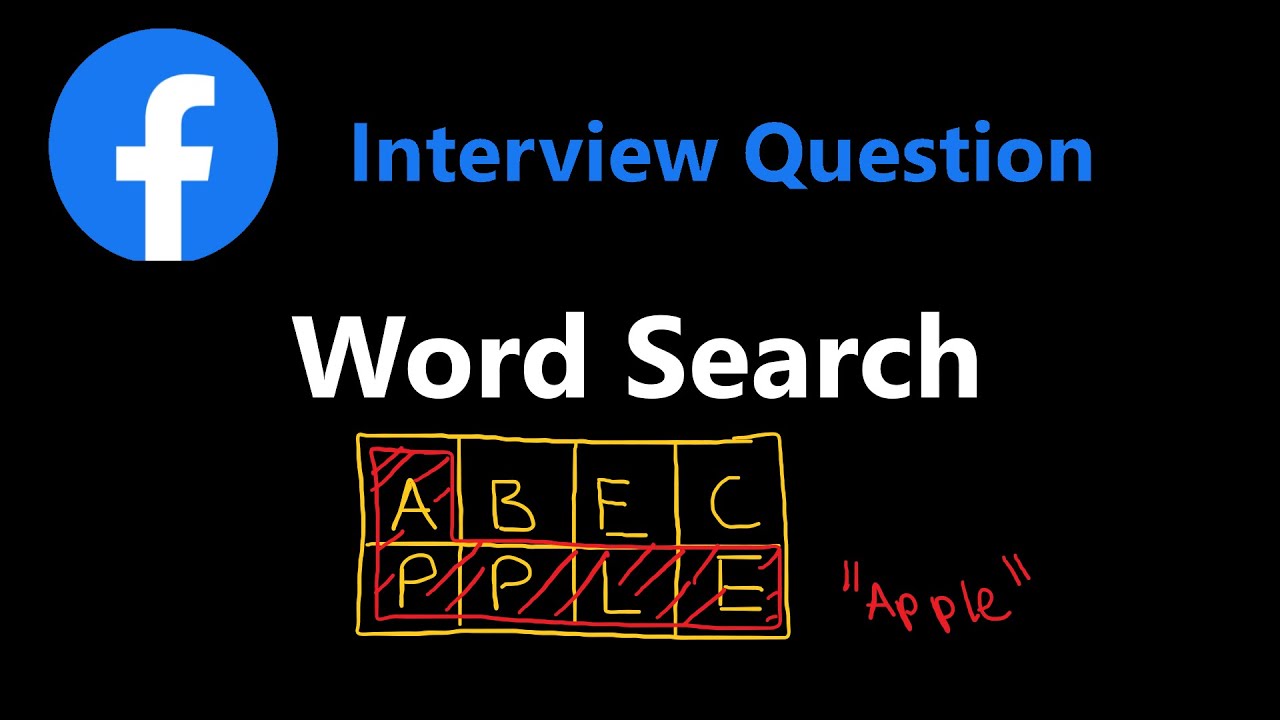
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Word Search Problem Solving
In the realm of coding problems, word search stands out as both a common and intriguing challenge. Presented with a grid or board filled with characters, the task involves searching for a target word within this board. The twist? The word must be constructed from horizontally or vertically adjacent cells, making the solution far from straightforward.
The Basics of the Problem
Imagine a grid, an array of characters arranged in rows and columns. Your goal is to find a specific word within this grid. If you can trace the word by moving through adjacent cells (either horizontally or vertically), you return true, signaling the word's presence. Otherwise, you return false. This setup provides a fascinating puzzle, as the word can take a winding path through the grid.
The Challenge of Efficiency
One might wonder if there's an efficient algorithm to crack this nut. The short answer is no. The solution to the word search problem relies on a brute-force approach, utilizing backtracking to explore possible paths for the target word.
Diving into Backtracking
Backtracking is essentially a methodical trial-and-error tactic. It involves exploring all potential paths in the grid to find the target word, making it an intuitive yet time-consuming solution. Here's how it unfolds in practice:
-
Start at every cell: Begin your search from each cell in the grid. If the starting character matches the first letter of your target word, you're off to a promising start.
-
Explore neighboring cells: Once you've found a matching starting letter, check the adjacent cells for the next character in your target word. This step is repeated, moving through the grid and checking each neighboring cell.
-
Avoid revisiting cells: To ensure you're not tracing the same path twice, employ a data structure (like a set) to track visited positions within your path.
-
Recursive Depth-First Search (DFS): Implement a nested DFS function to recursively explore each potential path. This function will take into account various conditions, such as remaining within the grid's bounds and ensuring characters match the target word sequentially.
Implementing the Solution
The backtracking solution involves creating a nested depth-for-search function that recursively searches through the grid. By passing the current position and the index of the character being searched, the function can methodically explore all possible paths.
Key Steps in the Algorithm:
-
Obtain the grid's dimensions and initialize a set to track the path, preventing the reuse of characters.
-
Create a depth-for-search function that:
- Returns true if the entire word is found.
- Checks for out-of-bounds scenarios or incorrect characters.
- Adds the current position to the path if the correct character is found, then continues the search.
-
After exploring all directions, remove the current position from the path set before returning to ensure cleanliness and correctness.
-
Apply this function to every cell in the grid, searching for the target word from each possible starting point.
The Complexity of the Solution
While backtracking offers a straightforward approach to solving the word search problem, it's not without its drawbacks, mainly efficiency. The time complexity stems from having to explore each cell in the grid, combined with the depth of the recursive search, leading to a complexity of approximately 4 to the power of the length of the word.
Conclusion
Solving the word search problem with backtracking might not be the most efficient method, but it's a powerful demonstration of how recursive depth-first search can tackle complex problems. Through understanding and applying this technique, one can navigate through grids to uncover hidden words, honing problem-solving skills in the process.
If you found this guide helpful and are interested in more coding insights, don't forget to like, subscribe, and stay tuned for future tutorials.