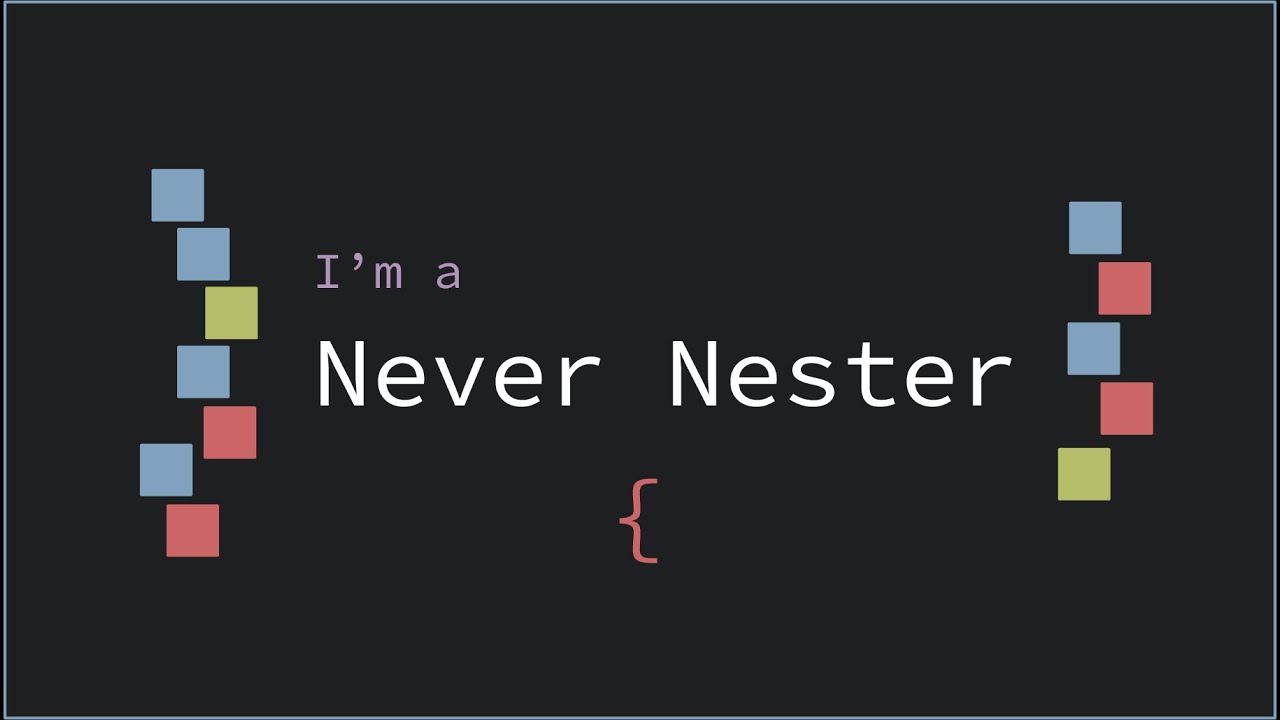
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeThe Never Nester Approach to Coding
In the world of programming, there's a group of developers known as "Never Nesters." These coders have an aversion to deeply nested code structures, preferring to keep their functions flat and easily readable. While this might seem like a niche preference, it's more common than you might think. Even influential figures in the programming world, such as Linus Torvalds, are suspected to follow this approach.
What is Nesting in Code?
Nesting in code refers to the practice of adding inner blocks within a function. Each open brace typically represents an additional level of depth. For example:
- A function with no inner blocks is considered one level deep.
- Adding an if statement increases the depth to two levels.
- Introducing a loop within that if statement takes it to three levels deep.
For a Never Nester, three levels of depth is generally the maximum they're willing to tolerate. Anything beyond this can quickly become difficult to read and understand.
The Problem with Deep Nesting
When code becomes too deeply nested, it can significantly impact readability and maintainability. A function that was once easy to follow can quickly become a complex maze of conditions and loops. This increased complexity forces developers to hold multiple conditions in their minds simultaneously, making the code harder to understand and more prone to errors.
Techniques for Reducing Nesting
Fortunately, there are effective methods to reduce nesting and improve code clarity. Two primary techniques are extraction and inversion.
Extraction
Extraction involves taking a portion of a function and moving it into its own separate function. This technique helps break down complex logic into smaller, more manageable pieces.
Inversion
Inversion is the process of flipping conditions and using early returns. Instead of nesting the main logic deep within conditional blocks, you check for invalid conditions first and return early if they're met. This approach keeps the main logic at a shallower nesting level.
Applying Extraction and Inversion: A Practical Example
Let's examine a real-world scenario where we can apply these techniques to improve code readability. Consider a function designed to manage multiple file downloads:
def run(self):
while True:
# Process incoming requests from the queue
while not self.queue.empty():
url = self.queue.get()
self.current_downloads.append({
'url': url,
'state': 'pending'
})
# Process current downloads
for download in self.current_downloads:
if download['state'] == 'pending':
# Start new download
download['state'] = 'in_progress'
download['download'] = self.downloader.start(download['url'])
elif download['state'] == 'in_progress':
# Check download progress
result = download['download'].process()
if result.status == 'complete':
download['state'] = 'complete'
elif result.status == 'in_progress':
pass
elif result.status == 'error':
if result.error_type == 'http':
if result.is_retryable and download.get('retries', 0) < 3:
download['retries'] = download.get('retries', 0) + 1
download['state'] = 'pending'
else:
self.failed_downloads.put(download)
self.current_downloads.remove(download)
elif result.error_type == 'connection':
if download.get('retries', 0) < 3:
download['retries'] = download.get('retries', 0) + 1
download['state'] = 'pending'
else:
self.connection_disabled = True
break
# Clear completed downloads
self.current_downloads = [d for d in self.current_downloads if d['state'] != 'complete']
# Wait for new downloads if queue is empty
if self.queue.empty() and not self.current_downloads:
self.new_download_event.wait()
self.new_download_event.clear()
# Check if connection is disabled
if self.connection_disabled:
self.current_downloads.clear()
self.connection_disabled = False
This function is deeply nested and handles multiple responsibilities, making it difficult to read and maintain. Let's apply extraction and inversion to improve its structure.
Step 1: Extract Major Sections
First, we'll extract the main sections of the function into separate methods:
def run(self):
while True:
self.process_queue()
self.process_downloads()
self.clear_completed_downloads()
self.wait_for_new_downloads()
self.handle_disabled_connection()
def process_queue(self):
while not self.queue.empty():
url = self.queue.get()
self.current_downloads.append({
'url': url,
'state': 'pending'
})
def process_downloads(self):
for download in self.current_downloads:
if download['state'] == 'pending':
self.process_pending_download(download)
elif download['state'] == 'in_progress':
self.process_in_progress_download(download)
def clear_completed_downloads(self):
self.current_downloads = [d for d in self.current_downloads if d['state'] != 'complete']
def wait_for_new_downloads(self):
if self.queue.empty() and not self.current_downloads:
self.new_download_event.wait()
self.new_download_event.clear()
def handle_disabled_connection(self):
if self.connection_disabled:
self.current_downloads.clear()
self.connection_disabled = False
Step 2: Further Extraction and Inversion
Now, let's focus on the process_pending_download
and process_in_progress_download
methods:
def process_pending_download(self, download):
download['state'] = 'in_progress'
download['download'] = self.downloader.start(download['url'])
def process_in_progress_download(self, download):
result = download['download'].process()
if result.status == 'complete':
download['state'] = 'complete'
elif result.status == 'error':
self.handle_download_error(download, result)
def handle_download_error(self, download, result):
if result.error_type == 'http':
self.handle_http_error(download, result)
elif result.error_type == 'connection':
self.handle_connection_error(download)
def handle_http_error(self, download, result):
if result.is_retryable and download.get('retries', 0) < 3:
self.retry_download(download)
else:
self.fail_download(download)
def handle_connection_error(self, download):
if download.get('retries', 0) < 3:
self.retry_download(download)
else:
self.connection_disabled = True
def retry_download(self, download):
download['retries'] = download.get('retries', 0) + 1
download['state'] = 'pending'
def fail_download(self, download):
self.failed_downloads.put(download)
self.current_downloads.remove(download)
Benefits of Reduced Nesting
By applying these techniques, we've transformed a deeply nested, complex function into a series of smaller, more focused functions. This refactored code offers several advantages:
-
Improved Readability: Each function now has a clear, single responsibility, making it easier to understand at a glance.
-
Better Maintainability: Smaller functions are easier to modify and debug.
-
Enhanced Testability: With logic broken down into smaller units, it becomes easier to write unit tests for each piece of functionality.
-
Reduced Cognitive Load: Developers no longer need to hold multiple conditions in their minds simultaneously while reading the code.
-
Easier Collaboration: Team members can more easily understand and contribute to specific parts of the codebase.
The Linux Kernel Perspective
Interestingly, the Linux kernel style guidelines align with the Never Nester philosophy. They state, "If you need more than three levels of indentation, you're screwed anyway and should fix your program." While this might seem extreme, it underscores the importance of keeping code simple and readable.
The Linux kernel developers even enforce this visually by setting the tab size to 8 characters wide. This makes deeply nested code visually unappealing and encourages developers to refactor and simplify their code.
Conclusion: The Value of Limiting Nesting
While you don't need to go to the extreme of setting 8-character wide tabs, there's significant value in limiting the depth of nesting in your code. By constraining how much you nest, you're forced to write better, more modular code.
The process of reducing nesting often leads to creating small, concise functions with single responsibilities. This approach aligns well with the Single Responsibility Principle from SOLID design principles, promoting more maintainable and extensible code.
Remember, the goal isn't just to reduce nesting for the sake of it, but to improve the overall quality and clarity of your code. By applying techniques like extraction and inversion, you can create code that's easier to read, understand, and maintain.
As you develop your coding style, consider adopting some Never Nester principles. You might find that it not only improves your code but also enhances your problem-solving skills as a developer. After all, writing clean, readable code is as much an art as it is a science.
Practical Tips for Reducing Nesting
-
Use Guard Clauses: Check for invalid conditions early in your functions and return or throw exceptions immediately. This can help reduce the overall nesting level.
-
Break Down Complex Functions: If a function is doing too much, consider breaking it into smaller, more focused functions.
-
Utilize Early Returns: Don't be afraid to return early from a function if certain conditions are met. This can help flatten your code.
-
Leverage Polymorphism: Instead of using deep if-else structures, consider using polymorphism to handle different cases.
-
Use Descriptive Function Names: When extracting code into new functions, use clear, descriptive names that explain what the function does. This improves readability without requiring deep nesting.
-
Consider Using a Linter: Many linters can be configured to warn you when your code becomes too deeply nested.
-
Refactor Regularly: Don't wait for your code to become a nested mess. Refactor regularly to keep your codebase clean and manageable.
-
Use Comments Wisely: If you find yourself needing to explain a deeply nested structure, it might be a sign that you need to refactor.
-
Embrace Functional Programming Concepts: Techniques from functional programming, such as map, filter, and reduce, can often replace nested loops and conditionals.
-
Review and Refactor: Regularly review your code and look for opportunities to reduce nesting. Often, a fresh perspective can reveal new ways to simplify your code.
By incorporating these practices into your coding routine, you'll find yourself naturally writing cleaner, more maintainable code with reduced nesting. Remember, the goal is not just to follow rules blindly, but to create code that is easier to understand, debug, and extend over time.
As you gain more experience with these techniques, you'll develop an intuition for when and how to apply them effectively. You may even find that your overall approach to problem-solving in code evolves, leading you to design more elegant solutions from the start.
Ultimately, reducing nested code is about more than just aesthetics or following a particular coding style. It's about creating code that is robust, flexible, and stands the test of time. By mastering these techniques, you're not just improving your code - you're elevating your craft as a developer.
The Bigger Picture: Code Quality and Software Architecture
While we've focused primarily on reducing nesting at the function level, it's important to understand how this practice fits into the broader context of code quality and software architecture.
Impact on Code Quality
Reducing nesting is just one aspect of improving overall code quality. Other important factors include:
- Consistency: Maintaining a consistent style throughout your codebase.
- Modularity: Designing your code in modular, reusable components.
- Documentation: Providing clear, concise documentation for your code.
- Testing: Implementing comprehensive unit and integration tests.
- Performance: Optimizing your code for efficiency and speed.
By addressing nesting issues, you're contributing to the overall quality of your codebase, making it easier for you and your team to maintain and extend the software over time.
Influence on Software Architecture
The principles we've discussed for reducing nesting can also be applied at a higher level to improve your overall software architecture:
-
Separation of Concerns: Just as we separate nested code into distinct functions, we can separate different aspects of our application into distinct modules or services.
-
Layered Architecture: The idea of keeping the main logic at a shallow nesting level can be extended to keeping core business logic separate from infrastructure concerns.
-
Dependency Inversion: By extracting deeply nested code into separate functions, we're often applying the Dependency Inversion Principle, which can lead to more flexible and testable architectures.
-
Microservices: In some cases, the process of breaking down a complex, nested function might reveal opportunities for separating functionality into distinct services.
By applying these principles consistently, you can create software architectures that are more scalable, maintainable, and adaptable to change.
Continuous Improvement and Learning
Becoming a skilled developer who writes clean, maintainable code is an ongoing journey. Here are some ways to continue improving your skills:
-
Code Reviews: Participate in code reviews, both as a reviewer and a reviewee. This exposes you to different coding styles and perspectives.
-
Open Source Contributions: Contributing to open source projects can expose you to high-quality codebases and best practices.
-
Reading: Regularly read books, articles, and blogs about software development. Some classic books like "Clean Code" by Robert C. Martin can provide valuable insights.
-
Practice: Regularly challenge yourself with coding exercises or personal projects where you can apply these principles.
-
Learn from Others: Engage with the developer community through forums, meetups, or conferences to learn from others' experiences.
-
Teach Others: Teaching or mentoring others can deepen your own understanding of these concepts.
Remember, the goal is not perfection, but continuous improvement. Every time you refactor a nested piece of code or design a clean, flat function from the start, you're honing your skills and contributing to a better codebase.
By embracing the principles of reducing nesting and writing clean code, you're not just improving individual functions or files - you're contributing to a culture of quality in software development. This mindset can lead to more robust, maintainable software systems and a more satisfying and productive development experience for you and your team.
So the next time you find yourself facing a deeply nested function or a complex piece of logic, remember the techniques we've discussed. Take a step back, consider how you can extract, invert, or otherwise simplify the code, and enjoy the process of crafting cleaner, more elegant solutions. Your future self (and your colleagues) will thank you for it.
Article created from: https://youtu.be/CFRhGnuXG-4?si=T0TERg7QCWvYd2L9