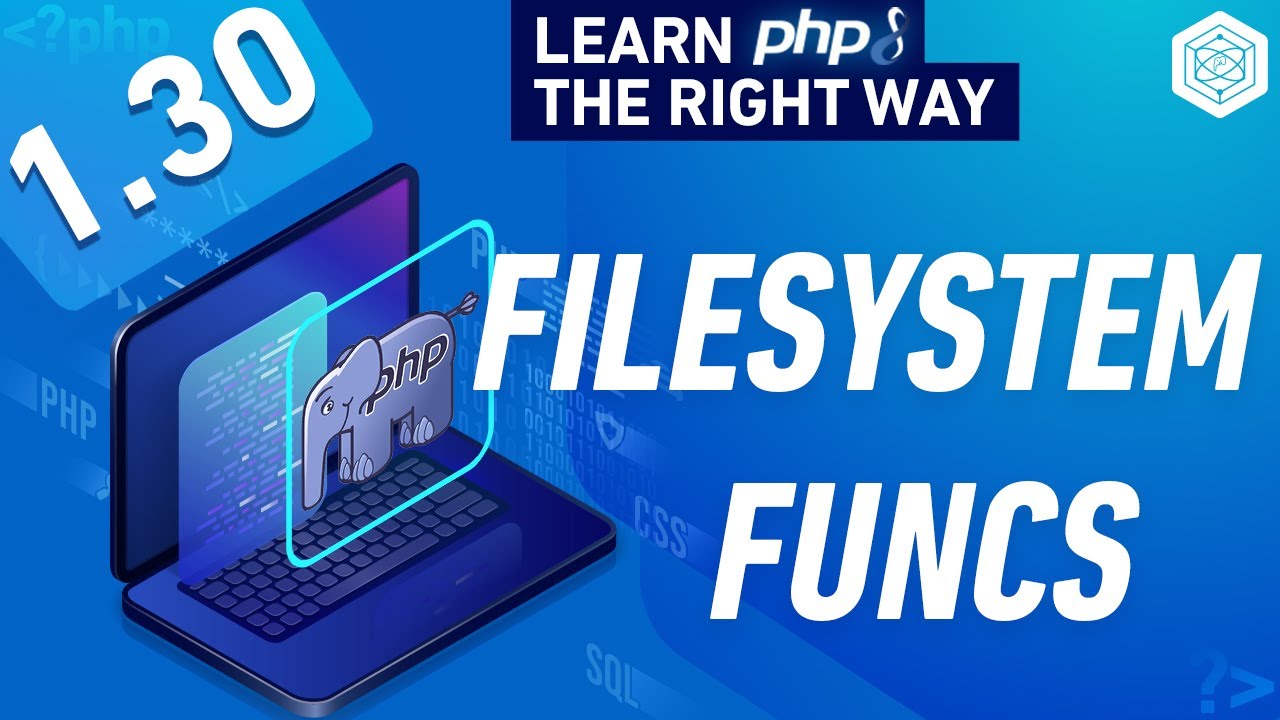
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeManaging files and directories is a fundamental aspect of PHP programming that every developer must master. PHP provides a wide range of built-in functions for file manipulation, enabling you to perform operations such as reading, writing, creating, and deleting files and directories. This guide will take you through the basics of file management in PHP, highlighting key functions and providing practical examples to help you understand how to work with files and directories efficiently.
Working with Directories
Listing Files and Directories
The scandir
function is your starting point for listing all files and directories within a given path. By using the magic constant to specify the current directory, scandir
returns an array of files and directories, which you can loop through to perform further operations. For example:
$dir = scandir('.');
var_dump($dir);
Creating and Deleting Directories
PHP allows you to create new directories using the mkdir
function and delete them with rmdir
. When creating directories, you can set permissions and decide whether to create directories recursively. Remember, rmdir
requires the directory to be empty before deletion:
mkdir('foo', 0777, true); // Creates a directory
rmdir('foo'); // Deletes the directory
File Operations
Checking File Existence and Size
Use the file_exists
function to check if a file or directory exists and the file_size
function to get the size of a file. PHP caches the return values of some file-related functions for performance, which is important to remember when working with file sizes:
if (file_exists('foo.txt')) {
echo file_size('foo.txt');
} else {
echo 'File not found';
}
Reading and Writing Files
Opening a file for reading or writing is done with fopen
, which returns a resource. You can read the file line by line using fgets
or work with CSV files using fgetcsv
. Writing to a file can be accomplished with fwrite
, or for simpler operations, file_put_contents
combines opening, writing, and closing a file into one step:
$file = fopen('foo.txt', 'r');
while (($line = fgets($file)) !== false) {
echo $line;
}
fclose($file);
Working with File Content
To get the entire content of a file into a variable, use file_get_contents
. This function also allows you to specify an offset and length to read specific parts of the file. Writing content back to a file is just as straightforward with file_put_contents
, which offers the option to append content instead of overwriting it:
$content = file_get_contents('foo.txt');
echo $content;
Copying, Renaming, and Deleting Files
Copying and moving files is handled by the copy
and rename
functions, respectively. To delete a file, use unlink
. These operations are essential for managing your file system effectively:
copy('foo.txt', 'bar.txt'); // Copies foo.txt to bar.txt
rename('foo.txt', 'baz.txt'); // Renames foo.txt to baz.txt
unlink('bar.txt'); // Deletes bar.txt
Retrieving File Information
The pathinfo
function provides a convenient way to get information about a file, such as its name, extension, and directory name. This is particularly useful for processing files based on their attributes:
$info = pathinfo('foo.txt');
print_r($info);
Conclusion
PHP's extensive set of file and directory management functions provides developers with the tools needed to perform complex file operations with ease. From basic tasks like creating and deleting directories to more advanced operations like reading, writing, and modifying file content, understanding these functions is crucial for any PHP developer. Remember to handle files with care, always check for existence before attempting to read or write, and manage resources efficiently to keep your applications running smoothly.
For more detailed examples and additional functions, check out the official PHP documentation.