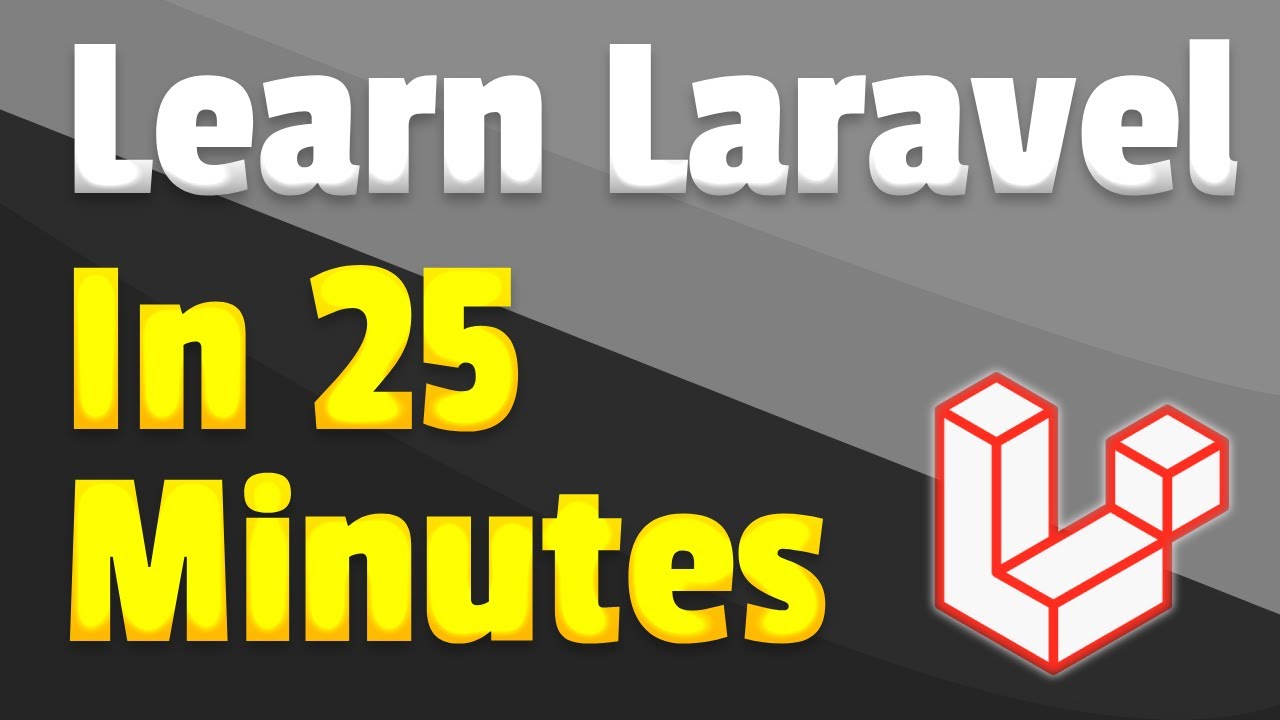
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeGetting Started with Laravel for Beginners
Laravel is a powerful PHP framework designed for the development of web applications. In this guide, we'll walk you through creating a basic CRUD (Create, Read, Update, Delete) application - a simple to-do list - using Laravel. By the end of this article, you'll have a foundational understanding of how to use Laravel to build web applications.
Creating a New Laravel Project
The journey begins by setting up a new Laravel project. Execute the following command in your terminal to create a project named to-do-list
:
laravel new to-do-list
After creating your project, navigate to the project directory and run the Laravel development server with:
php artisan serve
This command will start your application, making it accessible at localhost:8000
.
Crafting Your First View
The first thing to do is customize the default welcome view to serve as your application's interface. In Laravel, views are stored in the resources/views
directory with the .blade.php
extension, which is Laravel's templating engine. For our to-do list, we'll modify the welcome.blade.php
file, stripping it down to the essentials and adding a simple form with an input and a button to add new to-do items.
Setting Up the Database
Before we can store our to-do items, we need a database. After creating a database (e.g., using TablePlus), we need to connect it to our Laravel application by setting the appropriate environment variables in the .env
file. These include DB_DATABASE
, DB_USERNAME
, and DB_PASSWORD
.
Creating Models and Migrations
With our database ready, it's time to create a model and migration for our to-do list items. Models in Laravel interact with the database, while migrations are used to define the database's structure. Run the following Artisan command to create a model with an accompanying migration:
php artisan make:model ListItem -m
Edit the migration file to include the necessary columns for your to-do items, such as name
and is_complete
, then run the migrations with php artisan migrate
to create the table in your database.
Basic Routing and Form Handling
Routing in Laravel determines how HTTP requests are handled. By default, routes are defined in the routes/web.php
file. For our to-do list, we'll add a route to handle the form submission when adding new items. We'll also need to ensure that any form in Laravel includes a CSRF token for security purposes.
Controllers and Business Logic
To keep our code organized, we create controllers to handle the business logic of our application. Use the Artisan command php artisan make:controller ToDoListController
to create a new controller. Inside this controller, we can define methods for adding to-do items and marking them as complete, interacting with the model to perform database operations.
Displaying and Updating the To-Do List
The final step is to update the view to display the to-do items fetched from the database and provide a way to mark them as complete. We'll utilize Laravel's Blade syntax to loop through the list items and render them on the page. Each item will have a button to mark it as complete, which, when clicked, will update the item's is_complete
status in the database.
Wrapping Up
Once all the functionality is in place, you'll have a basic but functional to-do list application built with Laravel. Through this process, you've learned how to work with views, models, migrations, routing, and database manipulation in Laravel.
For a more detailed walkthrough and code examples, you can watch the full tutorial on YouTube: Laravel Basics Tutorial.
As you continue to learn and build with Laravel, remember that there's much more to explore, such as middleware, authentication, and more advanced features that make Laravel a robust solution for web application development.