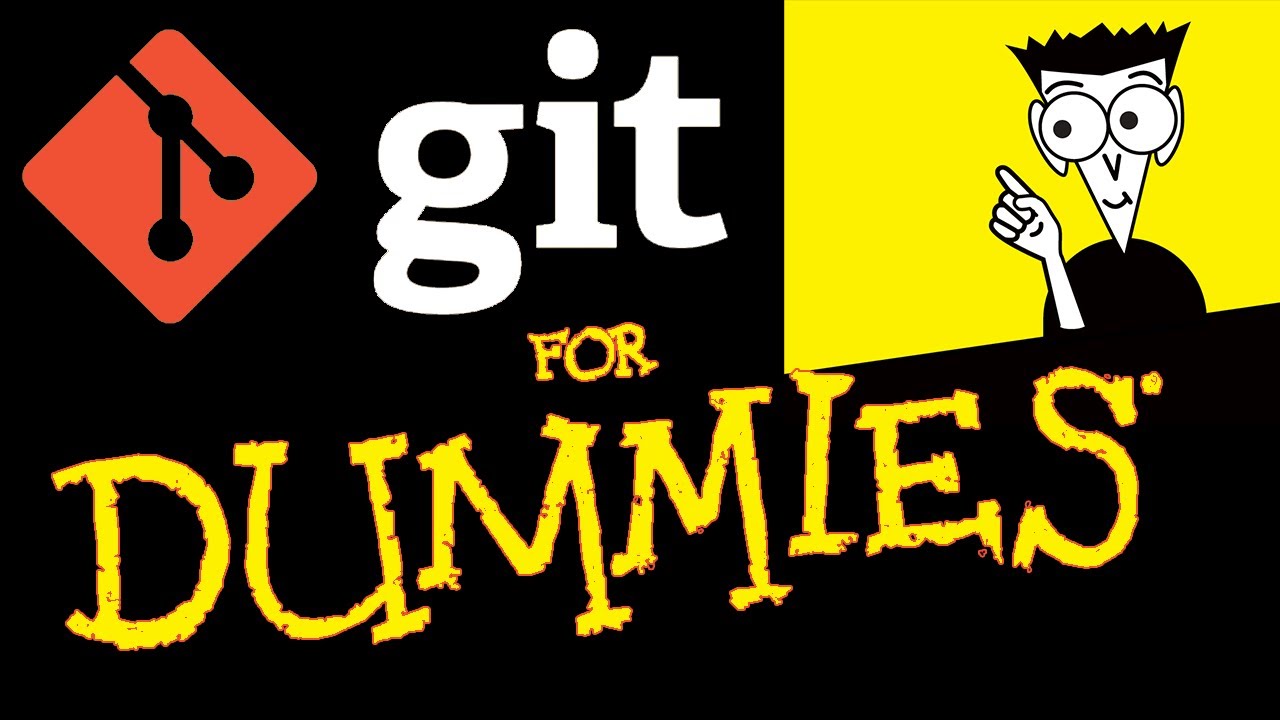
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Git and GitHub
Version control is an essential skill for any programmer. However, many tutorials overcomplicate Git and GitHub, making it seem more daunting than it really is. This guide aims to break down the core concepts and commands in simple, easy-to-understand terms.
What is Git?
Git is version control software that comes pre-installed on Mac and Linux systems. Windows users need to download Git Bash to use Git commands.
At its core, Git acts like a memory card for your code. It allows you to save your progress as you work on a project, much like saving your game periodically so you don't lose progress if something goes wrong.
Why Learn Git?
Git is a fundamental skill for programmers. You simply cannot be successful in programming without knowing how to use Git. It's as essential as knowing how to use the command line or write code itself.
Basic Git Workflow
Let's walk through a basic Git workflow to understand the core commands:
1. Initializing a Git Repository
To start using Git in a project, you first need to initialize a Git repository in your project folder:
git init
This is equivalent to inserting a memory card into your game system - it allows you to start saving your progress.
2. Saving Your Progress
To save your progress in Git, you use two commands:
git add .
git commit -m "Your commit message"
git add .
stages all changes in your current directory for the commit. You can also specify individual files instead of using the period.
git commit
actually saves your progress. The -m
flag allows you to add a message describing what changes you made.
3. Viewing Your Save History
To view a log of all your saved changes:
git log
This shows you a list of all your commits, including the commit message, date, and a unique identifier (hash) for each commit.
4. Going Back in Time
If you need to revert to a previous version of your code, you can use the git checkout
command with the commit hash:
git checkout [commit-hash]
This puts you in a "detached HEAD" state, which is like traveling back in time in your project history.
What is GitHub?
GitHub is a web-based platform that uses Git for version control. It's similar to other platforms like BitBucket and GitLab. GitHub allows you to store your Git repositories online, making it easier to collaborate with others and share your code.
Using GitHub
1. Creating a Repository
To use GitHub, you first need to create an account. Once you have an account, you can create a new repository:
- Click on "New repository" on your GitHub profile
- Name your repository
- Choose whether it should be public or private
- Click "Create repository"
2. Connecting Local Repository to GitHub
After creating a repository on GitHub, you'll need to connect your local Git repository to it. GitHub provides the commands for this:
git remote add origin [URL of your GitHub repository]
git branch -M main
git push -u origin main
3. Pushing Changes to GitHub
Once your local repository is connected to GitHub, you can push your commits to GitHub:
git push origin main
This uploads all your committed changes to GitHub.
4. Pulling Changes from GitHub
If there are changes on GitHub that you don't have locally, you can pull them down:
git pull origin main
This downloads and merges any changes from GitHub into your local repository.
Understanding Branches
Branches in Git allow you to diverge from the main line of development and work on different features or experiments without affecting the main code.
Creating a New Branch
To create a new branch:
git checkout -b [branch-name]
This creates a new branch and switches to it.
Switching Between Branches
To switch to an existing branch:
git checkout [branch-name]
Merging Branches
When you're ready to bring your changes from a branch back into the main code:
- Switch to the main branch:
git checkout main
- Merge the other branch:
git merge [branch-name]
Collaboration with GitHub
GitHub makes it easy to collaborate with others on projects.
Pull Requests
When you want to suggest changes to someone else's repository:
- Fork their repository
- Make your changes in a new branch
- Push your branch to your fork
- Open a pull request on the original repository
The repository owner can then review your changes and decide whether to merge them.
Best Practices
- Commit often: Make small, frequent commits rather than large, infrequent ones.
- Write clear commit messages: Describe what changes you made and why.
- Use branches for new features or experiments.
- Pull before you push: Always pull the latest changes before pushing your own to avoid conflicts.
- Review your changes before committing: Use
git diff
to see what you're about to commit.
Common Git Commands Summary
-
git init
: Initialize a new Git repository -
git add .
: Stage all changes for commit -
git commit -m "message"
: Commit staged changes -
git status
: Check the status of your working directory -
git log
: View commit history -
git checkout [branch-name]
: Switch to a different branch -
git branch
: List all branches -
git merge [branch-name]
: Merge a branch into the current branch -
git pull
: Fetch and merge changes from the remote repository -
git push
: Push local changes to the remote repository
Conclusion
Git and GitHub are powerful tools that every programmer should be comfortable using. While they may seem complex at first, the basic workflow is straightforward: make changes, stage them, commit them, and push them to GitHub. With practice, these commands will become second nature, allowing you to focus on writing great code and collaborating effectively with others.
Remember, the key to mastering Git and GitHub is consistent use. Start incorporating these tools into your daily coding practice, and you'll soon wonder how you ever managed without them. Happy coding!
Article created from: https://www.youtube.com/watch?v=mJ-qvsxPHpY&list=WL&index=2&t=48s