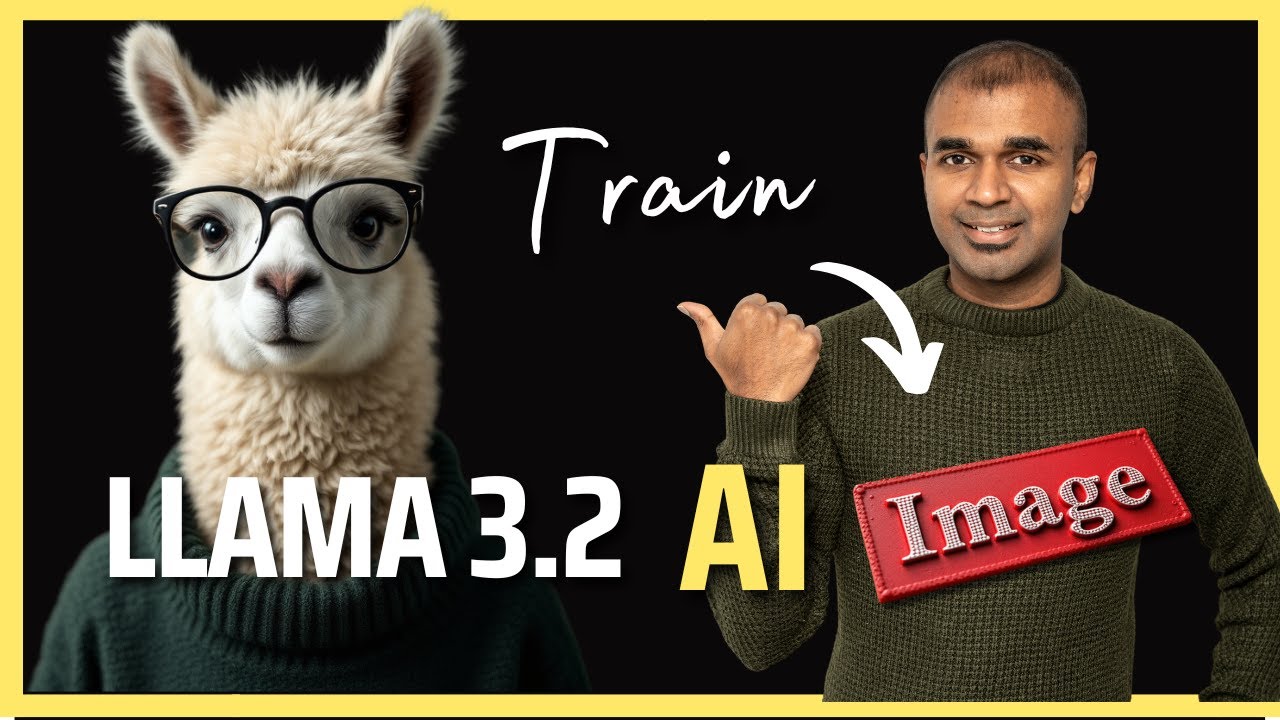
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeIntroduction to Fine-Tuning Llama 3.2 Vision Model
In the rapidly evolving field of artificial intelligence, the ability to analyze and interpret medical images accurately is becoming increasingly important. One of the most promising developments in this area is the fine-tuning of large language models for specific tasks, such as medical image analysis. This article will guide you through the process of fine-tuning the Llama 3.2 Vision model, an 11 billion parameter model developed by Meta, for improved performance in healthcare-related image analysis tasks.
Understanding the Llama 3.2 Vision Model
The Llama 3.2 Vision model is a powerful AI tool designed to analyze images and provide detailed descriptions based on visual input. When presented with an image and a question, the model can generate a response that describes what it "sees" in the image. This capability makes it particularly useful for applications in healthcare, where accurate interpretation of medical images is crucial.
Key Features of Llama 3.2 Vision Model:
- 11 billion parameters
- Developed by Meta
- Capable of analyzing and describing images
- Potential for significant impact in healthcare applications
The Need for Fine-Tuning
While the base Llama 3.2 Vision model is impressive, its responses to medical images may be too generic or lack the specific style and terminology preferred in medical contexts. Fine-tuning the model allows us to tailor its outputs to better suit the needs of healthcare professionals and improve its accuracy in interpreting medical images.
Benefits of Fine-Tuning:
- More accurate and relevant responses to medical images
- Improved understanding of medical terminology and concepts
- Better alignment with healthcare professionals' expectations
- Enhanced performance in specific medical imaging tasks
Preparing for Fine-Tuning
Before we begin the fine-tuning process, it's essential to set up the necessary environment and tools. Here's what you'll need:
Hardware Requirements:
- A powerful GPU (e.g., RTX A6000)
- Sufficient RAM and storage
Software Requirements:
- Python environment
- Unsloth library
- Hugging Face account and API token
Installation Steps:
-
Install the Unsloth library:
pip install unsloth
-
Export your Hugging Face token:
export HUGGING_FACE_HUB_TOKEN=your_token_here
-
Create a new Python file named
app.py
Loading the Model
The first step in our fine-tuning process is to load the pre-trained Llama 3.2 Vision model. We'll use the FastVisionModel class from the Unsloth library to accomplish this.
from unsloth import FastVisionModel
# Load the model
model = FastVisionModel.from_pretrained("meta-llama/Llama-2-11b-chat-hf")
This code snippet loads the 11 billion parameter Llama 2 chat model, which serves as the foundation for our fine-tuning process.
Configuring Fine-Tuning Parameters
Next, we need to specify which parts of the model we want to fine-tune. This allows us to focus on improving specific aspects of the model's performance without altering its entire structure.
# Configure fine-tuning parameters
peft_config = {
"r": 16,
"lora_alpha": 32,
"lora_dropout": 0.05,
"bias": "none",
"task_type": "CAUSAL_LM",
"target_modules": [
"q_proj",
"k_proj",
"v_proj",
"o_proj",
"gate_proj",
"up_proj",
"down_proj",
],
}
These parameters allow us to fine-tune specific layers, attention modules, and MLP modules within the model.
Loading the Dataset
For this fine-tuning task, we'll use a dataset of radiographic images with corresponding captions. This dataset, known as "Radiology mini," contains various types of X-rays and their descriptions.
from datasets import load_dataset
# Load the dataset
dataset = load_dataset("medical-imaging/radiology-mini")
This dataset will serve as our training data, teaching the model how to interpret and describe medical images accurately.
Preparing the Data
To prepare our data for fine-tuning, we need to combine the images, captions, and a specific instruction into a format that the model can understand.
def prepare_data(example):
instruction = "You are an expert radiographer. Describe accurately what you see in this image."
return {
"text": f"{instruction}\n\nImage: [image]\n\nHuman: What do you see in this image?\n\nAssistant: {example['caption']}",
"image": example['image']
}
converted_dataset = dataset.map(prepare_data)
This function creates a structured input for each image in our dataset, including an instruction, the image itself, and the corresponding caption.
Tokenization and Encoding
Before we can feed our data into the model, we need to convert the text and images into a format that the model can process. This involves tokenization and encoding.
from transformers import AutoTokenizer
tokenizer = AutoTokenizer.from_pretrained("meta-llama/Llama-2-11b-chat-hf")
def tokenize_function(examples):
return tokenizer(examples["text"], padding="max_length", truncation=True)
tokenized_dataset = converted_dataset.map(tokenize_function, batched=True)
This step converts our text data into numerical tokens that the model can understand.
Fine-Tuning Process
Now that we have prepared our data and model, we can begin the fine-tuning process. We'll use the SFTTrainer class from the Unsloth library to handle the training.
from unsloth import SFTTrainer, SFTConfig
trainer = SFTTrainer(
model=model,
tokenizer=tokenizer,
train_dataset=tokenized_dataset["train"],
dataset_text_field="text",
max_seq_length=512,
args=SFTConfig(
output_dir="./results",
num_train_epochs=3,
per_device_train_batch_size=4,
gradient_accumulation_steps=4,
learning_rate=2e-4,
fp16=True,
logging_steps=10,
save_strategy="epoch",
evaluation_strategy="epoch",
),
)
trainer.train()
This code sets up the training configuration and initiates the fine-tuning process. The model will be trained on our prepared dataset, gradually improving its ability to interpret and describe medical images.
Monitoring Training Progress
During the fine-tuning process, it's important to monitor the model's performance and resource usage. We can print various statistics to keep track of the training progress:
import torch
print(f"GPU Memory: {torch.cuda.max_memory_allocated() / 1e9:.02f} GB")
print(f"GPU Utilization: {torch.cuda.utilization()}%")
These metrics help us ensure that the training is progressing smoothly and that our hardware resources are being utilized effectively.
Evaluating the Fine-Tuned Model
After the fine-tuning process is complete, it's crucial to evaluate the model's performance to see how much it has improved. We can do this by comparing its responses to medical images before and after fine-tuning.
Before Fine-Tuning
Before fine-tuning, the model's responses to medical images might be generic or lack specific medical terminology. For example:
Human: What do you see in this image?
Assistant: The image appears to be an X-ray of a human skull. I can see the outline of the skull, including the cranium and jaw. There are also visible teeth in the lower part of the image. The X-ray seems to be capturing a side view or profile of the head.
While this description is not incorrect, it lacks the depth and specificity that a medical professional would expect.
After Fine-Tuning
After fine-tuning, the model's responses should be more accurate, detailed, and aligned with medical terminology. For example:
Human: What do you see in this image?
Assistant: The image is a panoramic radiograph of the oral and maxillofacial region. It shows:
1. Complete dentition: All teeth are visible, including third molars (wisdom teeth).
2. Mandible: The lower jaw bone is clearly defined, with a smooth cortical outline.
3. Maxilla: The upper jaw bone is visible, along with the maxillary sinuses.
4. Temporomandibular joints: Both TMJs are visible at the edges of the image.
5. Nasal cavity: The floor of the nasal cavity can be seen.
6. Cervical spine: A portion of the cervical vertebrae is visible.
7. Hyoid bone: The hyoid bone is faintly visible in the neck region.
8. No obvious pathology: There are no apparent fractures, lesions, or abnormal radiolucencies visible in this image.
The image quality is good, with appropriate contrast and clarity for diagnostic purposes. This panoramic radiograph provides a comprehensive view of the patient's oral and maxillofacial structures, which is useful for general dental assessment, treatment planning, and detecting potential abnormalities.
This response demonstrates a significant improvement in the model's ability to interpret and describe medical images, using appropriate terminology and providing a comprehensive analysis.
Saving the Fine-Tuned Model
Once we're satisfied with the performance of our fine-tuned model, we need to save it for future use. The Unsloth library provides convenient functions for saving the model and uploading it to Hugging Face Hub.
# Save the fine-tuned model locally
model.save_pretrained("./fine_tuned_model")
# Merge the adapter with the base model
merged_model = model.merge_and_unload()
# Save the merged model
merged_model.save_pretrained("./merged_model")
# Push the merged model to Hugging Face Hub
merged_model.push_to_hub("your-username/fine-tuned-llama-3-2-vision-medical")
This process creates two versions of the model:
- The fine-tuned model with a separate adapter
- A merged model that combines the base Llama 3.2 model with the fine-tuned adapter
Uploading the model to Hugging Face Hub makes it easily accessible for future use and sharing with the community.
Understanding LoRA and Model Merging
It's important to understand the concept of LoRA (Low-Rank Adaptation) and model merging in the context of fine-tuning large language models:
-
LoRA: This technique allows us to fine-tune only a small number of parameters, creating an "adapter" that modifies the behavior of the base model. This approach is more efficient and requires less computational resources than fine-tuning the entire model.
-
Model Merging: After fine-tuning, we have two components: the original Llama 3.2 Vision model and the LoRA adapter. Merging these components creates a single, unified model that incorporates the fine-tuned improvements without needing to load the adapter separately.
By using LoRA and model merging, we can achieve improved performance on specific tasks while maintaining the flexibility to use the original model for other purposes.
Potential Applications in Healthcare
The fine-tuned Llama 3.2 Vision model has numerous potential applications in healthcare, including:
-
Automated Screening: Quickly analyzing large volumes of medical images to flag potential abnormalities for further review by healthcare professionals.
-
Training and Education: Providing detailed descriptions of medical images to help train new radiologists and medical students.
-
Second Opinion: Offering an AI-powered second opinion to support radiologists in their diagnoses.
-
Research: Analyzing large datasets of medical images to identify patterns and trends that might not be apparent to human observers.
-
Telemedicine: Enhancing remote healthcare services by providing rapid, accurate interpretations of medical images shared digitally.
-
Emergency Triage: Assisting in the prioritization of cases in emergency departments by quickly assessing the severity of conditions based on medical images.
-
Rural Healthcare: Improving access to expert-level image analysis in areas with limited access to specialist radiologists.
-
Patient Communication: Generating clear, understandable descriptions of medical images to help explain conditions and treatments to patients.
Ethical Considerations and Limitations
While the fine-tuned Llama 3.2 Vision model offers exciting possibilities for medical image analysis, it's crucial to consider the ethical implications and limitations of using AI in healthcare:
-
Data Privacy: Ensure that all training data and patient information is handled in compliance with relevant privacy laws and regulations.
-
Bias and Fairness: Regularly assess the model for potential biases in its analysis, particularly across different demographic groups.
-
Transparency: Be clear about the use of AI in medical image analysis when communicating with patients and obtaining consent.
-
Human Oversight: The model should be used as a tool to assist healthcare professionals, not replace them. Final diagnoses and treatment decisions should always involve human judgment.
-
Continuous Evaluation: Regularly evaluate the model's performance against current medical standards and update it as necessary.
-
Limitations of Training Data: Recognize that the model's performance is limited by the scope and quality of its training data. It may not perform well on rare conditions or types of images it hasn't been exposed to.
-
Regulatory Compliance: Ensure that the use of AI in medical image analysis complies with relevant healthcare regulations and standards.
Future Directions
The field of AI in medical image analysis is rapidly evolving. Some potential future directions for research and development include:
-
Multimodal Integration: Combining image analysis with other data sources, such as patient history and lab results, for more comprehensive diagnoses.
-
Explainable AI: Developing methods to make the model's decision-making process more transparent and interpretable to healthcare professionals.
-
Continuous Learning: Implementing systems for the model to learn and improve from ongoing real-world use, while maintaining data privacy and security.
-
Specialization: Fine-tuning models for specific medical specialties or types of imaging (e.g., mammography, neuroimaging, cardiac imaging).
-
Edge Deployment: Optimizing the model for use on edge devices, allowing for rapid analysis in clinical settings without relying on cloud computing.
-
Collaborative AI: Developing systems that can work collaboratively with healthcare professionals, learning from their feedback and adjusting recommendations accordingly.
-
Predictive Analytics: Extending the model's capabilities to not only describe current images but also predict future outcomes or disease progression based on imaging data.
Conclusion
Fine-tuning the Llama 3.2 Vision model for medical image analysis represents a significant step forward in the application of AI to healthcare. By following the process outlined in this article, researchers and developers can create powerful tools to assist healthcare professionals in interpreting medical images more accurately and efficiently.
However, it's crucial to approach this technology with a balanced perspective, recognizing both its potential benefits and limitations. As we continue to refine and improve these models, we must prioritize ethical considerations, maintain human oversight, and work closely with healthcare professionals to ensure that AI remains a valuable tool in service of improved patient care.
The future of medical image analysis is bright, with AI poised to play an increasingly important role. By responsibly developing and deploying these technologies, we can look forward to a healthcare system that leverages the strengths of both human expertise and artificial intelligence to provide better outcomes for patients worldwide.
Article created from: https://www.youtube.com/watch?v=co0lDx5J23o