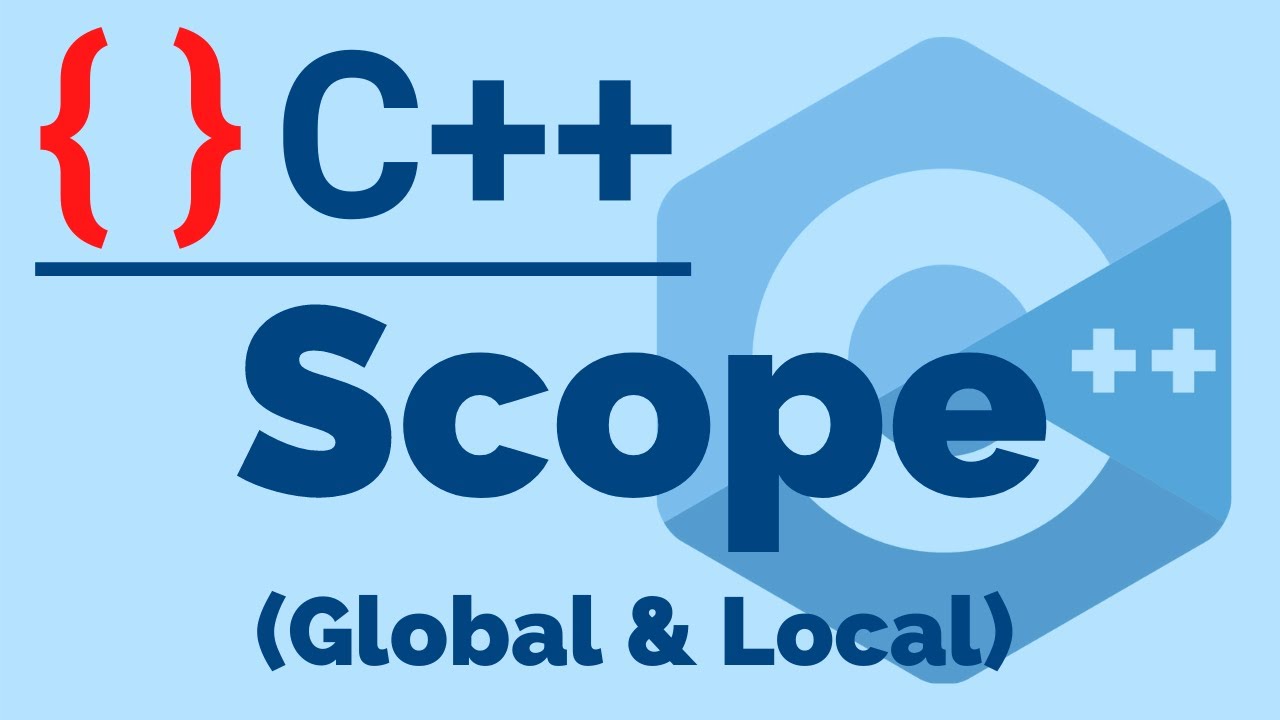
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Global and Local Scope in C++
In the world of C++ programming, understanding the concept of scope is crucial for writing efficient and bug-free code. This article delves into the nuances of global and local scope, using a practical example to illustrate how variables behave in different contexts.
The Problem at Hand
Let's examine a C++ code snippet that demonstrates the interplay between global and local variables:
int x = 1; // Global variable
int main() {
int& y = x; // Reference to global x
int x = 2; // Local variable x
cout << y << " " << x << endl;
return 0;
}
At first glance, this code might seem straightforward, but it raises some interesting questions about variable scope and visibility.
Breaking Down the Code
Global Variable Declaration
The code begins with the declaration of a global variable:
int x = 1;
This variable has global scope, meaning it's accessible throughout the entire program. It's initialized with the value 1.
Entering the Main Function
Inside the main()
function, we encounter our first local operation:
int& y = x;
Here, we're creating a reference y
that aliases the global variable x
. At this point, y
is essentially another name for the global x
.
Local Variable Declaration
The next line introduces a twist:
int x = 2;
This declares a new variable x
within the local scope of the main()
function. It's important to note that this x
is distinct from the global x
. The local x
is initialized with the value 2.
Printing the Values
Finally, we print the values of y
and x
:
cout << y << " " << x << endl;
The Output Explained
When we run this code, the output is:
1 2
Let's break down why we get this result:
- The value 1 is printed for
y
becausey
is a reference to the globalx
, which was initialized to 1. - The value 2 is printed for
x
because within themain()
function,x
refers to the local variable, not the global one.
Key Concepts Illustrated
Variable Shadowing
This example demonstrates a concept known as variable shadowing. The local variable x
in the main()
function shadows (or hides) the global variable x
. Within the main()
function, any direct reference to x
will refer to the local variable, not the global one.
Reference Binding
The reference y
is bound to the global x
at the point of its declaration. This binding doesn't change even when a local x
is introduced later in the same scope.
Scope Resolution
When the compiler encounters a variable name, it follows a specific order to resolve which variable is being referred to:
- It first looks in the current block.
- If not found, it moves to the enclosing block.
- This process continues outward until it reaches the global scope.
Best Practices and Considerations
Avoiding Global Variables
While this example uses a global variable to illustrate scope concepts, it's generally considered good practice to minimize the use of global variables in real-world applications. Global variables can lead to:
- Reduced code readability
- Increased potential for naming conflicts
- Difficulties in tracking state changes
Using Scope Resolution Operator
In situations where you need to access a global variable that's shadowed by a local variable, you can use the scope resolution operator ::
:
cout << ::x << endl; // Prints the value of the global x
Const References
When using references, especially to global variables, consider using const references if you don't intend to modify the referenced value:
const int& y = x;
This prevents accidental modifications and makes the code's intentions clearer.
Common Pitfalls and How to Avoid Them
Unintended Variable Shadowing
Variable shadowing, as demonstrated in our example, can sometimes lead to unintended consequences. To avoid this:
- Use distinct names for local and global variables.
- If you must use the same name, be explicit about which variable you're referring to.
Initialization of References
References must be initialized when declared. Failing to do so will result in a compilation error:
int& z; // Error: references must be initialized
Dangling References
Be cautious about creating references to local variables that go out of scope:
int& getDanglingReference() {
int local = 5;
return local; // Dangerous: returns reference to local variable
}
This can lead to undefined behavior when the reference is used after the function returns.
Advanced Scope Concepts
Function Scope
In addition to global and local scope, C++ has function scope. This applies to labels used with goto statements:
void function() {
goto label; // Valid
{
label: // This label has function scope
// Some code
}
}
Namespace Scope
Namespaces provide another level of scope in C++:
namespace MyNamespace {
int x = 10; // This x is in the scope of MyNamespace
}
int main() {
cout << MyNamespace::x << endl; // Accessing x from MyNamespace
return 0;
}
Block Scope
Variables declared within a block (enclosed by curly braces) have block scope:
int main() {
{
int blockVar = 5; // blockVar has block scope
}
// blockVar is not accessible here
return 0;
}
Practical Applications of Scope Understanding
Encapsulation in Classes
Understanding scope is crucial when working with classes in C++. Class members can have different access specifiers (public, private, protected), which determine their visibility:
class MyClass {
private:
int privateVar;
public:
void setVar(int val) { privateVar = val; }
int getVar() { return privateVar; }
};
Avoiding Name Collisions in Large Projects
In large projects, using namespaces and being mindful of scope can help avoid name collisions:
namespace ProjectA {
void function() { /* ... */ }
}
namespace ProjectB {
void function() { /* ... */ }
}
int main() {
ProjectA::function(); // Calls function from ProjectA
ProjectB::function(); // Calls function from ProjectB
return 0;
}
Scope and Memory Management
Understanding scope is also important for effective memory management in C++.
Stack vs Heap Allocation
Variables with local scope are typically allocated on the stack, while dynamically allocated memory (using new
) is on the heap:
void function() {
int stackVar; // Allocated on the stack
int* heapVar = new int; // Allocated on the heap
delete heapVar; // Don't forget to deallocate heap memory
}
RAII (Resource Acquisition Is Initialization)
C++ uses the RAII principle, where resource management is tied to object lifetime. This is closely related to scope:
class ResourceManager {
public:
ResourceManager() { /* Acquire resource */ }
~ResourceManager() { /* Release resource */ }
};
void function() {
ResourceManager rm; // Resource acquired
// Use resource
} // Resource automatically released when rm goes out of scope
Scope in Modern C++ Features
Lambda Expressions
Lambda expressions in C++ have their own scope rules, particularly regarding capture clauses:
int main() {
int x = 10;
auto lambda = [x]() { return x * 2; };
cout << lambda() << endl; // Prints 20
return 0;
}
Range-based For Loops
Variables declared in range-based for loops have a scope limited to the loop:
int main() {
vector<int> numbers = {1, 2, 3, 4, 5};
for (const auto& num : numbers) {
// num is only in scope within this loop
cout << num << " ";
}
// num is not accessible here
return 0;
}
Debugging Scope-Related Issues
When debugging C++ programs, understanding scope can be crucial:
- Use debugger watch windows to inspect variable values in different scopes.
- Be aware of how scope affects variable lifetime and visibility.
- Pay attention to compiler warnings about shadowed variables.
Conclusion
Mastering the concepts of global and local scope in C++ is fundamental to writing clear, efficient, and bug-free code. Through the practical example we've explored, we've seen how variable declarations, references, and scope resolution work together in a C++ program.
Key takeaways include:
- Global variables have program-wide scope but should be used judiciously.
- Local variables can shadow global variables of the same name.
- References bind to variables based on the scope at the point of declaration.
- Understanding scope is crucial for proper resource management and avoiding common pitfalls.
By applying these concepts in your C++ programming, you'll be better equipped to write robust and maintainable code. Remember to always consider the scope of your variables and how they interact with different parts of your program. Happy coding!
Article created from: https://www.youtube.com/watch?v=NLFkjgeTIvQ