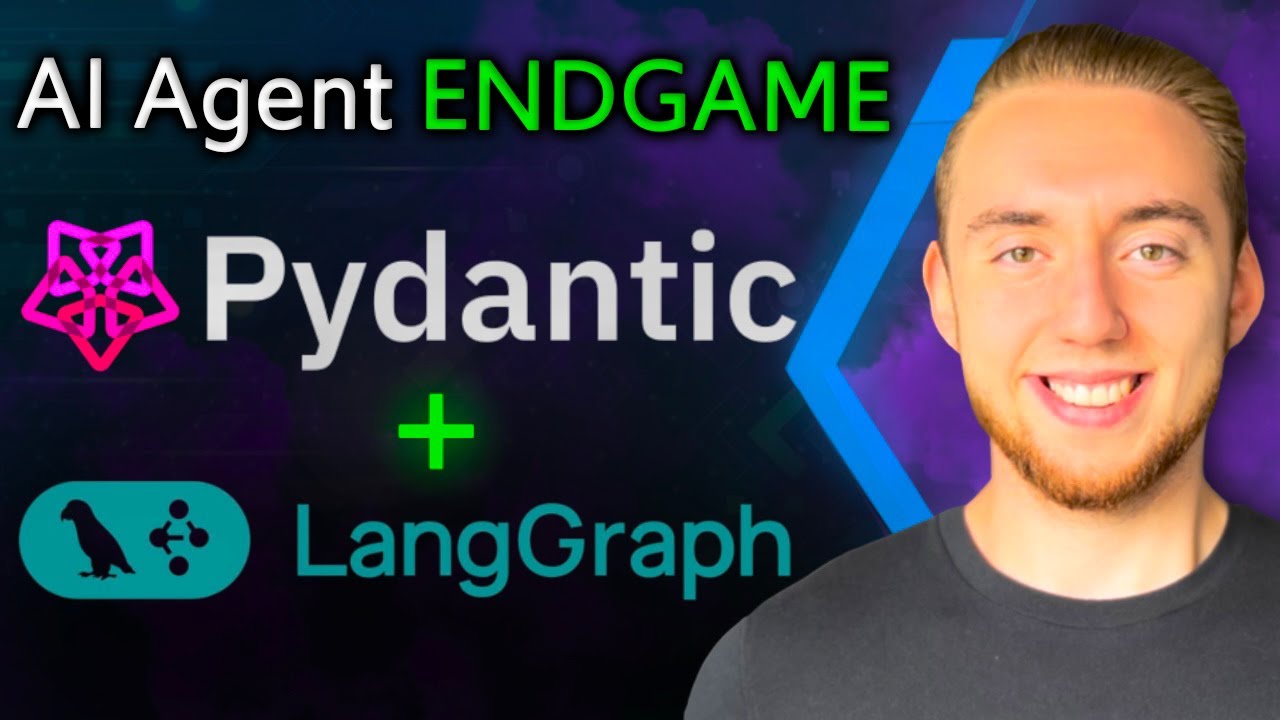
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Advanced AI Agent Development
As artificial intelligence continues to evolve, the ability to create sophisticated AI agents has become increasingly important. While there are many guides available online for building basic AI agents, most only cover the fundamentals. For those looking to develop truly advanced AI systems, a deeper understanding of powerful frameworks and tools is essential.
In this article, we'll explore how to build highly capable AI agents by combining two powerful technologies:
- Pydantic AI - A Python framework for easily building customizable AI agents
- LangGraph - An expressive workflow builder for orchestrating multiple AI agents
By leveraging these tools together, we can create AI systems that go far beyond simple chatbots or question-answering agents. We'll walk through building an AI agent called Archon that is actually capable of creating other AI agents - a truly impressive demonstration of what's possible with modern AI development techniques.
Understanding Pydantic AI
Pydantic AI is a Python framework that makes it straightforward to build customizable AI agents while still providing granular control over key aspects like testing, function calling, and chat history management.
The framework breaks down agent creation into three main components:
- Dependencies - API keys, database connections, and other resources the agent needs
- Agent definition - System prompt, language model selection, etc.
- Tool functions - Python functions that allow the agent to take actions
Let's look at a simple example of a weather agent built with Pydantic AI:
# Dependencies
from pydantic import BaseModel
from typing import List
class WeatherDependencies(BaseModel):
api_key: str
locations: List[str]
# Agent definition
from pydantic_agent import Agent
weather_agent = Agent(
name="WeatherAgent",
description="Agent for retrieving weather information",
model="gpt-3.5-turbo"
)
# Tool function
@weather_agent.tool()
def get_weather(location: str) -> str:
# Implementation to fetch weather data
pass
This structure makes it easy to define complex agents while keeping the code organized and maintainable.
Introducing LangGraph
While Pydantic AI excels at building individual agents, LangGraph provides the tools to orchestrate multiple agents into sophisticated workflows. It allows us to define how different AI agents interact and reason together to solve complex problems.
LangGraph uses a graph-based approach where:
- Nodes represent individual agents or processing steps
- Edges define the flow of information between nodes
This structure enables the creation of non-deterministic workflows, where the path through the graph can change dynamically based on intermediate results or user input.
A key strength of LangGraph is its low-level abstractions. Unlike some frameworks that try to automate everything, LangGraph provides fine-grained control while still handling the complexities of managing state and orchestrating multiple agents.
The Power of Combining Pydantic AI and LangGraph
By using Pydantic AI to build our individual agents and LangGraph to orchestrate them, we can create AI systems with capabilities far beyond what either framework could achieve alone. This combination allows us to:
- Build highly customized agents tailored to specific tasks
- Orchestrate complex workflows involving multiple specialized agents
- Create dynamic, adaptive AI systems that can handle a wide range of scenarios
To demonstrate the potential of this approach, we'll walk through building Archon - an AI agent capable of creating other AI agents.
Building Archon: An AI Agent Creator
Archon is designed to take a user's requirements for an AI agent and generate the necessary code and configuration to bring that agent to life. It leverages multiple sub-agents working together in a LangGraph workflow:
- Reasoning Agent - Analyzes user requirements and creates a scope document
- Coder Agent - Generates the actual code for the new AI agent
- Router Agent - Determines if further iterations are needed based on user feedback
- Finalization Agent - Summarizes the created agent and provides usage instructions
Let's break down the key components of Archon's implementation.
Setting Up the Environment
First, we need to import the necessary libraries and set up our configuration:
import os
from typing import Dict, Any
from dotenv import load_dotenv
from langchain.chat_models import ChatOpenAI
from pydantic import BaseModel
from langgraph.graph import Graph, END
from langgraph.prebuilt.tool_executor import ToolExecutor
# Load environment variables
load_dotenv()
# Configure logging
import logfire
logfire.configure()
# Set up API keys and base URLs
BASE_URL = os.getenv("BASE_URL")
LLM_API_KEY = os.getenv("LLM_API_KEY")
IS_OLLAMA = "localhost" in BASE_URL
This setup ensures we have access to our API keys and can configure the appropriate language models.
Defining the Reasoning Agent
The Reasoning Agent is responsible for analyzing the user's requirements and creating a scope document for the new AI agent:
reasoner_llm = ChatOpenAI(
model_name=os.getenv("REASONER_MODEL", "gpt-3.5-turbo"),
openai_api_key=LLM_API_KEY,
base_url=BASE_URL
)
reasoner_agent = Agent(
name="ReasonerAgent",
llm=reasoner_llm,
system_prompt="You are an AI agent designer. Your task is to create a detailed scope document for new AI agents based on user requirements."
)
@reasoner_agent.tool()
def create_scope_document(requirements: str) -> str:
# Implementation to create scope document
pass
Implementing the Coder Agent
The Coder Agent takes the scope document and generates the actual code for the new AI agent:
coder_llm = ChatOpenAI(
model_name=os.getenv("CODER_MODEL", "gpt-4"),
openai_api_key=LLM_API_KEY,
base_url=BASE_URL
)
coder_agent = Agent(
name="CoderAgent",
llm=coder_llm,
system_prompt="You are an expert AI developer. Your task is to generate code for new AI agents based on provided scope documents."
)
@coder_agent.tool()
def generate_agent_code(scope_document: str) -> str:
# Implementation to generate agent code
pass
Creating the Router Agent
The Router Agent determines whether to continue iterating on the agent or finalize the conversation:
router_agent = Agent(
name="RouterAgent",
llm=coder_llm, # Reusing the coder LLM for simplicity
system_prompt="You are a decision-making agent. Determine if the conversation should continue or if the agent creation process is complete."
)
@router_agent.tool()
def route_conversation(user_feedback: str) -> str:
# Implementation to decide next action
pass
Defining the Finalization Agent
The Finalization Agent summarizes the created agent and provides usage instructions:
finalization_agent = Agent(
name="FinalizationAgent",
llm=coder_llm, # Reusing the coder LLM
system_prompt="You are responsible for summarizing the created AI agent and providing clear usage instructions."
)
@finalization_agent.tool()
def finalize_agent(agent_code: str) -> str:
# Implementation to summarize and provide instructions
pass
Orchestrating the Workflow with LangGraph
Now that we have our individual agents defined, we can use LangGraph to orchestrate them into a cohesive workflow:
class ArchonState(BaseModel):
messages: List[Dict[str, Any]] = []
scope: str = ""
agent_code: str = ""
latest_user_message: str = ""
workflow = Graph()
workflow.add_node("reason", reasoner_agent)
workflow.add_node("code", coder_agent)
workflow.add_node("route", router_agent)
workflow.add_node("finalize", finalization_agent)
workflow.add_edge("reason", "code")
workflow.add_edge("code", "route")
workflow.add_edge("route", "code")
workflow.add_edge("route", "finalize")
workflow.add_edge("finalize", END)
workflow.set_entry_point("reason")
This graph structure defines how our agents interact, allowing for iterative improvement of the generated agent based on user feedback.
Executing the Workflow
To run our Archon agent, we can use the following code:
def run_archon(user_request: str):
state = ArchonState(latest_user_message=user_request)
final_state = workflow.run(state)
return final_state.agent_code
# Example usage
user_request = "Create an AI agent that can summarize long articles"
generated_agent = run_archon(user_request)
print(generated_agent)
This will execute our entire workflow, generating a new AI agent based on the user's request.
Advantages of the Pydantic AI + LangGraph Approach
By combining Pydantic AI and LangGraph in this way, we gain several significant advantages:
-
Modularity: Each agent is self-contained and can be easily modified or replaced without affecting the overall system.
-
Flexibility: The graph-based workflow allows for complex decision-making and iterative processes.
-
Scalability: New agents and nodes can be added to the workflow as needed to handle more complex tasks.
-
Maintainability: The clear separation of concerns makes the codebase easier to understand and maintain.
-
Reusability: Individual agents can be reused in multiple workflows or projects.
Potential Applications
The techniques demonstrated in building Archon can be applied to a wide range of AI development projects:
- Automated Code Generation: Create specialized code generators for different programming languages or frameworks.
- Complex Data Analysis: Build workflows that combine data retrieval, analysis, and visualization agents.
- Intelligent Task Automation: Develop systems that can break down complex tasks and delegate them to specialized agents.
- Advanced Chatbots: Create chatbots that can dynamically adapt their behavior based on user interactions.
- AI-Assisted Design: Build agents that can collaborate on design tasks, from UI mockups to 3D modeling.
Challenges and Considerations
While the combination of Pydantic AI and LangGraph is powerful, there are some challenges to keep in mind:
-
Complexity: As workflows grow more complex, managing state and ensuring proper flow between agents can become challenging.
-
Performance: Running multiple AI models in a single workflow can be computationally expensive and may require optimization.
-
Testing: Thoroughly testing complex AI workflows requires careful consideration and potentially specialized testing frameworks.
-
Ethical Considerations: As AI systems become more capable of generating code and other AI agents, it's crucial to implement safeguards and ethical guidelines.
Future Directions
The field of AI agent development is rapidly evolving, and there are several exciting directions for future work:
-
Improved Self-Modification: Developing agents that can not only create other agents but also modify and improve themselves.
-
Enhanced Reasoning Capabilities: Incorporating more advanced reasoning techniques to allow agents to handle increasingly complex tasks.
-
Multi-Modal Agents: Extending the framework to support agents that can work with various types of data, including text, images, and audio.
-
Federated Learning: Developing workflows that allow multiple instances of Archon to learn collaboratively while maintaining data privacy.
-
Integration with Other AI Technologies: Exploring how Pydantic AI and LangGraph can be combined with other cutting-edge AI technologies like reinforcement learning or neuro-symbolic AI.
Conclusion
The combination of Pydantic AI and LangGraph represents a powerful approach to building advanced AI agents. By leveraging the strengths of both frameworks, developers can create sophisticated, adaptable AI systems capable of handling complex tasks and workflows.
Archon, our AI agent creator, demonstrates the potential of this approach. While it's a relatively simple example, it showcases how multiple specialized agents can work together to achieve a complex goal - in this case, the creation of new AI agents based on user specifications.
As AI technology continues to advance, tools like Pydantic AI and LangGraph will play an increasingly important role in helping developers harness the full potential of artificial intelligence. Whether you're building specialized AI assistants, complex data analysis systems, or pushing the boundaries of what's possible with AI, these frameworks provide a solid foundation for your projects.
By mastering these tools and techniques, you'll be well-equipped to tackle the AI development challenges of today and tomorrow. The future of AI is collaborative, adaptive, and incredibly powerful - and it's being built with tools like Pydantic AI and LangGraph.
Article created from: https://youtu.be/U6LbW2IFUQw?si=qXEz4rWEIWLhi_bh