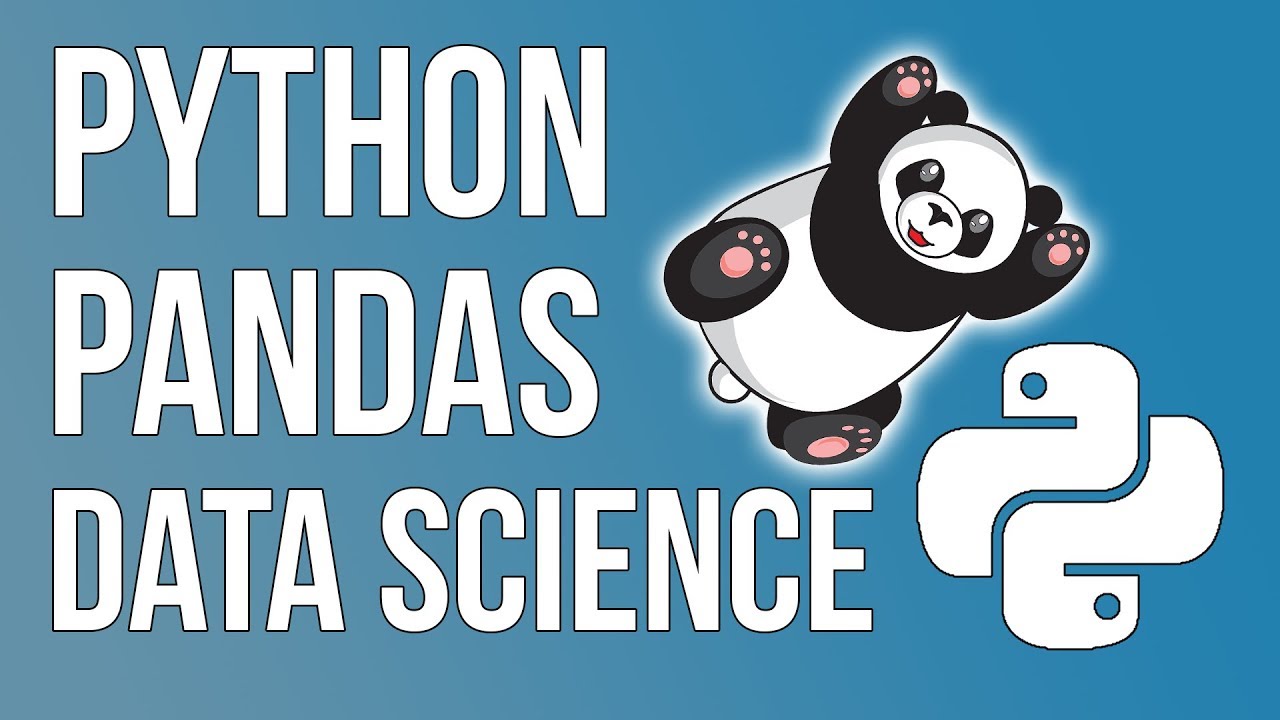
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Pandas
Pandas is one of the most powerful and widely used libraries for data analysis in Python. It provides high-performance, easy-to-use data structures and data analysis tools. In this comprehensive guide, we'll explore how to use Pandas effectively for various data manipulation and analysis tasks.
Installing Pandas
Before we begin, make sure you have Pandas installed. You can install it using pip:
pip install pandas
Loading Data into Pandas
One of the first steps in any data analysis project is loading your data. Pandas makes this process straightforward with functions to read various file formats.
Reading CSV Files
To read a CSV file, use the read_csv()
function:
import pandas as pd
df = pd.read_csv('pokemon_data.csv')
Reading Excel Files
For Excel files, use read_excel()
:
df_excel = pd.read_excel('pokemon_data.xlsx')
Reading Tab-Separated Files
For tab-separated files, you can still use read_csv()
but specify the delimiter:
df_tsv = pd.read_csv('pokemon_data.txt', delimiter='\t')
Exploring Your Data
Once you've loaded your data, it's time to explore it. Pandas provides several useful functions for this purpose.
Viewing the First Few Rows
Use the head()
function to view the first few rows of your DataFrame:
print(df.head())
Viewing the Last Few Rows
Similarly, use tail()
to view the last few rows:
print(df.tail())
Getting Column Names
To see the names of all columns in your DataFrame:
print(df.columns)
Basic Information About Your DataFrame
The info()
method provides a concise summary of your DataFrame:
df.info()
Statistical Summary of Numerical Columns
Use describe()
to get statistical information about your numerical columns:
print(df.describe())
Selecting Data
Pandas offers multiple ways to select specific data from your DataFrame.
Selecting Columns
To select a single column:
print(df['Name'])
To select multiple columns:
print(df[['Name', 'Type 1', 'HP']])
Selecting Rows
Use iloc[]
for integer-location based indexing:
print(df.iloc[0]) # First row
print(df.iloc[1:4]) # Rows 1 to 3
Use loc[]
for label-based indexing:
print(df.loc[df['Type 1'] == 'Fire'])
Filtering Data
Filtering allows you to select rows based on certain conditions.
Simple Filtering
fire_pokemon = df[df['Type 1'] == 'Fire']
print(fire_pokemon)
Multiple Conditions
Use &
for AND, |
for OR:
fire_or_water = df[(df['Type 1'] == 'Fire') | (df['Type 1'] == 'Water')]
print(fire_or_water)
Using isin()
To check if values are in a list:
starter_types = ['Grass', 'Fire', 'Water']
starters = df[df['Type 1'].isin(starter_types)]
print(starters)
Sorting Data
Pandas makes it easy to sort your data based on one or more columns.
Sorting by a Single Column
df_sorted = df.sort_values('Name')
print(df_sorted.head())
Sorting by Multiple Columns
df_multi_sort = df.sort_values(['Type 1', 'HP'], ascending=[True, False])
print(df_multi_sort.head())
Adding and Modifying Columns
You can easily add new columns or modify existing ones in your DataFrame.
Adding a New Column
df['Total'] = df['HP'] + df['Attack'] + df['Defense'] + df['Sp. Atk'] + df['Sp. Def'] + df['Speed']
print(df.head())
Modifying an Existing Column
df['HP'] = df['HP'] * 2
print(df.head())
Handling Missing Data
Missing data is a common issue in real-world datasets. Pandas provides several methods to handle this.
Checking for Missing Values
print(df.isnull().sum())
Dropping Rows with Missing Values
df_cleaned = df.dropna()
print(df_cleaned.shape)
Filling Missing Values
df_filled = df.fillna(0)
print(df_filled.head())
Grouping and Aggregating Data
Grouping allows you to split your data into groups based on some criteria and then perform operations on these groups.
Grouping by a Single Column
type_groups = df.groupby('Type 1')
print(type_groups['HP'].mean())
Grouping by Multiple Columns
type_legendary_groups = df.groupby(['Type 1', 'Legendary'])
print(type_legendary_groups['Attack'].mean())
Aggregating Multiple Columns
agg_results = df.groupby('Type 1').agg({
'HP': 'mean',
'Attack': 'max',
'Defense': 'min'
})
print(agg_results)
Merging and Joining DataFrames
Often, you'll need to combine data from multiple sources. Pandas provides several ways to do this.
Concatenating DataFrames
df1 = df.iloc[:400]
df2 = df.iloc[400:]
df_concat = pd.concat([df1, df2])
print(df_concat.shape)
Merging DataFrames
df_left = df[['Name', 'Type 1']]
df_right = df[['Name', 'Legendary']]
df_merged = pd.merge(df_left, df_right, on='Name')
print(df_merged.head())
Reshaping Data
Reshaping data is a common task in data analysis. Pandas provides functions like melt()
and pivot()
for this purpose.
Melting a DataFrame
df_melted = pd.melt(df, id_vars=['Name', 'Type 1'], value_vars=['HP', 'Attack', 'Defense'])
print(df_melted.head())
Pivoting a DataFrame
df_pivoted = df_melted.pivot(index='Name', columns='variable', values='value')
print(df_pivoted.head())
Working with Time Series Data
Pandas has excellent support for time series data.
Creating a DatetimeIndex
date_rng = pd.date_range(start='1/1/2022', end='12/31/2022', freq='D')
df_time = pd.DataFrame(date_rng, columns=['date'])
df_time['value'] = np.random.randn(len(date_rng))
print(df_time.head())
Resampling Time Series Data
df_time.set_index('date', inplace=True)
df_monthly = df_time.resample('M').mean()
print(df_monthly.head())
Handling Large Datasets
When working with large datasets that don't fit into memory, you can use chunking.
Reading Data in Chunks
chunk_size = 1000
chunks = []
for chunk in pd.read_csv('large_file.csv', chunksize=chunk_size):
# Process each chunk
chunks.append(chunk)
# Combine all chunks
df_large = pd.concat(chunks)
Exporting Data
After processing your data, you'll often want to save it for future use or sharing.
Saving to CSV
df.to_csv('processed_data.csv', index=False)
Saving to Excel
df.to_excel('processed_data.xlsx', index=False)
Saving to JSON
df.to_json('processed_data.json', orient='records')
Advanced Pandas Features
Using apply()
for Custom Operations
def double_hp(row):
return row['HP'] * 2
df['Double HP'] = df.apply(double_hp, axis=1)
print(df.head())
String Operations
Pandas provides vectorized string operations:
df['Name_Upper'] = df['Name'].str.upper()
print(df.head())
Categorical Data
Converting columns to categorical type can save memory and improve performance:
df['Type 1'] = df['Type 1'].astype('category')
print(df['Type 1'].dtype)
Conclusion
Pandas is an incredibly powerful library for data manipulation and analysis in Python. This guide has covered many of its key features, but there's always more to learn. As you work with different datasets and tackle various data analysis tasks, you'll discover even more ways that Pandas can make your work easier and more efficient.
Remember to consult the official Pandas documentation for more detailed information on these functions and to discover additional features. Happy data analyzing!
Article created from: https://www.youtube.com/watch?v=vmEHCJofslg