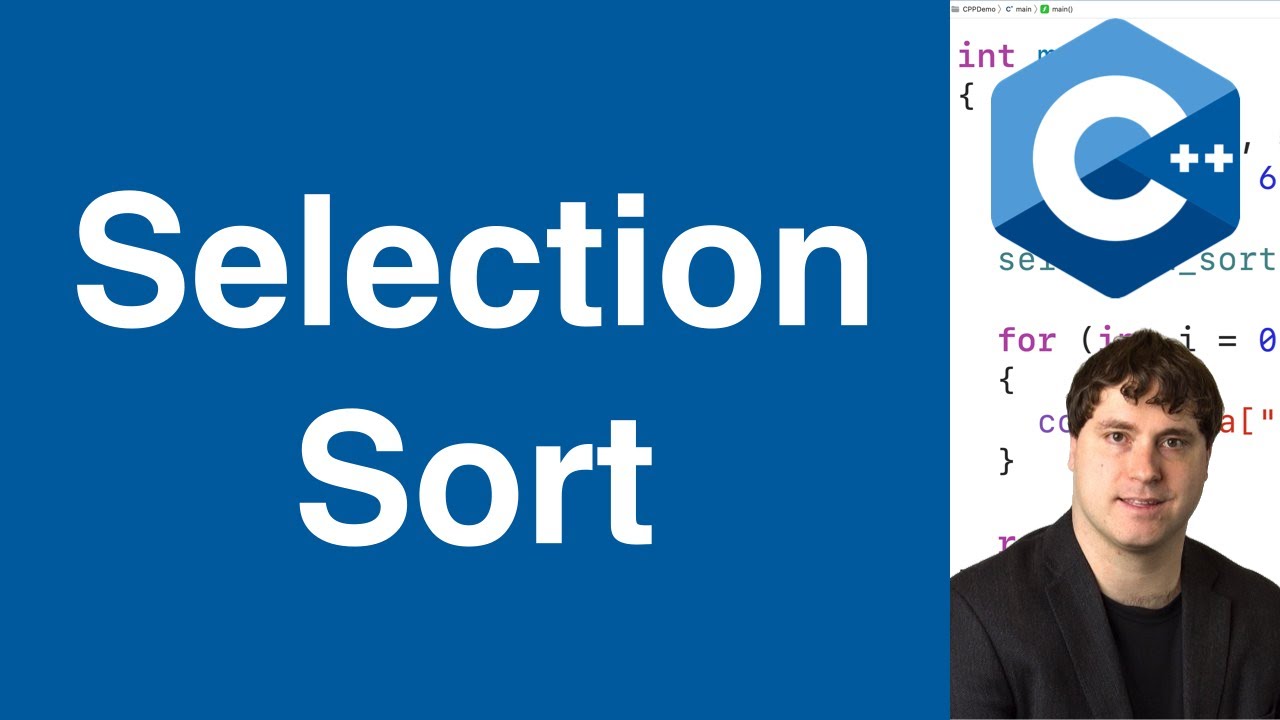
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeUnderstanding Selection Sort
Selection sort is a simple yet effective sorting algorithm that can be used to arrange elements in an array. While it may not be the most efficient for large datasets, understanding its implementation is crucial for budding programmers and those looking to grasp fundamental sorting concepts.
What is Selection Sort?
Selection sort is an in-place comparison sorting algorithm. It divides the input list into two parts:
- A sorted portion at the left end
- An unsorted portion at the right end
Initially, the sorted portion is empty, and the unsorted portion contains the entire list. The algorithm proceeds to find the smallest element in the unsorted portion and swaps it with the leftmost unsorted element, moving the boundary between the sorted and unsorted portions one element to the right.
How Selection Sort Works
Let's break down the process step-by-step:
- Start with an unsorted array
- Find the minimum element in the unsorted portion
- Swap it with the first element of the unsorted portion
- Move the boundary between sorted and unsorted portions one element to the right
- Repeat steps 2-4 until the entire array is sorted
Implementing Selection Sort in C++
Now that we understand the concept, let's implement the selection sort algorithm in C++. We'll create a function that takes an array and its length as parameters and sorts the array in ascending order.
Setting Up the Function
First, let's define our function:
void selectionSort(int arr[], int length) {
// Implementation will go here
}
This function takes two parameters:
-
arr[]
: The array to be sorted -
length
: The number of elements in the array
The Outer Loop
We'll start with an outer loop that iterates through the array:
for (int i = 0; i < length - 1; i++) {
// Find minimum element in unsorted portion
}
This loop runs from the first element to the second-to-last element. We don't need to consider the last element in the outer loop because it will automatically be in the correct position once all other elements are sorted.
Finding the Minimum Element
Inside the outer loop, we'll find the minimum element in the unsorted portion:
int minPosition = i;
for (int j = i + 1; j < length; j++) {
if (arr[j] < arr[minPosition]) {
minPosition = j;
}
}
Here, we assume the current element is the minimum and then compare it with all subsequent elements. If we find a smaller element, we update minPosition
.
Swapping Elements
After finding the minimum element, we swap it with the first unsorted element (if they're not already the same):
if (minPosition != i) {
int temp = arr[i];
arr[i] = arr[minPosition];
arr[minPosition] = temp;
}
Complete Implementation
Putting it all together, here's the complete implementation of the selection sort function:
void selectionSort(int arr[], int length) {
for (int i = 0; i < length - 1; i++) {
int minPosition = i;
for (int j = i + 1; j < length; j++) {
if (arr[j] < arr[minPosition]) {
minPosition = j;
}
}
if (minPosition != i) {
int temp = arr[i];
arr[i] = arr[minPosition];
arr[minPosition] = temp;
}
}
}
Testing the Implementation
To test our implementation, we'll create a main function that initializes an array, calls the selection sort function, and then prints the sorted array.
#include <iostream>
// ... selectionSort function here ...
int main() {
int arr[] = {6, 5, 3, 1, 4, 2};
int length = sizeof(arr) / sizeof(arr[0]);
std::cout << "Original array: ";
for (int i = 0; i < length; i++) {
std::cout << arr[i] << " ";
}
std::cout << std::endl;
selectionSort(arr, length);
std::cout << "Sorted array: ";
for (int i = 0; i < length; i++) {
std::cout << arr[i] << " ";
}
std::cout << std::endl;
return 0;
}
When you run this program, you should see the following output:
Original array: 6 5 3 1 4 2
Sorted array: 1 2 3 4 5 6
Time Complexity Analysis
Understanding the time complexity of selection sort is crucial for knowing when to use this algorithm.
Best, Worst, and Average Case
Selection sort has a time complexity of O(n^2) in all cases (best, worst, and average), where n is the number of elements in the array. This is because:
- The outer loop always runs n-1 times
- The inner loop runs n-1 times on the first iteration, n-2 times on the second iteration, and so on
This results in (n-1) + (n-2) + ... + 2 + 1 = n(n-1)/2 comparisons, which simplifies to O(n^2).
Space Complexity
Selection sort has a space complexity of O(1) because it only uses a constant amount of additional memory space regardless of the input size. It sorts the array in-place, meaning it doesn't require any extra arrays or data structures proportional to the input size.
Advantages and Disadvantages of Selection Sort
Like all algorithms, selection sort has its pros and cons.
Advantages
- Simplicity: Selection sort is one of the simplest sorting algorithms to understand and implement.
- In-place sorting: It doesn't require extra space and sorts the array within itself.
- Performs well on small lists: For small arrays or lists, selection sort can be efficient.
Disadvantages
- Inefficiency for large lists: With its O(n^2) time complexity, selection sort becomes very slow for large datasets.
- Not stable: Selection sort is not a stable sort, meaning it may change the relative order of equal elements.
- No early termination: Even if the array becomes sorted early in the process, the algorithm continues to run until completion.
When to Use Selection Sort
Despite its inefficiency for large datasets, there are scenarios where selection sort can be useful:
- Small datasets: When working with small arrays or lists, selection sort's simplicity can make it a good choice.
- Limited memory: In environments with very limited memory, selection sort's in-place nature can be advantageous.
- Educational purposes: Selection sort is excellent for teaching basic sorting concepts and algorithm analysis.
- Checking if an array is sorted: Selection sort can be modified to check if an array is already sorted in linear time.
Comparing Selection Sort with Other Sorting Algorithms
To put selection sort into perspective, let's briefly compare it with some other common sorting algorithms:
Bubble Sort
Both bubble sort and selection sort have a time complexity of O(n^2), but bubble sort generally performs more swaps. Selection sort performs better when writing to memory is a costly operation.
Insertion Sort
Insertion sort also has a worst-case and average time complexity of O(n^2), but it performs better on partially sorted arrays and has a best-case time complexity of O(n) for already sorted arrays.
Merge Sort
Merge sort has a time complexity of O(n log n) in all cases, making it much more efficient for large datasets. However, it requires O(n) additional space.
Quick Sort
Quick sort has an average time complexity of O(n log n) and sorts in-place, but its worst-case time complexity is O(n^2). It's generally faster than selection sort for large datasets.
Optimizing Selection Sort
While selection sort's basic structure limits major optimizations, there are a few tweaks that can improve its performance slightly:
-
Early termination check: Add a check to see if any swaps were made in the outer loop. If not, the array is already sorted, and the algorithm can terminate.
-
Bidirectional selection sort: This variant selects both the minimum and maximum elements in each pass, potentially reducing the number of passes by half.
-
Cache optimization: For large arrays, organize the code to maximize cache hits, which can improve performance on modern hardware.
Here's an example of how you might implement the early termination check:
void optimizedSelectionSort(int arr[], int length) {
bool swapped;
for (int i = 0; i < length - 1; i++) {
int minPosition = i;
swapped = false;
for (int j = i + 1; j < length; j++) {
if (arr[j] < arr[minPosition]) {
minPosition = j;
swapped = true;
}
}
if (!swapped) {
// Array is already sorted
break;
}
if (minPosition != i) {
int temp = arr[i];
arr[i] = arr[minPosition];
arr[minPosition] = temp;
}
}
}
Conclusion
Selection sort is a fundamental sorting algorithm that every programmer should understand. While it may not be the most efficient choice for large datasets, its simplicity makes it an excellent learning tool and a viable option for small lists or memory-constrained environments.
By implementing selection sort in C++, we've explored not just the algorithm itself, but also important programming concepts like array manipulation, nested loops, and basic algorithm analysis. This knowledge forms a solid foundation for understanding more complex sorting algorithms and data structures.
Remember, the key to mastering algorithms is practice and understanding their underlying principles. As you continue your programming journey, you'll encounter many more sorting algorithms, each with its own strengths and weaknesses. The insights gained from understanding selection sort will serve you well in comprehending and implementing these more advanced techniques.
Keep coding, keep learning, and most importantly, keep sorting!
Article created from: https://www.youtube.com/watch?v=Q-NXg6Tzyks