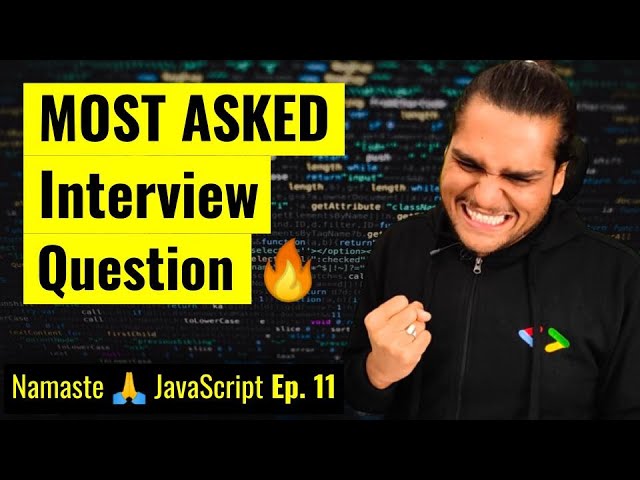
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeJavaScript is a language full of nuances and intricate behaviors that, if not fully understood, can turn coding into a hair-pulling experience, especially when dealing with closures. Closures are a frequent topic in interviews and a common source of confusion, but mastering them can significantly improve your coding skills and interview performance. This article will demystify closures through practical examples, illustrating their behavior in JavaScript and how to leverage them effectively in your code. Let's dive into the concept of closures, their quirks, and how to use them to your advantage in coding interviews and beyond.
Understanding Closures in JavaScript
At its core, a closure is a function bundled together with references to its surrounding state. In other words, a closure gives you access to an outer function’s scope from an inner function. This concept becomes particularly interesting and somewhat tricky when it involves asynchronous operations like setTimeout
.
Consider a simple example where we have a function x
and a variable i
set to 1. If we use setTimeout
to log the value of i
after a certain period, say 1000 milliseconds, the output is straightforward - it logs the value of i
, which is 1. This example illustrates the basic understanding of closures - the inner function (the callback of setTimeout
) remembers the value of i
from the outer scope.
However, when we introduce a loop to log values from 1 to 5 after each second, things get more complex. A common approach is to use a for loop with var i = 1; i <= 5; i++
and set a setTimeout
to log the value of i
multiplied by 1000 milliseconds. Surprisingly, this approach doesn't work as expected. Instead of logging the numbers 1 through 5 in sequence, it logs the number 6 five times!
The Problem Explained
The issue arises because of how JavaScript handles the var
keyword and closures. The var
keyword does not have block scope, and closures capture references, not values. So, all iterations of the loop share the same reference to i
, which by the end of the loop becomes 6. This shared reference is why all setTimeout
callbacks log 6 instead of the expected sequence.
Solving the Problem with let
The solution is surprisingly simple - use let
instead of var
. The let
keyword introduces block scope, meaning each iteration of the loop has its own i
, and each setTimeout
callback closes over a separate i
reference. This change correctly logs the numbers 1 through 5 in sequence after the respective delays.
A Closure-Based Solution
What if you're restricted from using let
? Closures come to the rescue again. By wrapping the setTimeout
in another function and passing i
as an argument, you create a new scope for each iteration. This approach forces each setTimeout
callback to close over a separate i
, even when using var
. This technique demonstrates the power and flexibility of closures in solving scope-related issues.
The Beauty of JavaScript Closures
Understanding closures is crucial for any JavaScript developer, not just for acing interviews but for writing efficient and bug-free code. Closures are a fundamental concept that, once mastered, reveal the elegance of JavaScript. They allow you to write more predictable and maintainable code, especially when dealing with asynchronous operations.
In interviews and practical coding, the ability to manipulate closures to your advantage can be a strong sign of your proficiency in JavaScript. It shows a deep understanding of the language's scope, execution context, and asynchronous nature. Moreover, it allows you to tackle common coding challenges with ease and elegance.
Conclusion
Closures in JavaScript are a powerful feature that, when understood and used correctly, can significantly enhance your coding and problem-solving skills. They are a common interview topic for a reason - understanding closures means understanding a core part of JavaScript. By mastering closures, you not only improve your chances in interviews but also become a better JavaScript developer. Remember, JavaScript, much like time and tide, waits for none. Embrace closures, and let them work for you, not against you.
For a deeper dive into closures and more JavaScript tips, check out the original video here.