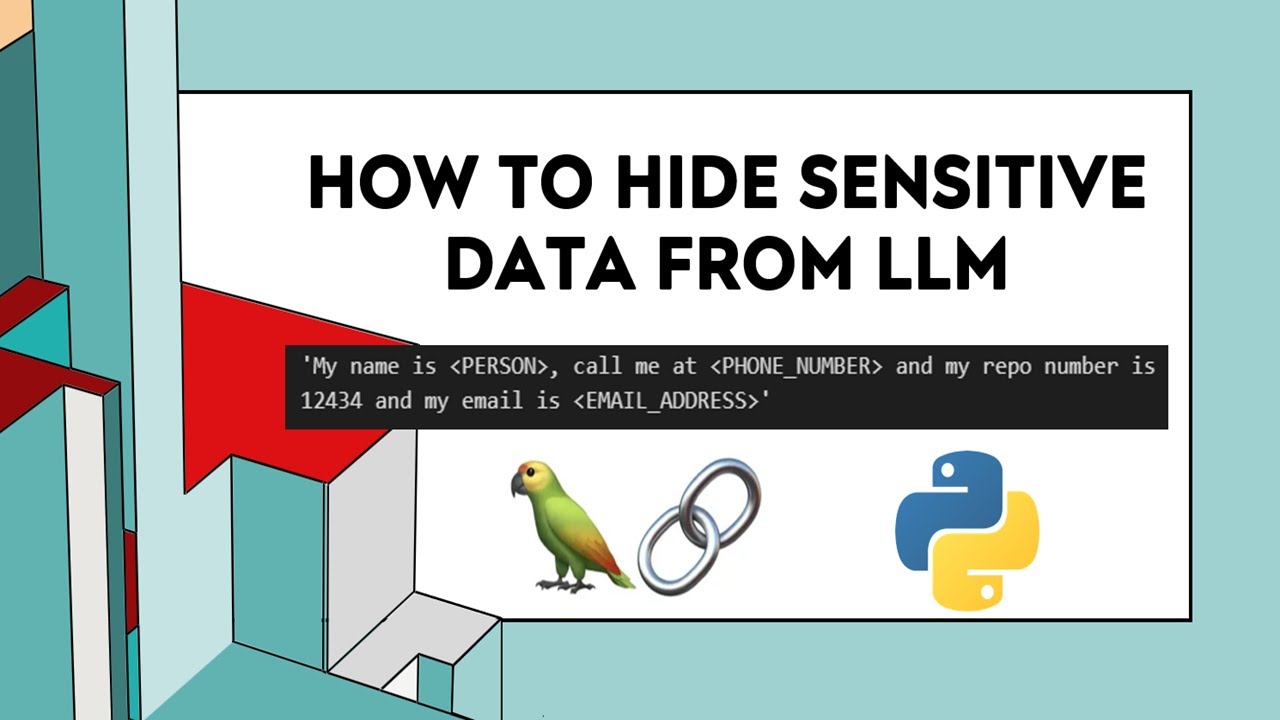
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction
In today's data-driven world, protecting sensitive information is crucial. Whether you're working with customer data, financial records, or any other confidential information, it's essential to have robust methods for masking or anonymizing sensitive data before processing or sharing it. This article will guide you through using Python, Langchain, and Microsoft's Presidio library to effectively hide sensitive information in your data.
Why Mask Sensitive Data?
Before we dive into the technical details, let's briefly discuss why masking sensitive data is important:
- Privacy Protection: Safeguarding personal information is a legal and ethical obligation.
- Regulatory Compliance: Many industries have strict regulations about handling sensitive data (e.g., GDPR, HIPAA).
- Risk Mitigation: Reducing the exposure of sensitive data minimizes the potential impact of data breaches.
- Data Sharing: Anonymized data can be shared more freely for analysis or processing purposes.
Getting Started
To begin, you'll need to install the necessary Python packages. Make sure you have the following installed:
- langchain
- presidio-anonymizer
- faker
You can install these packages using pip:
pip install langchain presidio-anonymizer faker
Basic Anonymization with Presidio
Let's start with a basic example of how to use Presidio's anonymizer to mask sensitive information.
from presidio_anonymizer import AnonymizerEngine
# Initialize the anonymizer
anonymizer = AnonymizerEngine()
# Sample text with sensitive information
text = "My name is Danny Gosh. My phone number is 324-567-8901 and my email is [email protected]"
# Anonymize the text
anonymized_text = anonymizer.anonymize(text=text)
print(anonymized_text.text)
This code will output something like:
My name is Heather. My phone number is 123-456-7890 and my email is [email protected]
As you can see, Presidio has automatically detected and replaced the name, phone number, and email address with anonymized versions.
Selective Anonymization
Sometimes, you might want to anonymize only specific types of information. Presidio allows you to do this by specifying the fields you want to anonymize.
# Anonymize only the person's name
anonymized_text = anonymizer.anonymize(text=text, analyze_fields=["PERSON"])
print(anonymized_text.text)
This will output something like:
My name is Jacquelyn Smith. My phone number is 324-567-8901 and my email is [email protected]
Notice that only the name has been changed, while the phone number and email remain the same.
Custom Patterns and Anonymization
Presidio is quite powerful, but it might not recognize all types of sensitive information out of the box. For instance, let's say you have a custom identifier like a "repo number" that needs to be anonymized.
text = "My name is Danny Gosh. Call me at 324-567-8901. My repo number is 12345 and my email is [email protected]"
anonymized_text = anonymizer.anonymize(text=text)
print(anonymized_text.text)
In this case, Presidio will anonymize the name, phone number, and email, but it won't recognize the "repo number" as sensitive information. To handle this, we need to create a custom pattern recognizer.
from presidio_anonymizer.entities import RecognizerResult, OperatorConfig
from presidio_anonymizer import AnonymizerEngine, RecognizerRegistry
from presidio_anonymizer.recognizers import PatternRecognizer
import re
# Define a pattern for the repo number
repo_pattern = r'\b\d{5}\b'
# Create a custom recognizer
repo_recognizer = PatternRecognizer(
supported_entity="REPO_NUMBER",
patterns=[{"name": "repo_number", "regex": repo_pattern}]
)
# Initialize the recognizer registry and add the custom recognizer
registry = RecognizerRegistry()
registry.add_recognizer(repo_recognizer)
# Initialize the anonymizer with the custom registry
anonymizer = AnonymizerEngine(registry=registry)
# Anonymize the text
anonymized_text = anonymizer.anonymize(text=text)
print(anonymized_text.text)
Now, the repo number will also be anonymized along with the other sensitive information.
Realistic Anonymization with Faker
While Presidio does a good job of anonymizing data, sometimes the replacements can look unrealistic (e.g., replacing a number with "REPO_NUMBER"). To make the anonymized data look more natural, we can use the Faker library to generate realistic fake data.
from faker import Faker
fake = Faker()
# Custom operator to generate fake repo numbers
class FakeRepoOperator(OperatorConfig):
def operate(self, text: str) -> str:
return str(fake.random_int(min=10000, max=99999))
# Add the custom operator to the anonymizer
anonymizer.add_operator("REPO_NUMBER", FakeRepoOperator())
# Anonymize the text
anonymized_text = anonymizer.anonymize(text=text)
print(anonymized_text.text)
This will replace the repo number with a realistic-looking 5-digit number instead of a placeholder.
Identifying Anonymized Entities
Sometimes, you might need to know which parts of the text were anonymized and what their original values were. Presidio provides a way to do this using the AnonymizerEngine
with the OperatorConfig.HASH
mode.
from presidio_anonymizer import AnonymizerEngine
from presidio_anonymizer.entities import OperatorConfig
# Initialize the anonymizer with reversible mode
anonymizer = AnonymizerEngine()
# Anonymize the text with placeholders
anonymized_result = anonymizer.anonymize(
text=text,
anonymizers={
"DEFAULT": OperatorConfig("replace", {"new_value": "<{entity_type}>"})
}
)
print(anonymized_result.text)
# Get the mapping of anonymized values
for item in anonymized_result.items:
print(f"{item.entity_type}: {text[item.start:item.end]} -> {item.anonymized_text}")
This will output the anonymized text with placeholders and provide a mapping of the original values to their anonymized versions.
Built-in Entities in Presidio
Presidio comes with several built-in entities that it can recognize and anonymize:
- PERSON
- EMAIL_ADDRESS
- PHONE_NUMBER
- IBAN_CODE
- CREDIT_CARD
- CRYPTO
- DATE_TIME
- DOMAIN_NAME
- IP_ADDRESS
- NRP
- LOCATION
- MEDICAL_LICENSE
- URL
- US_BANK_NUMBER
- US_DRIVER_LICENSE
- US_ITIN
- US_PASSPORT
- US_SSN
For any other types of sensitive information, you'll need to create custom recognizers as we did with the repo number example.
Best Practices for Data Anonymization
When implementing data anonymization in your projects, consider the following best practices:
-
Understand Your Data: Before anonymizing, thoroughly analyze your data to identify all types of sensitive information.
-
Use Consistent Anonymization: Ensure that the same piece of information is always anonymized in the same way across your dataset.
-
Test Thoroughly: Always test your anonymization process with various input data to ensure it's working as expected.
-
Keep Original Data Secure: Store the original, non-anonymized data securely and separately from the anonymized version.
-
Document Your Process: Keep detailed documentation of your anonymization process, including any custom patterns or operators used.
-
Regular Updates: Keep your anonymization tools and patterns up-to-date to handle new types of sensitive data that may emerge.
-
Compliance Check: Ensure your anonymization process complies with relevant data protection regulations in your industry and region.
-
Reversibility Consideration: Decide whether you need the ability to reverse the anonymization process, and if so, implement secure methods to do so.
-
Performance Optimization: For large datasets, optimize your anonymization process for performance to handle data efficiently.
-
Quality Assurance: Regularly review anonymized data to ensure the quality and realism of the anonymized information.
Advanced Techniques
Handling Structured Data
While we've focused on anonymizing free-text data, you might often need to anonymize structured data like JSON or CSV files. Here's an example of how you might approach this:
import json
from presidio_anonymizer import AnonymizerEngine
data = {
"customers": [
{"name": "John Doe", "email": "[email protected]", "phone": "123-456-7890"},
{"name": "Jane Smith", "email": "[email protected]", "phone": "987-654-3210"}
]
}
anonymizer = AnonymizerEngine()
def anonymize_dict(d):
for key, value in d.items():
if isinstance(value, str):
d[key] = anonymizer.anonymize(text=value).text
elif isinstance(value, dict):
anonymize_dict(value)
elif isinstance(value, list):
for item in value:
if isinstance(item, dict):
anonymize_dict(item)
anonymize_dict(data)
print(json.dumps(data, indent=2))
This recursive function will go through all string values in a nested dictionary or list structure and anonymize them.
Differential Privacy
For more advanced anonymization needs, especially when dealing with statistical data, you might want to explore differential privacy techniques. While Presidio doesn't directly support differential privacy, you can combine it with other libraries like IBM's diffprivlib:
from diffprivlib import mechanisms as mech
def anonymize_age(age, epsilon=0.1):
laplace = mech.Laplace(epsilon=epsilon, sensitivity=1, lower=0, upper=120)
return int(laplace.randomise(age))
# Usage
original_age = 35
anonymized_age = anonymize_age(original_age)
print(f"Original age: {original_age}, Anonymized age: {anonymized_age}")
This adds noise to the age value, making it harder to identify individuals while preserving the overall statistical properties of the dataset.
Challenges and Limitations
While tools like Presidio are powerful, it's important to be aware of their limitations:
-
Context Sensitivity: Anonymization tools may struggle with context-dependent information. For example, a seemingly innocent phrase might be identifying in a specific context.
-
New Types of Sensitive Data: As new forms of personal data emerge, anonymization tools need to be constantly updated to recognize and handle them.
-
Over-anonymization: There's a risk of over-anonymizing data to the point where it loses its utility for analysis or processing.
-
Under-anonymization: Conversely, not anonymizing enough can leave individuals vulnerable to re-identification.
-
Performance with Large Datasets: Anonymizing large volumes of data can be computationally expensive and time-consuming.
-
Multilingual Support: Many anonymization tools are primarily designed for English text and may struggle with other languages.
Future Trends in Data Anonymization
As data privacy concerns continue to grow, we can expect to see several trends in the field of data anonymization:
-
AI-Powered Anonymization: Machine learning models that can better understand context and identify sensitive information more accurately.
-
Federated Learning: Techniques that allow machine learning on decentralized data without exposing the raw data.
-
Homomorphic Encryption: Methods that allow computation on encrypted data without decrypting it.
-
Synthetic Data Generation: Creating entirely artificial datasets that maintain the statistical properties of the original data without containing any real personal information.
-
Blockchain for Data Privacy: Using blockchain technology to create immutable audit trails of data access and anonymization processes.
Conclusion
Protecting sensitive information is a critical aspect of data handling in today's digital landscape. Tools like Langchain and Presidio provide powerful capabilities for anonymizing data, but they require careful implementation and consideration of the specific needs of your data and use case.
By following the techniques and best practices outlined in this guide, you can significantly enhance the privacy protection of your data processing pipeline. Remember that anonymization is not a one-time task but an ongoing process that requires regular review and updates to stay effective against new privacy challenges and regulations.
As you implement these techniques, always stay informed about the latest developments in data privacy and anonymization. The field is rapidly evolving, and new tools and methods are constantly emerging to help you better protect sensitive information while maintaining the utility of your data.
By prioritizing data privacy and implementing robust anonymization practices, you not only comply with regulations but also build trust with your users and stakeholders, creating a strong foundation for responsible data usage in your organization.
Article created from: https://www.youtube.com/watch?v=YaPYpWk22bE