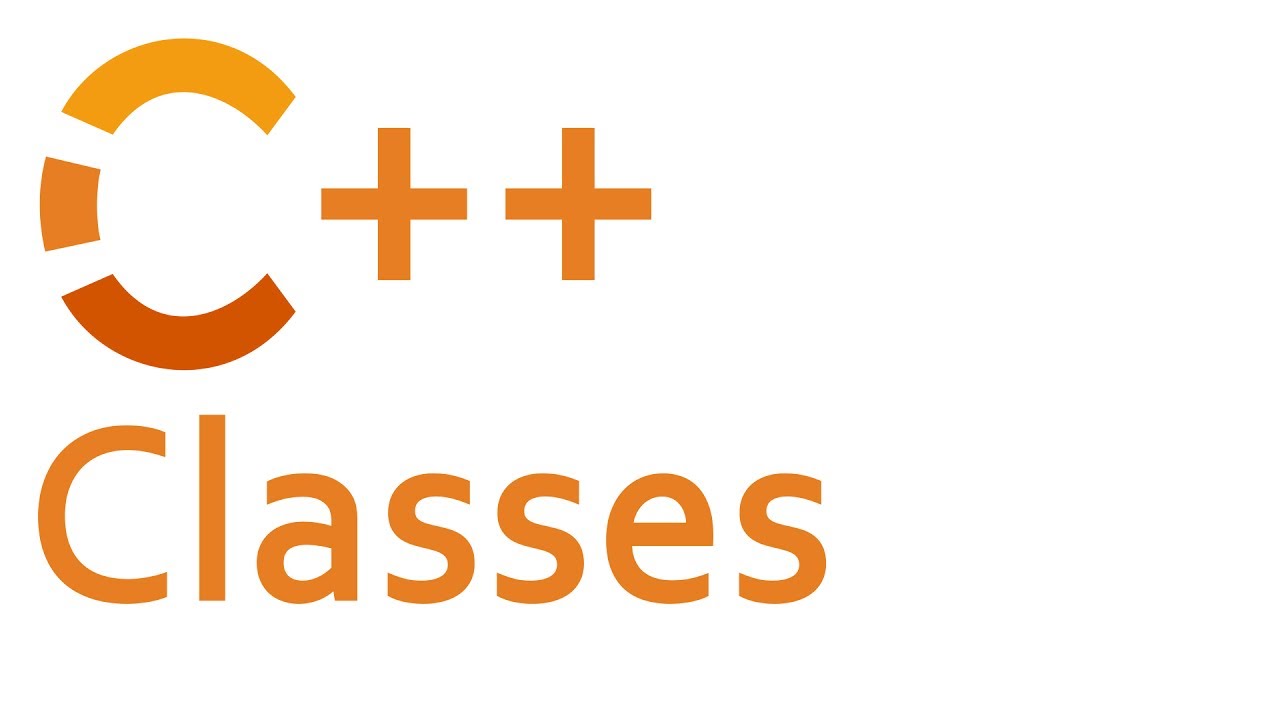
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to C++ Classes and Object-Oriented Programming
C++ is a versatile programming language that supports multiple programming paradigms, including object-oriented programming (OOP). One of the key features that enables OOP in C++ is the concept of classes. In this comprehensive guide, we'll delve into the world of C++ classes, exploring their structure, benefits, and how they form the foundation of object-oriented programming.
What Are C++ Classes?
Classes in C++ are user-defined data types that encapsulate data and functions into a single unit. They serve as blueprints for creating objects, which are instances of the class. This encapsulation allows for better organization of code and promotes the principle of data abstraction.
The Structure of a C++ Class
A basic C++ class consists of the following components:
- Class keyword
- Class name
- Access specifiers (public, private, protected)
- Data members (variables)
- Member functions (methods)
Here's a simple example of a C++ class:
class Player {
public:
int x;
int y;
int speed;
void move(int xa, int ya) {
x += xa * speed;
y += ya * speed;
}
};
In this example, we've defined a Player
class with three data members (x, y, and speed) and one member function (move).
The Benefits of Using Classes in C++
Classes offer several advantages in C++ programming:
-
Code Organization: Classes help group related data and functions together, making the code more organized and easier to manage.
-
Data Encapsulation: By bundling data and methods that operate on that data, classes provide a level of encapsulation that helps protect the data from unauthorized access.
-
Code Reusability: Once a class is defined, it can be used to create multiple objects, promoting code reuse.
-
Abstraction: Classes allow you to create abstract data types, hiding complex implementation details behind a simple interface.
-
Modularity: Large programs can be broken down into smaller, more manageable pieces using classes.
Creating Objects from Classes
Once a class is defined, you can create objects (instances) of that class. Here's how you can create an object of the Player
class:
Player player1;
This creates a new Player
object called player1
. You can then access its members using the dot notation:
player1.x = 0;
player1.y = 0;
player1.speed = 2;
player1.move(1, -1);
Public vs. Private Access Specifiers
C++ classes use access specifiers to control the visibility of their members. The two main access specifiers are:
- Public: Members declared as public can be accessed from outside the class.
- Private: Members declared as private can only be accessed within the class.
By default, all members of a class are private. To make members public, you need to use the public:
access specifier:
class Player {
public:
int x;
int y;
int speed;
void move(int xa, int ya);
private:
int health;
};
In this example, x
, y
, speed
, and move()
are public, while health
is private.
Member Functions (Methods)
Member functions, also known as methods, are functions that are part of a class. They can access and modify the class's data members. Member functions can be defined inside or outside the class declaration.
Defining Methods Inside the Class
class Player {
public:
int x, y, speed;
void move(int xa, int ya) {
x += xa * speed;
y += ya * speed;
}
};
Defining Methods Outside the Class
class Player {
public:
int x, y, speed;
void move(int xa, int ya);
};
void Player::move(int xa, int ya) {
x += xa * speed;
y += ya * speed;
}
When defining methods outside the class, you need to use the scope resolution operator (::) to specify which class the method belongs to.
Constructors and Destructors
Constructors and destructors are special member functions in C++ classes.
Constructors
Constructors are called when an object is created. They initialize the object's data members and allocate any necessary resources. Constructors have the same name as the class and don't have a return type.
class Player {
public:
int x, y, speed;
Player() : x(0), y(0), speed(1) {}
Player(int startX, int startY, int startSpeed) : x(startX), y(startY), speed(startSpeed) {}
};
In this example, we've defined two constructors: a default constructor and a parameterized constructor.
Destructors
Destructors are called when an object is destroyed. They clean up any resources allocated by the object. Destructors have the same name as the class preceded by a tilde (~) and don't take any parameters.
class Player {
public:
Player();
~Player() {
// Cleanup code here
}
};
Inheritance in C++ Classes
Inheritance is a fundamental concept in object-oriented programming that allows you to create new classes based on existing classes. The new class (derived class) inherits properties and methods from the existing class (base class).
class Character {
public:
int health;
void takeDamage(int amount);
};
class Player : public Character {
public:
int score;
void increaseScore(int points);
};
In this example, Player
is derived from Character
and inherits its health
member and takeDamage()
method.
Polymorphism in C++ Classes
Polymorphism allows objects of different classes to be treated as objects of a common base class. This is typically achieved through virtual functions and function overriding.
class Shape {
public:
virtual double area() = 0;
};
class Circle : public Shape {
public:
double radius;
double area() override {
return 3.14159 * radius * radius;
}
};
class Rectangle : public Shape {
public:
double width, height;
double area() override {
return width * height;
}
};
In this example, Circle
and Rectangle
both override the area()
function from the Shape
base class.
Friend Functions and Classes
Friend functions and classes are granted access to private and protected members of a class.
class Player {
private:
int health;
public:
friend void healPlayer(Player& p);
};
void healPlayer(Player& p) {
p.health += 10; // Can access private member
}
In this example, healPlayer()
is a friend function of the Player
class and can access its private members.
Static Members in C++ Classes
Static members belong to the class rather than to any specific object of the class.
class Player {
public:
static int playerCount;
Player() { playerCount++; }
~Player() { playerCount--; }
};
int Player::playerCount = 0;
In this example, playerCount
is a static member that keeps track of the number of Player
objects created.
Operator Overloading in C++ Classes
Operator overloading allows you to define how operators work with objects of your class.
class Vector2D {
public:
double x, y;
Vector2D operator+(const Vector2D& other) {
return Vector2D{x + other.x, y + other.y};
}
};
This example overloads the +
operator for the Vector2D
class.
Templates and Classes
Templates allow you to write generic classes that can work with different data types.
template<typename T>
class Stack {
private:
vector<T> elements;
public:
void push(T const& elem) { elements.push_back(elem); }
T pop() {
T top = elements.back();
elements.pop_back();
return top;
}
};
This Stack
class can be used with any data type.
Exception Handling in C++ Classes
Exception handling allows you to deal with runtime errors gracefully.
class DivideByZeroException {};
class Calculator {
public:
double divide(double a, double b) {
if (b == 0) throw DivideByZeroException();
return a / b;
}
};
try {
Calculator calc;
double result = calc.divide(10, 0);
} catch (DivideByZeroException&) {
cout << "Error: Division by zero" << endl;
}
This example demonstrates how to throw and catch exceptions in a class context.
Best Practices for C++ Classes
-
Use meaningful names: Choose clear, descriptive names for your classes, methods, and members.
-
Keep classes focused: Each class should have a single, well-defined purpose.
-
Use access specifiers appropriately: Make members private unless they need to be accessed externally.
-
Use const correctness: Mark methods that don't modify the object's state as const.
-
Consider using the Rule of Three/Five: If you define a destructor, copy constructor, or copy assignment operator, you should probably define all three (or five, including move constructor and move assignment operator in C++11 and later).
-
Use smart pointers: When dealing with dynamic memory, prefer smart pointers over raw pointers to prevent memory leaks.
-
Avoid circular dependencies: Be cautious when creating classes that depend on each other to prevent compilation issues.
Conclusion
C++ classes are a powerful feature that forms the backbone of object-oriented programming in C++. They provide a way to organize code, encapsulate data, and create reusable components. By mastering C++ classes, you'll be able to write more efficient, maintainable, and scalable code.
Remember that while classes offer many benefits, they're not always necessary for every programming task. Use them judiciously where they provide clear advantages in terms of code organization and reusability.
As you continue your journey in C++ programming, you'll discover more advanced features and techniques related to classes. Keep practicing, experimenting, and building projects to solidify your understanding of these concepts. Happy coding!
Article created from: https://youtu.be/2BP8NhxjrO0