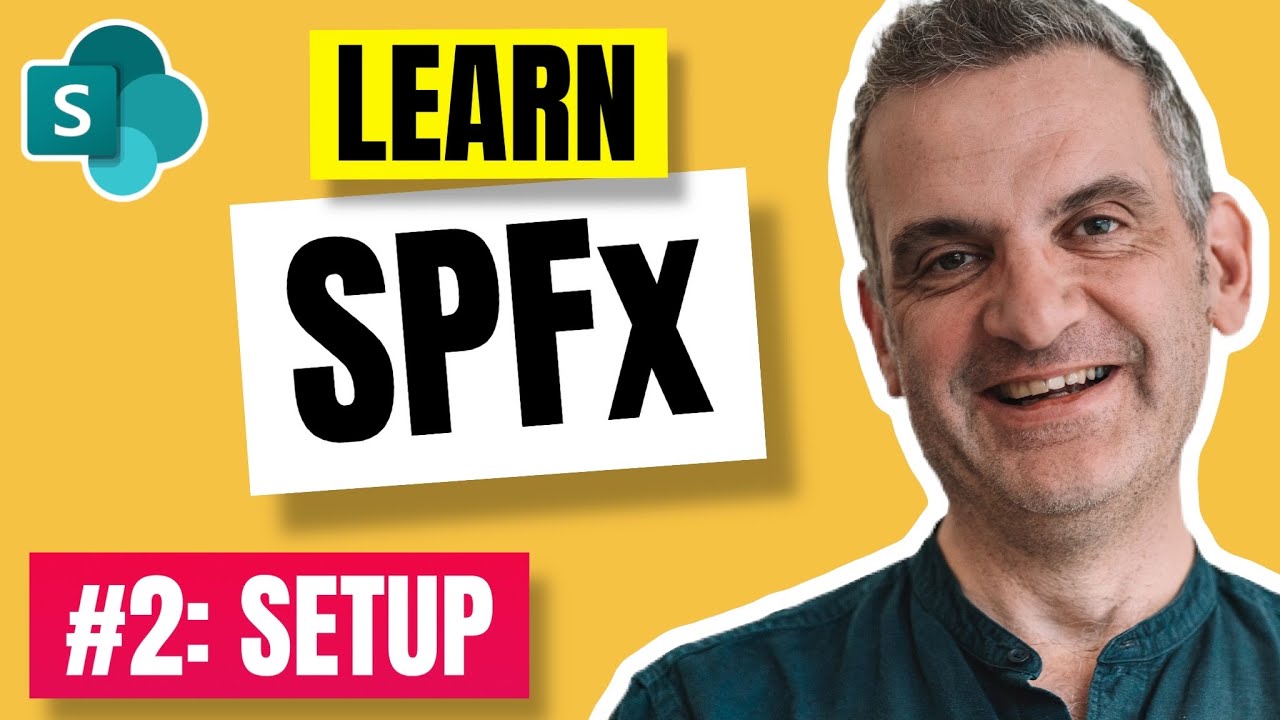
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to SharePoint Framework Development Tools
Developing for the SharePoint Framework (SPFx) requires a specific set of tools and a proper development environment setup. This comprehensive guide will walk you through the essential tools needed and the process of creating your first SharePoint Framework web part.
Key Tools for SharePoint Framework Development
Before diving into the development process, it's crucial to understand and install the necessary tools. Here's a breakdown of the primary tools required for SPFx development:
Visual Studio Code
Microsoft's Visual Studio Code is the recommended code editor for SharePoint Framework development. It's a lightweight yet powerful editor that provides excellent support for JavaScript, TypeScript, and other web technologies.
Installation steps:
- Visit the Visual Studio Code download page
- Select the appropriate package for your operating system (Windows or macOS)
- Download and run the installer
Node.js
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine. It's essential for running SharePoint Framework projects and managing package dependencies.
Installation steps:
- Go to the Node.js website
- Download version 10 (or the latest version compatible with SharePoint Framework)
- Run the installer for your operating system
npm (Node Package Manager)
npm comes bundled with Node.js and is used to install and manage package dependencies for your SharePoint Framework projects.
Gulp
Gulp is a toolkit used to automate tasks in your development workflow. In SharePoint Framework, it's used for building projects, packaging solutions, and running development servers.
Installation: After installing Node.js and npm, you can install Gulp globally using the following command:
npm install gulp-cli -g
Yeoman
Yeoman is a scaffolding tool that generates the initial project structure and files for your SharePoint Framework solutions.
Installation: Install Yeoman and the SharePoint Framework generators using npm:
npm install -g yo @microsoft/generator-sharepoint
Modern Web Browser
A modern web browser is necessary for running and testing your SharePoint Framework projects. Microsoft Edge, Google Chrome, or Mozilla Firefox are recommended.
Creating Your First SharePoint Framework Web Part
Now that we have all the necessary tools installed, let's walk through the process of creating your first SharePoint Framework web part.
Step 1: Create a New Project
-
Open your command line interface (CLI)
-
Navigate to the directory where you want to create your project
-
Run the following command:
yo @microsoft/sharepoint
-
Follow the prompts:
- Choose a solution name (e.g., "MyFirstWebPart")
- Select "SharePoint Online only (latest)" when asked which baseline packages you'd like to target
- Choose whether to use the current folder or create a new folder
- Select "WebPart" as the client-side component type
- Name your web part (e.g., "HelloWorld")
- Choose "No JavaScript framework" for this example
Step 2: Trust the Developer Certificate
Run the following command to trust the developer certificate:
gulp trust-dev-cert
Answer "yes" to any prompts that appear.
Step 3: Serve Your Web Part
To run your web part in the development environment, use the following command:
gulp serve
This will start a local web server and open your default browser to the SharePoint Workbench, where you can test your web part.
Understanding the Generated Code
After creating your first web part, it's important to understand the structure and files generated by Yeoman. Let's break down the key components:
Project Structure
MyFirstWebPart/
├── config/
├── node_modules/
├── src/
│ └── webparts/
│ └── helloWorld/
│ ├── HelloWorldWebPart.ts
│ ├── HelloWorldWebPart.manifest.json
│ └── loc/
├── .gitignore
├── .yo-rc.json
├── gulpfile.js
├── package.json
├── tsconfig.json
└── README.md
Key Files
- HelloWorldWebPart.ts: This is the main TypeScript file for your web part. It contains the logic and rendering code.
- HelloWorldWebPart.manifest.json: This file defines the metadata for your web part, such as its ID, version, and configurable properties.
- package.json: This file lists all the dependencies for your project and defines various scripts for building and running your web part.
- tsconfig.json: This file configures the TypeScript compiler options for your project.
- gulpfile.js: This file defines the Gulp tasks used to build and serve your project.
Customizing Your Web Part
Now that you have a basic understanding of the project structure, let's make some simple customizations to your web part.
Modifying the Render Method
Open the HelloWorldWebPart.ts
file and locate the render()
method. This method is responsible for rendering the HTML content of your web part. Let's modify it to display a custom message:
public render(): void {
this.domElement.innerHTML = `
<div class="${styles.helloWorld}">
<div class="${styles.container}">
<div class="${styles.row}">
<div class="${styles.column}">
<span class="${styles.title}">Welcome to SharePoint!</span>
<p class="${styles.subTitle}">This is my first custom web part.</p>
<p class="${styles.description}">${escape(this.properties.description)}</p>
<a href="https://aka.ms/spfx" class="${styles.button}">
<span class="${styles.label}">Learn more</span>
</a>
</div>
</div>
</div>
</div>`;
}
Adding Configurable Properties
You can add configurable properties to your web part to allow users to customize its behavior. In the HelloWorldWebPart.manifest.json
file, you can define these properties:
{
// ...
"preconfiguredEntries": [{
// ...
"properties": {
"description": "Hello World",
"test": "Multi-line text field",
"test1": true,
"test2": "2",
"test3": true
}
}]
}
Then, in your HelloWorldWebPart.ts
file, you can define these properties in the IHelloWorldWebPartProps
interface:
export interface IHelloWorldWebPartProps {
description: string;
test: string;
test1: boolean;
test2: string;
test3: boolean;
}
Building and Deploying Your Web Part
Once you've made your customizations, you'll want to build and deploy your web part to SharePoint.
Building Your Web Part
To build your web part, run the following command:
gulp build
This will compile your TypeScript code, bundle your assets, and create the necessary files for deployment.
Packaging Your Web Part
To create a package that can be deployed to SharePoint, use the following command:
gulp bundle --ship
gulp package-solution --ship
This will create a .sppkg
file in the sharepoint/solution
folder of your project.
Deploying to SharePoint
To deploy your web part to SharePoint:
- Go to your SharePoint App Catalog
- Upload the
.sppkg
file - Click "Deploy"
Your web part will now be available to add to SharePoint pages.
Best Practices for SharePoint Framework Development
As you continue developing with the SharePoint Framework, keep these best practices in mind:
-
Use TypeScript: TypeScript provides strong typing and better tooling support, which can help catch errors early in the development process.
-
Leverage React for Complex UIs: While we started with a no-framework approach, React is highly recommended for building complex user interfaces in SharePoint Framework.
-
Implement Error Handling: Always include proper error handling in your web parts to provide a better user experience and easier debugging.
-
Optimize Performance: Be mindful of performance, especially when making API calls or rendering large amounts of data.
-
Follow SharePoint Design Principles: Adhere to SharePoint's design principles to ensure your web parts integrate seamlessly with the SharePoint environment.
-
Use PnP Libraries: The PnP (Patterns and Practices) community provides several useful libraries that can simplify SharePoint development.
-
Keep Dependencies Updated: Regularly update your project dependencies to ensure you have the latest features and security patches.
-
Write Unit Tests: Implement unit tests for your web parts to ensure reliability and ease of maintenance.
Troubleshooting Common Issues
During SharePoint Framework development, you may encounter some common issues. Here are some troubleshooting tips:
-
Node Version Conflicts: Ensure you're using a compatible version of Node.js. If you need to switch between versions, consider using a Node version manager like nvm.
-
Build Errors: If you encounter build errors, try deleting the
node_modules
folder and runningnpm install
again to refresh your dependencies. -
Workbench Issues: If your web part isn't appearing in the workbench, check the browser console for any error messages.
-
CORS Errors: When making API calls, you may encounter CORS (Cross-Origin Resource Sharing) errors. Ensure your API endpoints are properly configured to allow requests from your development environment.
-
TypeScript Errors: If you're seeing TypeScript compilation errors, double-check your
tsconfig.json
file and ensure all necessary types are installed.
Conclusion
Developing for the SharePoint Framework opens up a world of possibilities for creating custom solutions that integrate seamlessly with SharePoint. By following this guide, you've taken the first steps in setting up your development environment, creating your first web part, and understanding the basics of SPFx development.
Remember, SharePoint Framework development is an ongoing learning process. As you continue to build more complex solutions, you'll discover new techniques, best practices, and ways to optimize your code. Stay curious, keep experimenting, and don't hesitate to engage with the SharePoint development community for support and inspiration.
With the foundation you've built here, you're well-equipped to start exploring more advanced topics in SharePoint Framework development, such as working with the SharePoint REST API, implementing custom property panes, and leveraging React for building sophisticated user interfaces.
Happy coding, and may your SharePoint development journey be filled with exciting challenges and rewarding solutions!
Article created from: https://youtu.be/k-z9BhaiBrA?si=OULCliSxxjUV7ZMr