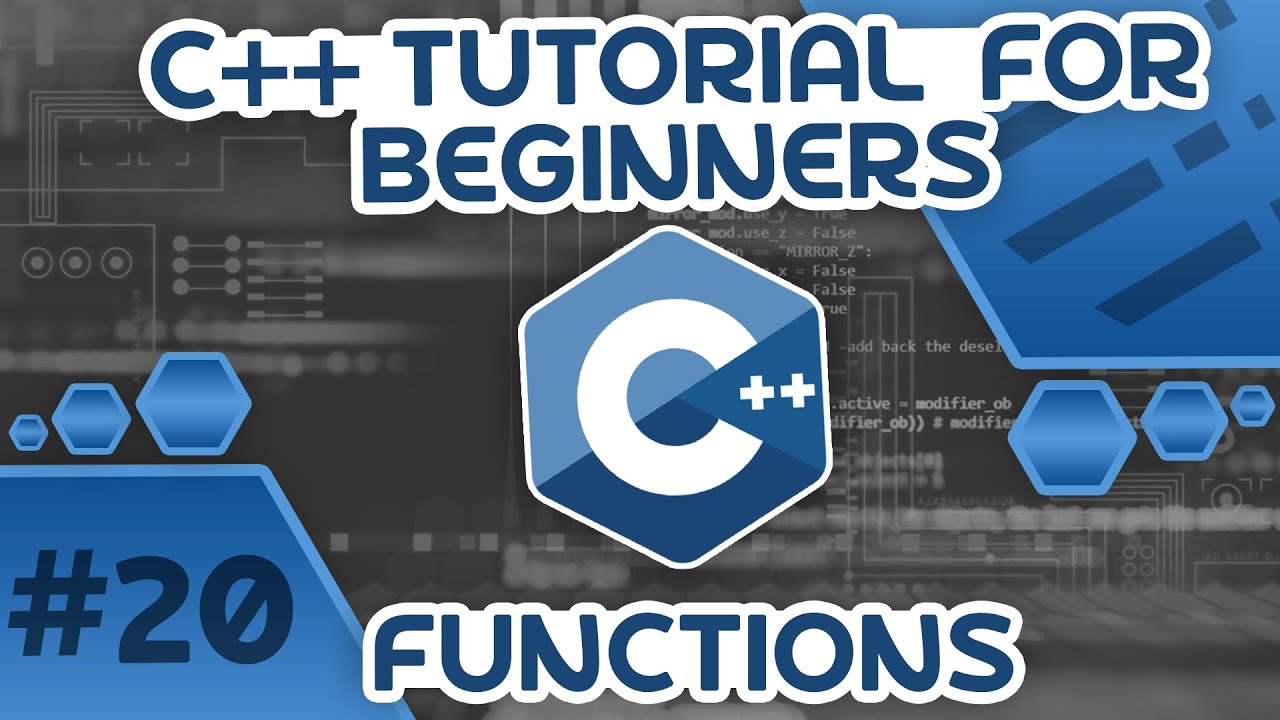
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to C++ Functions
Functions are a fundamental building block in C++ programming. They allow you to create reusable blocks of code that perform specific tasks, making your programs more organized and efficient. In this comprehensive guide, we'll explore the ins and outs of C++ functions, from basic syntax to advanced concepts.
What is a Function?
A function is a self-contained block of code that performs a specific task. It can take input (parameters), process that input, and return a result. Functions serve several purposes in programming:
- Code reusability
- Improved readability
- Easier maintenance
- Better organization of logic
Basic Function Syntax
Here's the basic syntax for defining a function in C++:
return_type function_name(parameter1_type parameter1_name, parameter2_type parameter2_name, ...) {
// Function body
// Code to be executed
return value; // Optional, depending on return_type
}
Let's break down each component:
- return_type: The data type of the value the function will return (e.g., int, double, string). Use 'void' if the function doesn't return anything.
- function_name: A descriptive name for your function.
- parameters: Input values the function will work with (optional).
- function body: The code that performs the desired task.
- return statement: Used to send a value back to the caller (if the function has a non-void return type).
Creating Your First Function
Let's start with a simple example of a function that adds two numbers:
int add(int x, int y) {
return x + y;
}
This function takes two integer parameters, adds them together, and returns the result. To use this function, you would call it like this:
int result = add(2, 3);
std::cout << result; // Outputs: 5
Void Functions
Not all functions need to return a value. Functions that perform an action without returning a result are called void functions. Here's an example:
void printHello() {
std::cout << "Hello, World!";
}
You can call this function simply by writing:
printHello();
Function Prototypes
In C++, you need to declare a function before you can use it. This is typically done at the beginning of your program or in a header file. A function declaration, also known as a function prototype, tells the compiler about the function's name, return type, and parameters. Here's the syntax:
return_type function_name(parameter1_type, parameter2_type, ...);
For example:
int add(int, int); // Function prototype
int main() {
int result = add(2, 3);
std::cout << result;
return 0;
}
int add(int x, int y) {
return x + y;
}
Default Parameters
C++ allows you to specify default values for function parameters. If a caller doesn't provide an argument for a parameter with a default value, the default value is used. Here's an example:
void greet(std::string name = "Guest") {
std::cout << "Hello, " << name << "!";
}
int main() {
greet(); // Outputs: Hello, Guest!
greet("Alice"); // Outputs: Hello, Alice!
return 0;
}
Function Overloading
C++ supports function overloading, which allows you to define multiple functions with the same name but different parameter lists. The compiler determines which function to call based on the arguments provided. For example:
int add(int x, int y) {
return x + y;
}
double add(double x, double y) {
return x + y;
}
int main() {
std::cout << add(2, 3); // Calls int version
std::cout << add(2.5, 3.7); // Calls double version
return 0;
}
Inline Functions
Inline functions are a performance optimization feature in C++. When a function is declared as inline, the compiler may replace the function call with the actual function code, potentially improving performance for small, frequently-called functions. Here's an example:
inline int square(int x) {
return x * x;
}
Recursion
Recursion is a programming technique where a function calls itself to solve a problem. It's particularly useful for tasks that can be broken down into smaller, similar sub-problems. Here's a simple example of a recursive function to calculate factorial:
int factorial(int n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
}
Pass by Value vs. Pass by Reference
In C++, function parameters can be passed by value or by reference. Understanding the difference is crucial for writing efficient and correct code.
Pass by Value
When you pass an argument by value, a copy of the argument is created and passed to the function. Changes made to the parameter inside the function do not affect the original argument. For example:
void incrementByValue(int x) {
x++;
}
int main() {
int num = 5;
incrementByValue(num);
std::cout << num; // Still outputs 5
return 0;
}
Pass by Reference
When you pass an argument by reference, the function receives a reference to the original variable. Changes made to the parameter inside the function affect the original argument. To pass by reference, use the & symbol:
void incrementByReference(int& x) {
x++;
}
int main() {
int num = 5;
incrementByReference(num);
std::cout << num; // Outputs 6
return 0;
}
Const Parameters
You can use the 'const' keyword to prevent a function from modifying a parameter passed by reference. This is useful when you want to avoid accidental modifications:
void printValue(const int& x) {
std::cout << x;
// x++; // This would cause a compiler error
}
Function Pointers
Function pointers allow you to store and pass functions as arguments to other functions. This can be useful for implementing callback mechanisms or creating more flexible code. Here's a basic example:
int add(int x, int y) { return x + y; }
int subtract(int x, int y) { return x - y; }
int operate(int x, int y, int (*operation)(int, int)) {
return operation(x, y);
}
int main() {
std::cout << operate(5, 3, add); // Outputs: 8
std::cout << operate(5, 3, subtract); // Outputs: 2
return 0;
}
Lambda Functions
Lambda functions, introduced in C++11, allow you to create anonymous functions inline. They're particularly useful for short, one-off functions. Here's a simple example:
#include <algorithm>
#include <vector>
int main() {
std::vector<int> numbers = {4, 1, 3, 5, 2};
std::sort(numbers.begin(), numbers.end(), [](int a, int b) { return a > b; });
// numbers is now sorted in descending order
return 0;
}
Best Practices for Writing Functions
- Keep functions small and focused: Each function should do one thing and do it well.
- Use descriptive names: Function names should clearly describe what the function does.
- Limit the number of parameters: If a function needs many parameters, consider grouping them into a struct or class.
- Use const for parameters that shouldn't be modified: This prevents accidental modifications and clarifies intent.
- Return early: If you can determine the result early in the function, return immediately instead of using nested if-else statements.
- Use function overloading judiciously: Don't overload functions with completely different behaviors.
- Consider using default parameters instead of function overloading for simple variations.
- Document your functions: Use comments to explain what the function does, its parameters, and its return value.
Conclusion
Functions are a powerful feature in C++ that allow you to write modular, reusable, and organized code. By mastering functions, you'll be able to create more efficient and maintainable programs. Remember to practice writing different types of functions and experiment with the various features C++ offers. As you gain experience, you'll develop a better intuition for when and how to use functions effectively in your projects.
Keep exploring and practicing, and you'll soon find yourself writing more elegant and efficient C++ code!
Article created from: https://www.youtube.com/watch?v=C83tPpvxIJA&embeds_referring_euri=https%3A%2F%2Fpalomar.instructure.com%2F