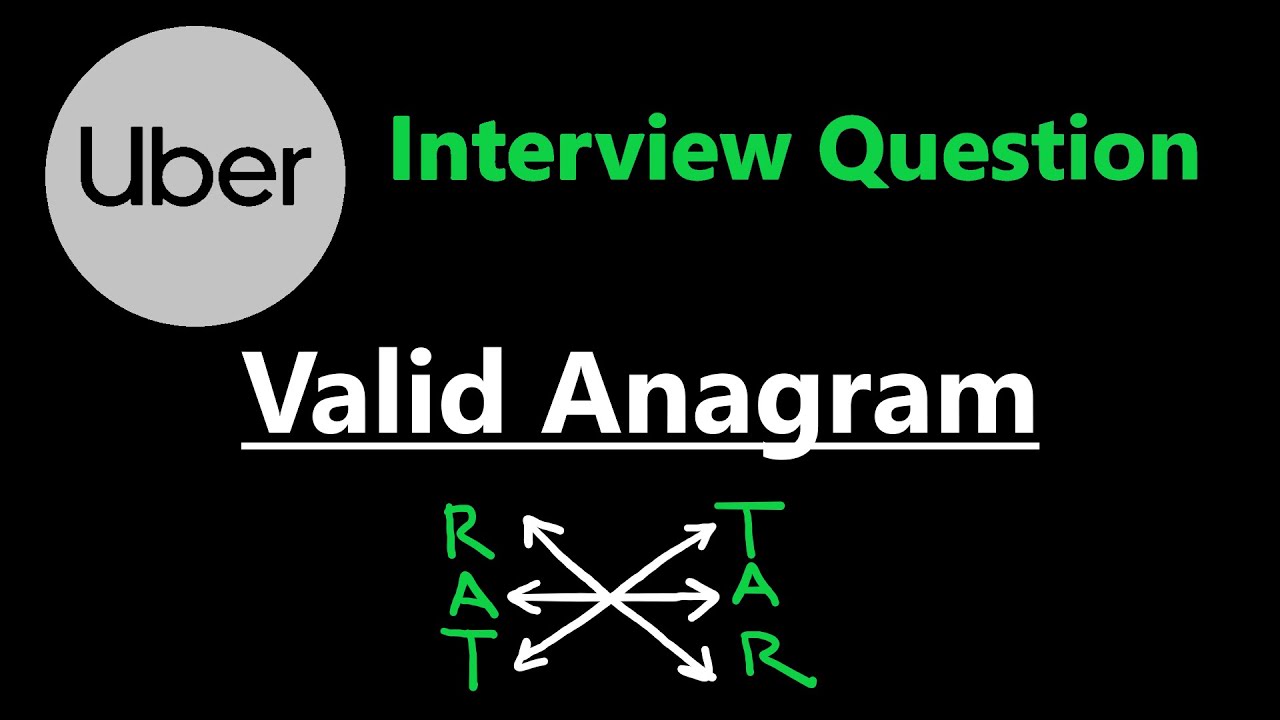
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIn the realm of coding challenges, determining whether two strings are anagrams of each other is a classic problem that tests a programmer's ability to manipulate and compare data structures. An anagram, by definition, involves rearranging the characters of one string to form another, implying that both strings must consist of the same characters in exactly the same quantity but possibly in a different order. This article delves into solving the 'Valid Anagram' problem using various techniques, each with its own set of advantages and computational considerations.
Understanding the Problem
The primary objective is to ascertain whether two given strings, s
and t
, are anagrams of each other. To affirm that t
is an anagram of s
, every character in s
must be present in t
in the exact quantity. This requirement extends beyond mere character matching to include a precise count match for each character.
For instance, for s = 'anagram'
and t = 'nagaram'
, a detailed examination reveals a perfect match in character occurrence, thus confirming their anagram status. Conversely, strings like rat
and car
, despite sharing some characters, fail the anagram test due to a mismatch in at least one character's occurrence.
Solution 1: Utilizing Hash Maps
The first and most intuitive solution involves counting the occurrences of each character in both strings. This can be efficiently achieved using hash maps. A hash map for each string is created where the key represents a character, and the value denotes the character's frequency. After populating these hash maps, a comparison of the character counts from both maps determines the anagram status.
This method, while straightforward, bears a time complexity of O(s + t) and a similar space complexity, given the construction of hash maps proportional to the sizes of s
and t
.
Python Implementation Snippet
if len(s) != len(t):
return False
# Creating hash maps for both strings
count_s, count_t = {}, {}
for char in s:
count_s[char] = count_s.get(char, 0) + 1
for char in t:
count_t[char] = count_t.get(char, 0) + 1
# Comparing hash maps
for char in count_s:
if count_s[char] != count_t.get(char, 0):
return False
return True
Solution 2: Sorting
A less memory-intensive approach involves sorting both strings and then comparing them directly. If both sorted strings match, they are anagrams; otherwise, they are not. This solution primarily hinges on the time complexity of the sorting algorithm used, typically O(n log n). However, it is crucial to discuss with the interviewer the assumptions regarding the space complexity of sorting, as it varies with the sorting algorithm and the programming language's implementation.
Python Implementation Snippet
return sorted(s) == sorted(t)
Conclusion
Solving the 'Valid Anagram' problem showcases the importance of algorithm selection based on the specific requirements of a problem, including time and space complexity considerations. While hash maps offer a straightforward solution, sorting presents a potentially more space-efficient alternative, especially in languages or scenarios where sorting does not require significant additional memory. Understanding these solutions not only aids in tackling similar problems but also enhances one's ability to choose the most appropriate algorithm for a given task.
For a more detailed explanation and code examples, watch the full video here.