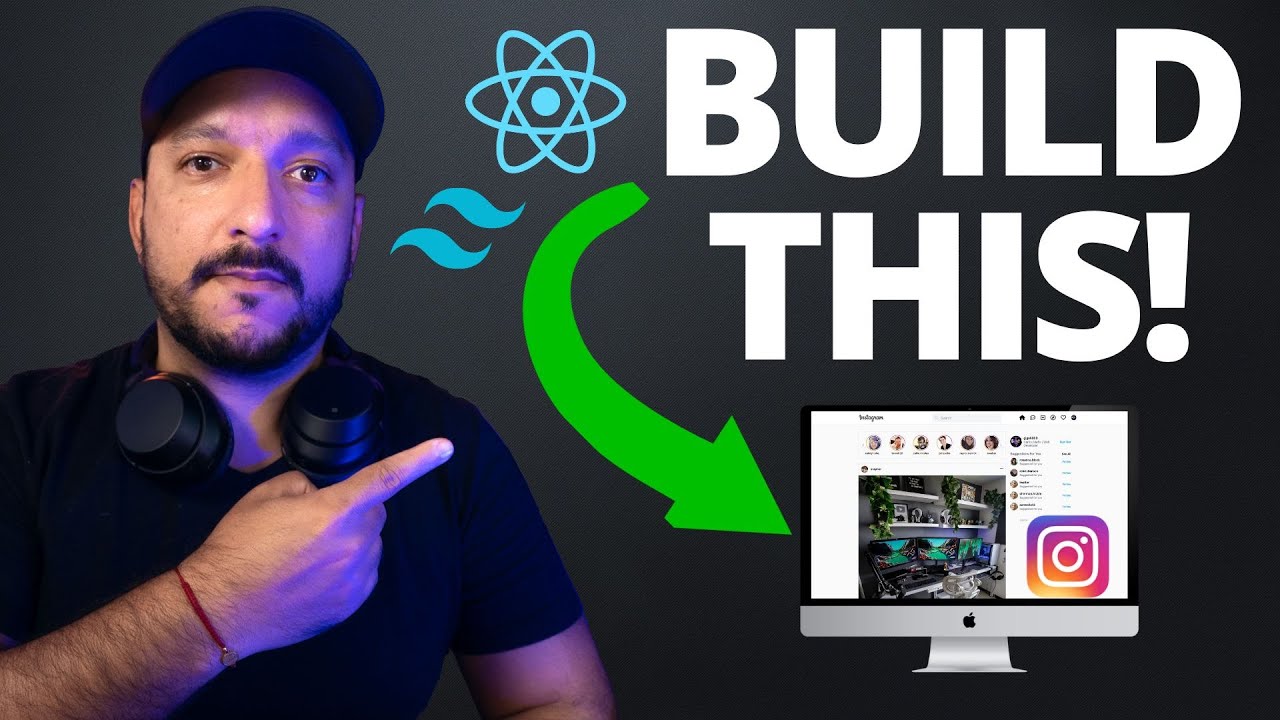
Create articles from any YouTube video or use our API to get YouTube transcriptions
Start for freeCreating an Instagram Clone with React and GraphQL
In the realm of web development, building complex applications like social media platforms can be both challenging and rewarding. In this guide, we delve into creating an Instagram clone using React for the frontend and GraphQL for the backend, covering key features such as creating posts, liking, commenting, and user authentication.
Setting Up the Project Environment
To kickstart our project, we need to set up our development environment. This involves installing necessary packages and dependencies for React and GraphQL. Ensure you have Node.js installed, then run the following commands to create a new React app and add GraphQL dependencies:
npx create-react-app instagram-clone
cd instagram-clone
npm install @apollo/client graphql react-router-dom
Implementing User Authentication
User authentication is crucial for a social media app. We start by creating a login feature using GraphQL mutations. First, write a GraphQL mutation for user login in a separate file (login.js
) within a graphql
directory:
import { gql } from '@apollo/client';
export const LOGIN_MUTATION = gql`
mutation Login($email: String!, $password: String!) {
login(email: $email, password: $password) {
token
}
}
`;
In your Login
component, use the useMutation
hook from Apollo Client to execute this mutation upon form submission, handling user inputs for email and password accordingly. Store the received token in local storage for session management.
Building the Post Creation Feature
Creating posts involves handling file uploads and text inputs. Utilize the react-dropzone
library for drag-and-drop file uploads and define another GraphQL mutation (addPost.js
) for post creation:
import { gql } from '@apollo/client';
export const ADD_POST_MUTATION = gql`
mutation AddPost($file: Upload!, $caption: String!) {
addPost(file: $file, caption: $caption) {
id
image
caption
}
}
`;
Incorporate this mutation in a NewPost
component, displaying a form for the caption and a drop zone for image uploads. On submission, execute the mutation with the selected file and caption.
Displaying Posts and Interaction
Fetch and display posts on the home page using a GraphQL query (getFeed.js
). Implement functionalities for liking and commenting on posts by defining corresponding mutations (likePost.js
, addComment.js
). Use the useQuery
and useMutation
hooks for fetching posts and interacting with them.
Final Touches: Navigation and Responsiveness
Implement navigation using react-router-dom
and ensure your application is responsive. Use Tailwind CSS or similar frameworks to streamline the styling process and achieve a polished look.
Conclusion
Building an Instagram clone with React and GraphQL offers a hands-on learning experience in developing full-stack applications. By following this guide, you'll gain insights into handling user authentication, file uploads, and real-time interactions, key components of modern web applications.
For detailed code examples and further explanations, refer to the comprehensive guide and source code provided. Embark on this project to enhance your skills in React, GraphQL, and full-stack development.