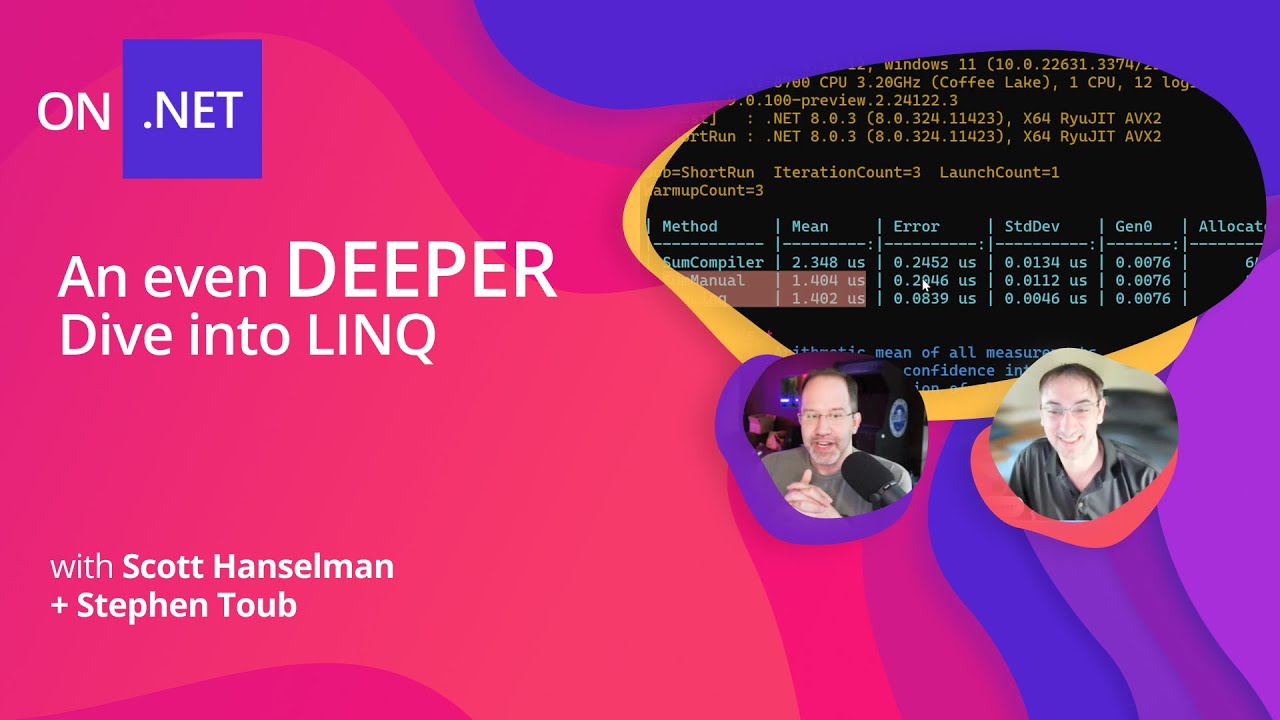
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction
LINQ (Language Integrated Query) is a powerful feature in .NET that allows developers to write expressive, declarative code for data manipulation and querying. While it might seem like magic at first, understanding how LINQ operates under the hood can significantly enhance your coding skills and performance optimizations. This article delves deep into LINQ, starting from creating basic iterators to exploring advanced optimizations like custom iterators for improved performance.
Crafting Custom Iterators
The journey begins with a simple exploration of creating custom iterators using the yield return
statement. This approach, while straightforward, illustrates the foundational principles of how LINQ queries are executed. It's shown that by manually implementing iterators, one can achieve performance nearly identical to compiler-generated code, demonstrating the efficiency of manual optimizations when done correctly.
Diving Into LINQ's Internals
A deeper dive reveals how LINQ itself is optimized for performance. A fascinating aspect of LINQ is its ability to specialize based on the type of data being operated on. For instance, iterating over an array or a list triggers specialized implementations (ArraySelectIterator
, ListSelectIterator
, etc.) that are significantly faster than the generic versions. This specialization is a testament to the thoughtful design of LINQ, aiming to provide the best possible performance across different scenarios.
Implementing Custom Optimizations
Moving beyond the basics, the discussion transitions to implementing custom optimizations. By examining how .NET ships with numerous custom iterators for different operations (e.g., Where
, Select
), it becomes clear that for frequently used patterns, such as Where
followed by Select
, .NET provides highly optimized paths. This leads to the creation of a WhereSelect
iterator that combines filtering and projection in a single, efficient loop, showcasing how in-depth knowledge of LINQ can lead to significant performance improvements.
Applying Optimizations in Real-World Scenarios
The article also addresses the practicalities of applying these optimizations in real-world coding scenarios. By understanding the internal workings of LINQ, developers can make informed decisions about the data structures and patterns they use, leading to more performant applications. For example, knowing that LINQ has specific optimizations for arrays and lists can guide developers in choosing the most appropriate data structure for their needs.
Conclusion
Mastering LINQ is not just about using its methods effectively but also understanding the intricacies of its implementation. This article demonstrates that with a deep understanding of LINQ's internal mechanisms, developers can write more efficient queries, utilize custom iterators for specific performance needs, and ultimately write better, faster .NET applications. As LINQ continues to evolve, staying informed about its internal optimizations and how to leverage them will remain a valuable skill for .NET developers.
For a more detailed exploration and examples, watch the full video here.