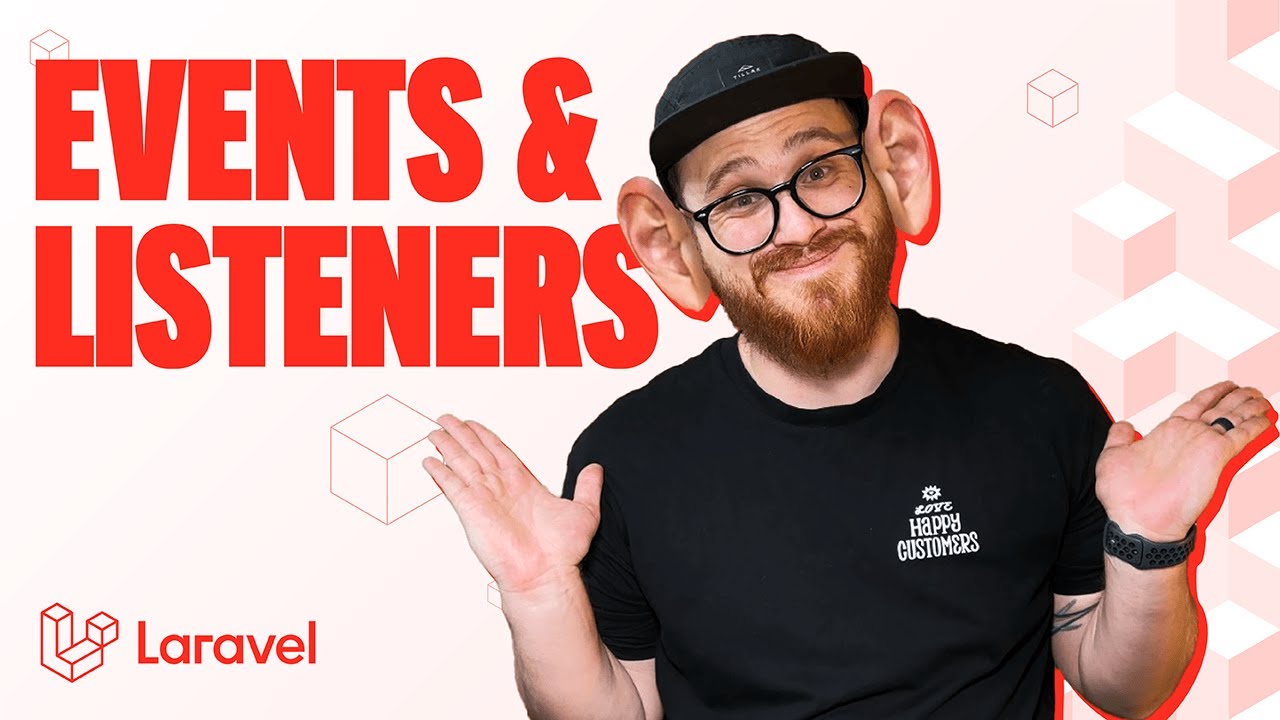
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Laravel Events and Listeners
In the world of web development, maintaining clean and organized code is crucial for the long-term success of any project. As applications grow in complexity, it's common for developers to face challenges in managing various interconnected processes. Laravel, a popular PHP framework, offers a powerful solution to this problem through its events and listeners system.
This article will delve deep into the concept of Laravel events and listeners, exploring how they can help streamline your code logic and improve the overall structure of your application. We'll cover everything from the basics to advanced implementation techniques, providing you with the knowledge to leverage this powerful feature effectively.
Understanding the Problem: The Telephone Game Analogy
Before we dive into the technical aspects of Laravel events and listeners, let's consider a familiar childhood game that illustrates the problem we're trying to solve.
Remember the game of telephone? It starts with one person whispering a phrase to another, who then passes it on to the next person, and so on. By the time the message reaches the last person, it's often completely different from the original phrase. This game demonstrates how information can become distorted or misinterpreted as it passes through multiple intermediaries.
In software development, we face a similar challenge. As our applications grow, we often find ourselves adding more and more logic to a single action or job. What starts as a simple process can quickly become a tangled mess of interconnected tasks, each potentially affecting the others. This is where Laravel events and listeners come to the rescue.
The Problem in Code: A Growing Ticket Purchase Process
Let's examine a practical example to illustrate this problem in the context of a Laravel application. Imagine we have a ticket purchasing system with the following initial logic:
class ProcessTicketPurchaseJob implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
public function handle()
{
// Process the ticket purchase
// Save to database
// Update inventory
// Notify the user
$user->notify(new TicketPurchasedNotification($ticketType));
// Notify admin
$admin->notify(new AdminTicketPurchaseNotification($order));
// Update analytics
$this->updateAnalytics($order);
// Additional notifications and processes...
}
}
As you can see, what started as a simple ticket purchase process has grown to include multiple notifications and additional tasks. This approach, while functional, has several drawbacks:
- Lack of Separation of Concerns: The job is responsible for too many different tasks, violating the single responsibility principle.
- Reduced Flexibility: Adding or modifying processes requires changes to the core job logic.
- Increased Complexity: As more features are added, the job becomes harder to understand and maintain.
- Potential for Errors: With so much happening in one place, it's easier for bugs to creep in and harder to isolate issues.
Introducing Laravel Events and Listeners
Laravel events and listeners provide an elegant solution to this problem by allowing us to decouple various aspects of our application logic. Here's how they work:
- Events: These are simple PHP classes that represent something that has happened in your application. They typically don't contain any logic but serve as data containers.
- Listeners: These are classes that respond to specific events. They contain the logic that should be executed when an event occurs.
By using events and listeners, we can break down our monolithic job into smaller, more manageable pieces of code. This approach offers several benefits:
- Improved Code Organization: Each listener can focus on a specific task, making the code easier to understand and maintain.
- Enhanced Flexibility: New functionality can be added by creating new listeners without modifying existing code.
- Better Testability: Individual listeners can be tested in isolation, improving overall code quality.
- Scalability: As your application grows, you can easily add new listeners to handle additional requirements.
Implementing Events and Listeners in Laravel
Let's refactor our ticket purchase process using Laravel events and listeners. We'll start by creating an event for the ticket purchase.
Creating the Event
To create a new event, we can use the Laravel Artisan command:
php artisan make:event TicketPurchased
This command generates a new event class in the app/Events
directory. Let's modify it to include the necessary data:
namespace App\Events;
use App\Models\User;
use Illuminate\Foundation\Events\Dispatchable;
use Illuminate\Queue\SerializesModels;
class TicketPurchased
{
use Dispatchable, SerializesModels;
public function __construct(
public User $user,
public string $ticketType
) {}
}
This event class now holds the user who purchased the ticket and the type of ticket purchased.
Creating Listeners
Next, we'll create listeners to handle various tasks that need to be performed when a ticket is purchased. Let's start with a listener for sending a notification to the user:
php artisan make:listener SendTicketPurchaseNotification --event=TicketPurchased
This command creates a new listener class in the app/Listeners
directory. Let's implement the logic for sending the notification:
namespace App\Listeners;
use App\Events\TicketPurchased;
use App\Notifications\TicketPurchasedNotification;
class SendTicketPurchaseNotification
{
public function handle(TicketPurchased $event): void
{
$event->user->notify(new TicketPurchasedNotification($event->ticketType));
}
}
We can create similar listeners for other tasks, such as notifying the admin or updating analytics:
php artisan make:listener NotifyAdminOfTicketPurchase --event=TicketPurchased
php artisan make:listener UpdateAnalyticsForTicketPurchase --event=TicketPurchased
Refactoring the Job
Now that we have our event and listeners set up, we can refactor our original job to use the new event:
class ProcessTicketPurchaseJob implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
public function handle()
{
// Process the ticket purchase
// Save to database
// Update inventory
// Dispatch the event
event(new TicketPurchased($user, $ticketType));
}
}
As you can see, our job is now much simpler and focused solely on processing the ticket purchase and dispatching the event. All other tasks have been moved to separate listeners.
Advanced Event and Listener Techniques
Now that we've covered the basics, let's explore some more advanced techniques for working with Laravel events and listeners.
Automatic Event Dispatching with Model Events
Laravel provides a convenient way to automatically dispatch events when certain actions occur on your Eloquent models. This can be particularly useful for events that should always occur when a model is created, updated, or deleted.
Here's how you can set up automatic event dispatching for a Ticket
model:
namespace App\Models;
use App\Events\TicketPurchased;
use Illuminate\Database\Eloquent\Model;
class Ticket extends Model
{
protected $dispatchesEvents = [
'created' => TicketPurchased::class,
];
}
With this setup, the TicketPurchased
event will be automatically dispatched whenever a new Ticket
model is created.
Queueable Listeners
For listeners that perform time-consuming tasks, you can make them queueable. This allows the listener to be pushed onto the queue for background processing, improving the responsiveness of your application.
To make a listener queueable, simply implement the ShouldQueue
interface:
namespace App\Listeners;
use App\Events\TicketPurchased;
use Illuminate\Contracts\Queue\ShouldQueue;
class UpdateAnalyticsForTicketPurchase implements ShouldQueue
{
public function handle(TicketPurchased $event): void
{
// Time-consuming analytics update logic
}
}
Event Subscribers
If you have a class that needs to listen to multiple events, you can use an event subscriber. This allows you to group several event listeners within a single class:
namespace App\Listeners;
class TicketEventSubscriber
{
public function handleTicketPurchase($event) {}
public function handleTicketRefund($event) {}
public function handleTicketTransfer($event) {}
public function subscribe($events)
{
$events->listen(
'App\Events\TicketPurchased',
[TicketEventSubscriber::class, 'handleTicketPurchase']
);
$events->listen(
'App\Events\TicketRefunded',
[TicketEventSubscriber::class, 'handleTicketRefund']
);
$events->listen(
'App\Events\TicketTransferred',
[TicketEventSubscriber::class, 'handleTicketTransfer']
);
}
}
You'll need to register this subscriber in your EventServiceProvider
:
protected $subscribe = [
TicketEventSubscriber::class,
];
Best Practices for Using Events and Listeners
To make the most of Laravel's events and listeners system, consider the following best practices:
-
Keep Events Light: Events should primarily be data containers. Avoid putting complex logic in your event classes.
-
Single Responsibility: Each listener should have a single, well-defined purpose. If a listener is doing too much, consider breaking it into multiple listeners.
-
Use Descriptive Names: Choose clear, descriptive names for your events and listeners that indicate their purpose.
-
Consider Performance: For high-frequency events, be mindful of the number of listeners and their performance impact. Use queued listeners for time-consuming tasks.
-
Leverage Model Events: When appropriate, use model events to automatically dispatch events based on model lifecycles.
-
Document Your Events: Maintain documentation of your events and their listeners, especially in larger projects where multiple developers may be working with the event system.
-
Test Your Listeners: Write unit tests for your listeners to ensure they behave correctly when events are fired.
Conclusion
Laravel events and listeners provide a powerful mechanism for decoupling and organizing your application logic. By breaking down complex processes into discrete, manageable pieces, you can create more maintainable, flexible, and scalable applications.
We've covered the basics of creating and using events and listeners, as well as some advanced techniques like automatic event dispatching, queueable listeners, and event subscribers. By applying these concepts and following best practices, you can significantly improve the structure and maintainability of your Laravel applications.
Remember, the key to effective use of events and listeners is to strike a balance between decoupling your code and maintaining clarity. As with any architectural pattern, it's important to use events and listeners judiciously, applying them where they provide clear benefits to your application's design and maintainability.
As you continue to work with Laravel, experiment with events and listeners in your projects. You'll likely find that they become an invaluable tool in your development toolkit, helping you create cleaner, more organized, and more efficient code.
Further Resources
To deepen your understanding of Laravel events and listeners, consider exploring the following resources:
-
Laravel Official Documentation on Events: The primary source for up-to-date information on working with events in Laravel.
-
Laracasts Video Tutorials: Often feature in-depth explanations and practical examples of Laravel features, including events and listeners.
-
Laravel News: Regularly publishes articles and tutorials that may cover advanced uses of events and listeners.
-
Laravel Podcast: Occasionally discusses architectural patterns and best practices, which may include insights on effective use of events.
-
GitHub Laravel Repository: Examining the Laravel source code can provide insights into how events are implemented and used within the framework itself.
By leveraging these resources and continuously practicing with events and listeners, you'll be well on your way to mastering this powerful feature of Laravel and improving the overall quality of your PHP applications.
Article created from: https://www.youtube.com/watch?v=_8Rrq_RtaB0