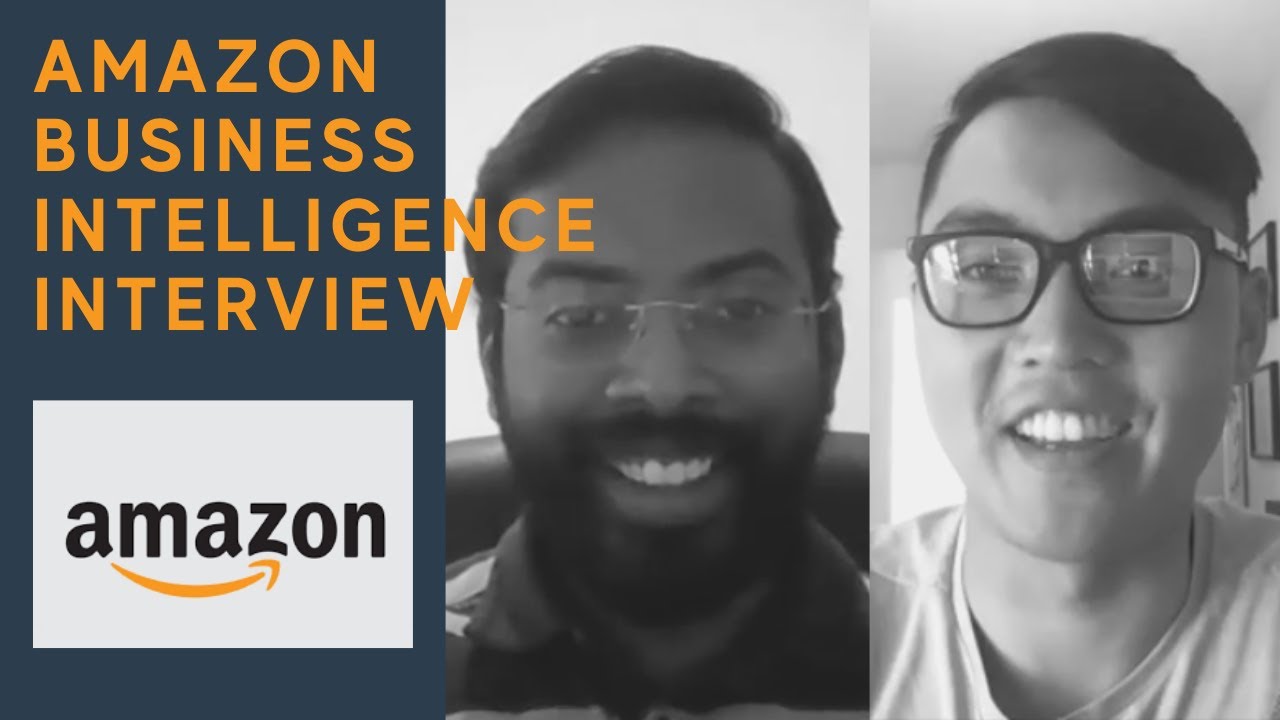
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to E-commerce Product Deduplication
E-commerce platforms face a significant challenge in managing duplicate product listings. With millions of items listed by various sellers, it's common to find multiple entries for the same product, often with slight variations in naming or description. This issue can lead to a cluttered user experience, inconsistent pricing, and difficulties in inventory management. In this article, we'll explore strategies for tackling this problem at scale, focusing on techniques that can be applied to large databases with minimal manual intervention.
The Importance of Product Deduplication
Before diving into the technical aspects, it's crucial to understand why product deduplication is essential for e-commerce platforms:
- Improved user experience
- Accurate inventory management
- Consistent pricing across listings
- Better search functionality
- Enhanced data quality for analytics
Initial Approaches to Deduplication
When faced with the task of deduplicating products, several initial strategies come to mind:
Unique Identifier Matching
In an ideal scenario, each product would have a unique identifier such as an SKU (Stock Keeping Unit) or ASIN (Amazon Standard Identification Number). This approach involves:
- Collecting all SKUs across different sellers
- Performing a distinct count of SKUs
- Identifying duplicates based on repeated SKUs
However, this method assumes that all sellers use consistent SKUs, which isn't always the case, especially on platforms that allow third-party listings.
Image Similarity Analysis
When unique identifiers are unavailable or inconsistent, analyzing product images can be an effective approach:
- Use image recognition algorithms to identify similar product images
- Compare visual features across listings
- Group products with highly similar images
This method can be particularly useful for visually distinct products but may struggle with generic items or products with multiple variants.
Text-Based Similarity
Analyzing product descriptions and titles using natural language processing techniques:
- Tokenize and clean text data
- Apply techniques like TF-IDF (Term Frequency-Inverse Document Frequency)
- Calculate similarity scores between product descriptions
- Group products with high textual similarity
This approach can catch variations in naming conventions but may require fine-tuning to avoid false positives.
Review Content Analysis
Leveraging customer reviews to identify potential duplicates:
- Aggregate reviews for each product
- Analyze review content for similarities across products
- Identify products with highly similar review patterns
This method can be particularly effective for popular products with many reviews but may be less reliable for newer or less frequently purchased items.
Scaling the Deduplication Process
When dealing with thousands or millions of products, manual review of potential duplicates becomes impractical. Here are strategies to scale the deduplication process:
Unsupervised Clustering
Implementing unsupervised machine learning techniques to group similar products:
- Feature extraction from product data (text, images, price, etc.)
- Apply clustering algorithms (e.g., K-means, DBSCAN)
- Analyze cluster characteristics to identify potential duplicate groups
Feature Engineering for Improved Clustering
To enhance the effectiveness of clustering, consider incorporating additional features:
- Product attributes (color, size, material)
- Price range
- Brand information
- Release date or model year
- Seller characteristics (e.g., electronics specialist vs. general merchant)
Hybrid Approach: Combining Multiple Signals
Integrate multiple data points to create a more robust deduplication system:
- Assign weights to different similarity measures (image, text, price, etc.)
- Calculate a composite similarity score
- Set thresholds for automatic deduplication and manual review
Implementing a Confidence Scoring System
Develop a scoring system to prioritize deduplication efforts:
- Assign confidence scores to potential duplicates
- Automatically merge high-confidence matches
- Flag medium-confidence matches for quick human review
- Ignore or deprioritize low-confidence matches
Optimizing Manual Intervention
While the goal is to automate as much as possible, some level of human oversight is often necessary. Here's how to optimize the manual review process:
Prioritization Based on Business Impact
Focus manual efforts on products where accuracy is most critical:
- High-value items (e.g., electronics, luxury goods)
- Best-selling products
- Items with significant price discrepancies across listings
Sampling for Quality Control
Implement a sampling strategy to validate the automated process:
- Randomly select a percentage of automated deduplications for review
- Adjust algorithms based on findings from manual checks
Crowdsourcing for Scale
Leverage the power of the crowd to handle manual reviews:
- Develop a simple interface for comparing potential duplicates
- Utilize services like Amazon Mechanical Turk for large-scale human input
- Implement quality control measures to ensure accuracy of crowdsourced decisions
Continuous Improvement and Monitoring
Deduplication is an ongoing process that requires constant refinement:
Feedback Loop
Establish a system to incorporate learnings from manual reviews:
- Track common patterns in false positives and negatives
- Regularly update algorithms based on new insights
- Adjust confidence thresholds as the system improves
Performance Metrics
Develop key performance indicators (KPIs) to measure the effectiveness of the deduplication process:
- Reduction in duplicate listings over time
- Accuracy rate of automated deduplication
- Time saved through automation vs. manual review
A/B Testing
Continuously test and refine the deduplication algorithm:
- Run A/B tests on different clustering methods
- Experiment with various feature combinations
- Optimize thresholds for automatic vs. manual review
Technical Implementation Considerations
When implementing a large-scale deduplication system, several technical aspects need to be addressed:
Scalable Infrastructure
Ensure the system can handle the volume of data:
- Utilize distributed computing frameworks like Apache Spark
- Implement efficient data storage solutions (e.g., NoSQL databases)
- Consider cloud-based solutions for flexibility and scalability
Real-time vs. Batch Processing
Decide on the timing of deduplication efforts:
- Real-time checking for new listings
- Periodic batch processing for existing inventory
- Hybrid approach based on product categories or listing frequency
API Integration
Develop APIs to integrate the deduplication system with other platform components:
- Listing management systems
- Search functionality
- Inventory tracking
- Analytics dashboards
Handling Edge Cases
Every deduplication system will encounter challenging scenarios. Here are some edge cases to consider:
Bundle Products
Products sold as part of a bundle can be particularly tricky:
- Develop rules for comparing individual items vs. bundles
- Consider bundle-specific attributes in the similarity calculation
Customizable Products
Items that can be customized or personalized require special handling:
- Identify base product similarities while accounting for customization options
- Develop separate rules for comparing customizable vs. standard products
Seasonal or Limited Edition Items
Products that change regularly or have limited runs pose unique challenges:
- Incorporate time-based features in the similarity calculation
- Develop strategies for archiving and reactivating seasonal listings
Legal and Ethical Considerations
When implementing a deduplication system, it's important to consider the legal and ethical implications:
Seller Rights
Ensure the deduplication process respects seller agreements:
- Develop clear policies on how duplicate listings are handled
- Provide mechanisms for sellers to dispute automated deduplication decisions
Data Privacy
Be mindful of data protection regulations:
- Ensure compliance with laws like GDPR when processing seller and customer data
- Implement data anonymization techniques where appropriate
Fairness and Bias
Regularly audit the deduplication system for potential biases:
- Check for unintended consequences on specific product categories or seller types
- Ensure the system doesn't disproportionately affect certain groups of sellers
Future Trends in E-commerce Deduplication
As technology evolves, new opportunities for improving deduplication processes emerge:
Advanced AI and Machine Learning
Leveraging cutting-edge AI technologies:
- Deep learning models for improved image and text similarity
- Reinforcement learning for optimizing deduplication decisions
- Natural language processing advancements for better understanding of product descriptions
Blockchain for Product Verification
Exploring blockchain technology to enhance product authenticity:
- Creating immutable records of product origins
- Facilitating cross-platform product identification
IoT and Smart Tagging
Utilizing Internet of Things (IoT) devices for improved product tracking:
- Smart tags that provide unique identifiers for physical products
- Integration with inventory management systems for real-time tracking
Conclusion
Deduplicating products on large e-commerce platforms is a complex challenge that requires a multifaceted approach. By combining advanced algorithms, scalable infrastructure, and strategic human oversight, it's possible to significantly reduce duplicate listings and improve the overall quality of the product catalog.
Key takeaways for implementing an effective deduplication system include:
- Utilize multiple data points for similarity assessment
- Implement unsupervised clustering techniques for scalability
- Develop a confidence scoring system to optimize manual review
- Continuously refine algorithms through feedback loops and A/B testing
- Address technical challenges with scalable infrastructure and API integrations
- Consider legal and ethical implications of automated deduplication
As e-commerce continues to grow, the importance of efficient product deduplication will only increase. By staying ahead of technological trends and continuously refining their approaches, platforms can ensure a cleaner, more user-friendly shopping experience for their customers while maintaining a fair and manageable environment for sellers.
Implementing these strategies requires a significant investment in technology and processes, but the long-term benefits in terms of user satisfaction, data quality, and operational efficiency make it a worthwhile endeavor for any serious e-commerce player in today's competitive digital marketplace.
Article created from: https://www.youtube.com/watch?v=5ZaYUgPxs_w