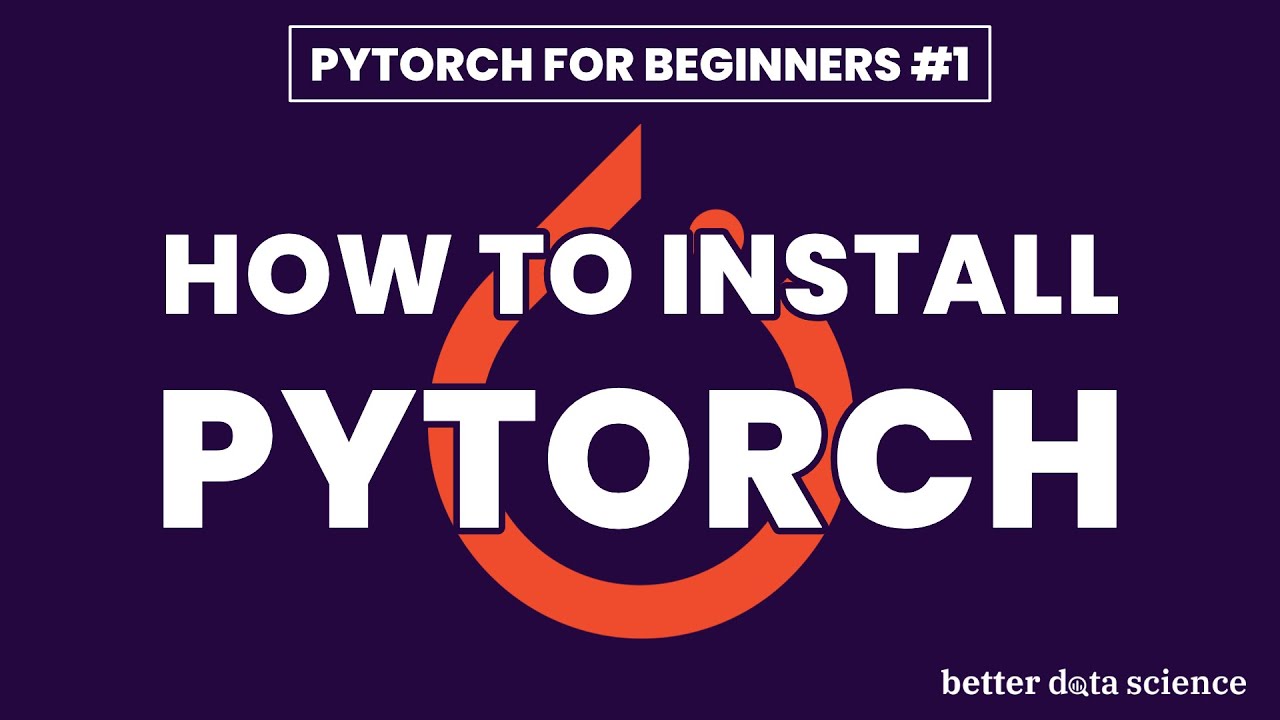
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to PyTorch Installation
PyTorch has become one of the most popular deep learning frameworks, known for its flexibility and ease of use. This guide will walk you through the process of installing PyTorch on your local system, with a focus on Apple Silicon support. Don't worry if you're using a different operating system - these installation steps are applicable across various platforms.
Finding the Correct Installation Command
The first step in installing PyTorch is to find the appropriate installation command for your system. Here's how to do it:
- Visit the official PyTorch website
- Select the options that match your operating system and Python environment
- Choose the PyTorch version you want to install (usually the latest stable version)
- Select your preferred package manager (e.g., pip, conda)
- Choose your compute platform (CUDA version or CPU)
For example, if you want to install the latest stable version of PyTorch on macOS using Anaconda for dependency management, you would select those options on the website. The PyTorch website will then automatically generate the installation command for you.
Best Practices: Using Virtual Environments
Before running the installation command, it's crucial to understand the importance of using virtual environments. Virtual environments allow you to create isolated Python environments for different projects, preventing conflicts between package versions and ensuring a clean, reproducible setup.
Creating a New Python Virtual Environment
If you're using Anaconda for dependency management, you can create a new virtual environment with the following steps:
-
Open your terminal or command prompt
-
Run the following command:
conda create -n pytorch_env python=3.x -y
Replace
3.x
with your desired Python version (e.g., 3.9, 3.10) -
Activate the newly created environment:
conda activate pytorch_env
You're now inside a new virtual environment, ready to install PyTorch and other necessary libraries.
Installing PyTorch
With your virtual environment activated, you can now install PyTorch using the command generated by the PyTorch website. Simply copy and paste this command into your terminal:
conda install pytorch torchvision torchaudio -c pytorch
Depending on your internet speed, the installation process might take a few minutes. You'll see various messages during the installation, indicating the progress and any additional dependencies being installed.
Installing Essential Data Science Libraries
While PyTorch is powerful on its own, you'll likely want to install some additional data science libraries to complement your deep learning workflow. Here are some essential libraries you might want to consider:
- Pandas: For data manipulation and analysis
- Jupyter: For interactive computing and creating notebooks
- Matplotlib: For creating static, animated, and interactive visualizations
- Scikit-learn: For machine learning algorithms and data preprocessing
You can install these libraries using the following command:
conda install pandas jupyter matplotlib scikit-learn
Feel free to add any other libraries you frequently use in your data science projects.
Verifying PyTorch Installation
After installing PyTorch and the additional libraries, it's important to verify that everything is working correctly. Here's how you can do that:
-
Launch Jupyter Lab by running
jupyter lab
in your terminal -
Create a new notebook
-
Run the following code to check the PyTorch version:
import torch print(torch.__version__)
If you see the version number printed without any errors, congratulations! You have successfully installed PyTorch.
MPS Acceleration for Apple Silicon
If you're using an Apple Silicon machine (M1, M2, etc.), you might be wondering about GPU acceleration. While CUDA is not available for these devices, Apple has introduced Metal Performance Shaders (MPS) to enable high-performance GPU training on macOS devices.
Checking MPS Availability
To verify if the MPS backend is available and if your PyTorch version was built with MPS support, run the following commands:
print(torch.backends.mps.is_available())
print(torch.backends.mps.is_built())
If both commands return True
, you have MPS support.
Testing MPS Acceleration
To truly test if MPS acceleration is working, you can run a simple learning process. Here's a code snippet that simulates a basic neural network training:
import torch
import math
device = torch.device("mps")
# Generate some data
x = torch.linspace(-math.pi, math.pi, 2000, device=device)
y = torch.sin(x)
# Randomly initialize weights
a = torch.randn((), device=device, requires_grad=True)
b = torch.randn((), device=device, requires_grad=True)
c = torch.randn((), device=device, requires_grad=True)
d = torch.randn((), device=device, requires_grad=True)
learning_rate = 5e-6
for t in range(2000):
# Forward pass
y_pred = a + b * x + c * x ** 2 + d * x ** 3
# Compute loss
loss = (y_pred - y).pow(2).sum()
if t % 100 == 0:
print(t, loss.item())
# Backward pass
loss.backward()
# Update weights
with torch.no_grad():
a -= learning_rate * a.grad
b -= learning_rate * b.grad
c -= learning_rate * c.grad
d -= learning_rate * d.grad
# Zero gradients
a.grad = None
b.grad = None
c.grad = None
d.grad = None
print(f'Result: y = {a.item()} + {b.item()} x + {c.item()} x^2 + {d.item()} x^3')
If this code runs without errors, it means you have PyTorch installed with MPS support, and it's utilizing your GPU for computations.
Troubleshooting Common Installation Issues
While the installation process is generally straightforward, you might encounter some issues. Here are some common problems and their solutions:
-
Incompatible Python versions: Ensure that your Python version is compatible with the PyTorch version you're trying to install. Check the PyTorch documentation for version compatibility.
-
Conda environment conflicts: If you're using Anaconda and experiencing conflicts, try creating a new environment with minimal packages and then install PyTorch.
-
CUDA version mismatch: For NVIDIA GPU users, make sure your CUDA version is compatible with the PyTorch version you're installing.
-
MPS not available: For Apple Silicon users, ensure you're running macOS 12.3 or later, as MPS support was introduced in this version.
-
Installation hanging: If the installation seems to hang, it might be downloading large files. Be patient, or check your internet connection.
Keeping PyTorch Updated
To ensure you're always using the latest features and bug fixes, it's important to keep PyTorch updated. Here's how you can update PyTorch:
-
Activate your PyTorch environment:
conda activate pytorch_env
-
Update PyTorch:
conda update pytorch torchvision torchaudio -c pytorch
It's a good practice to check for updates regularly, especially before starting new projects.
Exploring PyTorch Features
Now that you have PyTorch installed, you're ready to explore its powerful features. Here are some key areas to focus on:
Tensors
Tensors are the fundamental data structure in PyTorch. They're similar to NumPy arrays but can be used on GPUs for faster computations. Here's a quick example:
import torch
# Create a tensor
x = torch.tensor([1, 2, 3, 4, 5])
print(x)
# Perform operations
y = x * 2
print(y)
Autograd
Autograd is PyTorch's automatic differentiation engine. It allows you to automatically compute gradients, which is crucial for training neural networks:
import torch
x = torch.tensor(2.0, requires_grad=True)
y = x**2
y.backward()
print(x.grad) # Should print 4.0
Neural Network Modules
PyTorch provides a high-level API for building neural networks through its nn
module:
import torch
import torch.nn as nn
class SimpleNet(nn.Module):
def __init__(self):
super(SimpleNet, self).__init__()
self.fc1 = nn.Linear(10, 5)
self.fc2 = nn.Linear(5, 1)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
model = SimpleNet()
print(model)
DataLoaders
DataLoaders in PyTorch make it easy to handle large datasets and batch processing:
from torch.utils.data import Dataset, DataLoader
import numpy as np
class MyDataset(Dataset):
def __init__(self, size):
self.data = np.random.rand(size, 10)
self.labels = np.random.randint(0, 2, size)
def __len__(self):
return len(self.data)
def __getitem__(self, idx):
return torch.FloatTensor(self.data[idx]), torch.LongTensor([self.labels[idx]])
dataset = MyDataset(1000)
dataloader = DataLoader(dataset, batch_size=32, shuffle=True)
for batch_data, batch_labels in dataloader:
print(f"Batch data shape: {batch_data.shape}, Batch labels shape: {batch_labels.shape}")
break
Best Practices for PyTorch Development
As you start developing with PyTorch, keep these best practices in mind:
-
Use GPU acceleration when available: PyTorch makes it easy to move computations to GPU:
device = torch.device("cuda" if torch.cuda.is_available() else "cpu") model = model.to(device) data = data.to(device)
-
Utilize PyTorch's built-in datasets: PyTorch provides many popular datasets out of the box:
from torchvision.datasets import MNIST from torchvision.transforms import ToTensor train_dataset = MNIST(root='./data', train=True, download=True, transform=ToTensor())
-
Implement proper error handling: Wrap your training loops in try-except blocks to catch and handle errors gracefully.
-
Use learning rate schedulers: Adjust your learning rate during training for better convergence:
from torch.optim.lr_scheduler import StepLR scheduler = StepLR(optimizer, step_size=30, gamma=0.1)
-
Implement early stopping: Stop training when the validation loss stops improving to prevent overfitting.
-
Use model checkpointing: Save your model periodically during training:
torch.save(model.state_dict(), 'model_checkpoint.pth')
-
Utilize PyTorch's profiler: Use the built-in profiler to identify performance bottlenecks in your code.
Advanced PyTorch Topics
Once you're comfortable with the basics, you can explore more advanced PyTorch topics:
Custom Datasets and Data Augmentation
Create custom datasets and implement data augmentation techniques to improve model generalization:
from torchvision import transforms
transform = transforms.Compose([
transforms.RandomHorizontalFlip(),
transforms.RandomRotation(10),
transforms.ToTensor(),
transforms.Normalize((0.5,), (0.5,))
])
dataset = MyDataset(transform=transform)
Transfer Learning
Leverage pre-trained models for your specific tasks:
import torchvision.models as models
resnet = models.resnet50(pretrained=True)
for param in resnet.parameters():
param.requires_grad = False
resnet.fc = nn.Linear(resnet.fc.in_features, num_classes)
Custom Loss Functions
Implement custom loss functions for specific problems:
class CustomLoss(nn.Module):
def __init__(self):
super(CustomLoss, self).__init__()
def forward(self, outputs, targets):
return torch.mean((outputs - targets)**2)
criterion = CustomLoss()
Distributed Training
Utilize multiple GPUs or machines for faster training:
import torch.distributed as dist
import torch.multiprocessing as mp
from torch.nn.parallel import DistributedDataParallel
def train(rank, world_size):
dist.init_process_group("nccl", rank=rank, world_size=world_size)
model = DistributedDataParallel(model, device_ids=[rank])
# Training loop here
mp.spawn(train, args=(world_size,), nprocs=world_size, join=True)
Conclusion
Installing PyTorch is the first step in your journey to mastering deep learning. With PyTorch now set up on your system, you're ready to explore its vast capabilities, from building simple neural networks to implementing cutting-edge research papers.
Remember to keep practicing, experimenting with different models and datasets, and staying updated with the latest PyTorch developments. The PyTorch community is vast and supportive, so don't hesitate to seek help when you encounter challenges.
As you continue your PyTorch journey, you'll discover its flexibility in tackling various machine learning tasks, from computer vision to natural language processing and reinforcement learning. The skills you develop with PyTorch will be invaluable in your data science and machine learning career.
Happy coding, and may your models converge quickly!
Article created from: https://youtu.be/mS2X1QmIUCI?si=5inwUYJQdK6Ukq_v