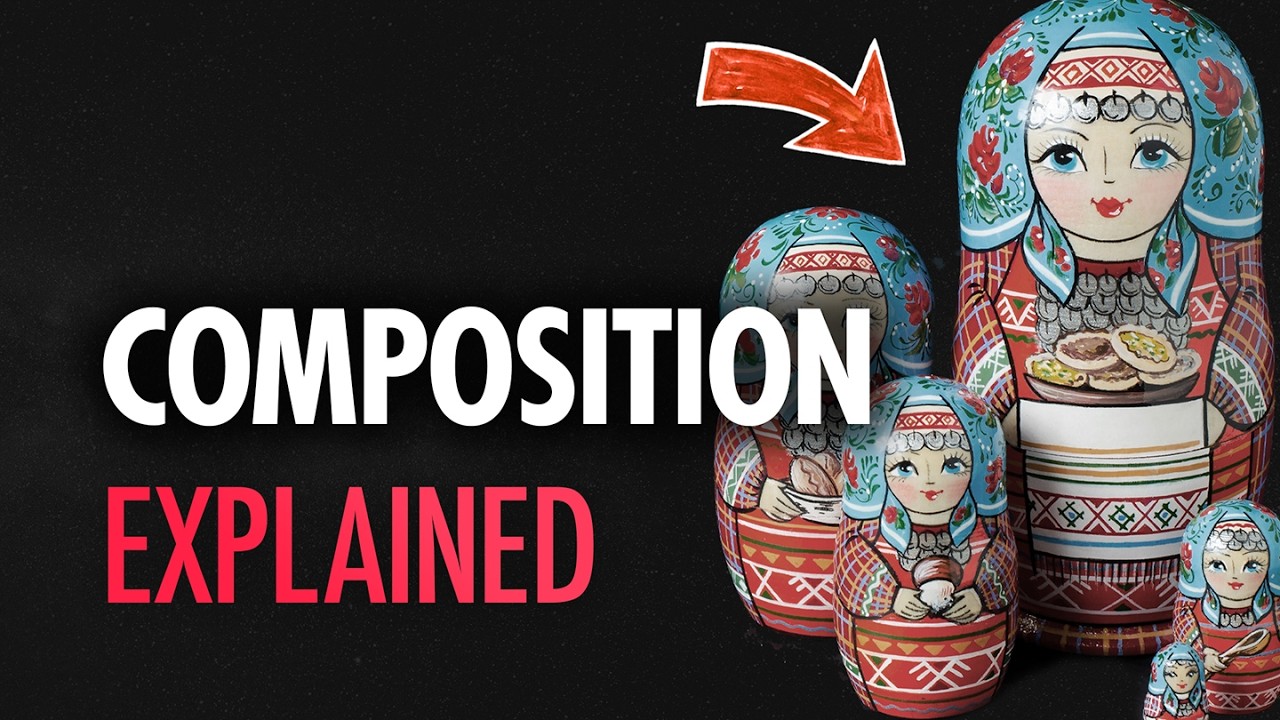
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeThe Debate: Composition vs Inheritance
In the world of software development, there's an ongoing debate about the best approach to building flexible and maintainable code structures. Many experienced developers advocate for favoring composition over inheritance. But what does this really mean, and why is it so important?
Understanding Inheritance
Inheritance is a fundamental concept in object-oriented programming (OOP). It allows a class to inherit properties and methods from another class, creating a parent-child relationship. This approach can seem intuitive at first, as it mirrors real-world hierarchies and relationships.
For example, if we're building a system to manage different types of vehicles, we might start with a base "Vehicle" class and then create subclasses like "Car," "Truck," and "Motorcycle" that inherit from it. This allows us to reuse common code and create a logical structure for our objects.
The Pitfalls of Inheritance
However, as our software grows more complex, inheritance can lead to several problems:
- Tight coupling: Subclasses are tightly bound to their parent classes, making it difficult to change one without affecting the other.
- Inflexibility: As requirements change, the rigid hierarchy created by inheritance can become a constraint rather than a benefit.
- Complicated nested hierarchies: Large inheritance trees can become difficult to understand and maintain.
- The "diamond problem": In languages that support multiple inheritance, conflicts can arise when a class inherits from two classes that have a common ancestor.
Enter Composition
Composition offers an alternative approach. Instead of building objects through inheritance, we create them by combining smaller, reusable components. This is often described as "has-a" relationships rather than "is-a" relationships.
Using our vehicle example, instead of inheriting from a "Vehicle" class, we might create a "Truck" object by composing it from separate "Engine," "Wheels," and "Cargo" components. This approach offers several advantages:
- Flexibility: It's easier to add or remove components as needed without affecting the entire class hierarchy.
- Loose coupling: Components can be developed and tested independently, leading to more modular code.
- Easier maintenance: Changes to one component are less likely to have unintended consequences on other parts of the system.
- Better reusability: Components can be shared across different types of objects more easily.
Practical Examples: Kotlin vs Go
Let's explore these concepts further by looking at practical code examples in two different languages: Kotlin and Go. This comparison will help illustrate the differences between inheritance-based and composition-based approaches.
Kotlin: The Inheritance Approach
Kotlin, like Java, is known for its strong support of OOP principles, including inheritance. Let's consider a scenario where we need to create various shapes for a drawing application.
// Base class
open class Shape(val x: Int, val y: Int) {
open fun draw() {
println("Drawing a shape at ($x, $y)")
}
open fun area(): Double {
return 0.0
}
}
// Subclasses
class Rectangle(x: Int, y: Int, val width: Int, val height: Int) : Shape(x, y) {
override fun draw() {
println("Drawing a rectangle at ($x, $y) with width $width and height $height")
}
override fun area(): Double {
return width * height.toDouble()
}
}
class Circle(x: Int, y: Int, val radius: Int) : Shape(x, y) {
override fun draw() {
println("Drawing a circle at ($x, $y) with radius $radius")
}
override fun area(): Double {
return Math.PI * radius * radius
}
}
At first glance, this structure seems logical and clean. We have a base Shape
class that defines common properties and methods, and specific shapes inherit from it, overriding methods as needed.
However, let's consider what happens when we need to add more shapes or functionality:
class Line(x1: Int, y1: Int, x2: Int, y2: Int) : Shape(x1, y1) {
override fun draw() {
println("Drawing a line from ($x, $y) to ($x2, $y2)")
}
// The area() method doesn't make sense for a line
override fun area(): Double {
throw UnsupportedOperationException("Lines don't have an area")
}
}
// New requirement: some shapes should be clickable
interface Clickable {
fun onClick()
}
class ClickableRectangle(x: Int, y: Int, width: Int, height: Int) : Rectangle(x, y, width, height), Clickable {
override fun onClick() {
println("Rectangle clicked!")
}
}
Now we're starting to see some issues:
- The
Line
class is forced to inherit thearea()
method, which doesn't make sense for a line. - We've had to create a new
ClickableRectangle
class to add click functionality. What if we need clickable circles too? We'd end up with a complex hierarchy of classes. - What if we need to add more properties or behaviors that only apply to some shapes? The inheritance structure becomes increasingly difficult to manage.
Go: The Composition Approach
Now let's look at how we might approach the same problem using Go, which encourages composition over inheritance.
package main
import (
"fmt"
"math"
)
// Define interfaces for behaviors
type Drawable interface {
Draw()
}
type AreaCalculable interface {
Area() float64
}
type Clickable interface {
OnClick()
}
// Define structs for different shapes
type Point struct {
X, Y int
}
type Rectangle struct {
Point
Width, Height int
}
type Circle struct {
Point
Radius int
}
type Line struct {
Start, End Point
}
// Implement methods for each shape
func (r Rectangle) Draw() {
fmt.Printf("Drawing a rectangle at (%d, %d) with width %d and height %d\n", r.X, r.Y, r.Width, r.Height)
}
func (r Rectangle) Area() float64 {
return float64(r.Width * r.Height)
}
func (c Circle) Draw() {
fmt.Printf("Drawing a circle at (%d, %d) with radius %d\n", c.X, c.Y, c.Radius)
}
func (c Circle) Area() float64 {
return math.Pi * float64(c.Radius*c.Radius)
}
func (l Line) Draw() {
fmt.Printf("Drawing a line from (%d, %d) to (%d, %d)\n", l.Start.X, l.Start.Y, l.End.X, l.End.Y)
}
// Add click behavior
func (r Rectangle) OnClick() {
fmt.Println("Rectangle clicked!")
}
func (c Circle) OnClick() {
fmt.Println("Circle clicked!")
}
// Create a function that can draw any Drawable
func drawShape(d Drawable) {
d.Draw()
}
// Create a function that can calculate area of any AreaCalculable
func calculateArea(a AreaCalculable) float64 {
return a.Area()
}
// Create a function that can handle clicks on any Clickable
func handleClick(c Clickable) {
c.OnClick()
}
func main() {
rect := Rectangle{Point{10, 20}, 30, 40}
circ := Circle{Point{100, 100}, 50}
line := Line{Point{0, 0}, Point{100, 100}}
drawShape(rect)
drawShape(circ)
drawShape(line)
fmt.Printf("Rectangle area: %.2f\n", calculateArea(rect))
fmt.Printf("Circle area: %.2f\n", calculateArea(circ))
handleClick(rect)
handleClick(circ)
}
In this Go example, we've used composition and interfaces to create a more flexible structure:
- We define interfaces for different behaviors (
Drawable
,AreaCalculable
,Clickable
). - We create separate structs for each shape, composing them from smaller parts (like
Point
). - We implement methods for each shape to satisfy the interfaces.
- We can easily add or remove behaviors for each shape without affecting others.
- We use interface parameters in functions to work with any shape that implements the required behavior.
This approach offers several advantages:
- Flexibility: We can easily add new shapes or behaviors without modifying existing code.
- Modularity: Each shape and behavior is independent, making the code easier to understand and maintain.
- Reusability: We can mix and match behaviors as needed for different shapes.
- Testability: It's easier to write unit tests for smaller, focused components.
The Benefits of Composition
As we've seen from the Go example, composition offers several key benefits over inheritance:
1. Increased Flexibility
Composition allows us to build complex objects from simpler, reusable parts. This makes it easier to adapt our code to changing requirements. If we need a new type of shape or a new behavior, we can simply create a new struct or interface without disturbing existing code.
2. Better Encapsulation
With composition, we can hide the internal details of our components. This leads to cleaner interfaces and reduces the risk of breaking changes affecting other parts of the system.
3. Improved Testability
Smaller, more focused components are easier to test in isolation. This can lead to more comprehensive and maintainable test suites.
4. Easier Refactoring
Because components are loosely coupled, it's often easier to refactor code that uses composition. We can change the internals of a component without affecting the rest of the system, as long as we maintain the same interface.
5. Avoidance of the "Fragile Base Class" Problem
In inheritance hierarchies, changes to a base class can have unexpected effects on subclasses. This is known as the "fragile base class" problem. Composition helps avoid this by keeping components separate and independent.
When to Use Inheritance
While composition offers many advantages, it's important to note that inheritance isn't always bad. There are scenarios where inheritance can be the right choice:
-
When there's a clear "is-a" relationship: If you have a genuine subtype relationship where the subclass is truly a more specific version of the superclass, inheritance can be appropriate.
-
For framework design: Many frameworks use inheritance as a way to allow users to plug into and extend functionality.
-
When dealing with immutable types: In some cases, especially with immutable types, inheritance can lead to cleaner, more intuitive code.
-
For simple, stable hierarchies: If you have a small, well-defined hierarchy that's unlikely to change, inheritance might be simpler and more straightforward.
However, even in these cases, it's worth considering whether composition could offer a more flexible solution.
Best Practices for Using Composition
To make the most of composition in your code:
-
Design for interfaces, not implementations: Focus on defining clear interfaces for your components. This allows for easier substitution and extension.
-
Keep components small and focused: Each component should have a single, well-defined responsibility.
-
Use dependency injection: Instead of hard-coding dependencies, pass them in as parameters. This makes your code more flexible and easier to test.
-
Favor composition over inheritance by default: Start with composition, and only use inheritance when it clearly adds value and simplifies your code.
-
Use the Strategy pattern: This pattern uses composition to define a family of algorithms, encapsulate each one, and make them interchangeable.
-
Leverage mixins or traits: Some languages offer features like mixins (Ruby) or traits (Scala, PHP) that provide a form of multiple inheritance through composition.
Conclusion
The debate between composition and inheritance is not about declaring one approach universally superior. Rather, it's about understanding the strengths and weaknesses of each approach and choosing the right tool for the job.
Composition offers a more flexible, modular approach to building software systems. It allows us to create complex objects and behaviors by combining simpler, reusable components. This can lead to code that's easier to understand, maintain, and extend.
Inheritance, while powerful, can lead to rigid hierarchies and unexpected dependencies between classes. It's best used judiciously, in situations where there's a clear and stable "is-a" relationship between classes.
By favoring composition over inheritance, we can create more adaptable, maintainable software architectures. This approach aligns well with modern software development practices, emphasizing modularity, testability, and flexibility.
As you design and implement your next software project, consider how you can leverage the power of composition to create cleaner, more flexible code structures. Remember, the goal is not to avoid inheritance entirely, but to use it thoughtfully and sparingly, turning to composition as your default approach for building complex systems.
By mastering these concepts and applying them judiciously, you'll be well-equipped to create software that can evolve and adapt to changing requirements, setting a strong foundation for long-term maintainability and scalability.
Article created from: https://youtu.be/_hb_oRhASuw